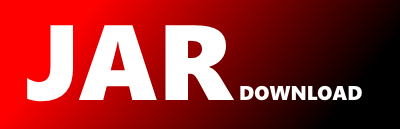
com.google.ortools.constraintsolver.RoutingSearchParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-or-tools Show documentation
Show all versions of google-or-tools Show documentation
A project to publish Google OR-Tools as a dependable dependency in Maven.
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ortools/constraint_solver/routing_parameters.proto
package com.google.ortools.constraintsolver;
/**
*
* Parameters defining the search used to solve vehicle routing problems.
*
*
* Protobuf type {@code operations_research.RoutingSearchParameters}
*/
public final class RoutingSearchParameters extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:operations_research.RoutingSearchParameters)
RoutingSearchParametersOrBuilder {
private static final long serialVersionUID = 0L;
// Use RoutingSearchParameters.newBuilder() to construct.
private RoutingSearchParameters(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RoutingSearchParameters() {
firstSolutionStrategy_ = 0;
useFilteredFirstSolutionStrategy_ = false;
savingsNeighborsRatio_ = 0D;
savingsAddReverseArcs_ = false;
localSearchMetaheuristic_ = 0;
guidedLocalSearchLambdaCoefficient_ = 0D;
useDepthFirstSearch_ = false;
optimizationStep_ = 0L;
solutionLimit_ = 0L;
timeLimitMs_ = 0L;
lnsTimeLimitMs_ = 0L;
useLightPropagation_ = false;
fingerprintArcCostEvaluators_ = false;
logSearch_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RoutingSearchParameters(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
firstSolutionStrategy_ = rawValue;
break;
}
case 16: {
useFilteredFirstSolutionStrategy_ = input.readBool();
break;
}
case 26: {
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder subBuilder = null;
if (localSearchOperators_ != null) {
subBuilder = localSearchOperators_.toBuilder();
}
localSearchOperators_ = input.readMessage(com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(localSearchOperators_);
localSearchOperators_ = subBuilder.buildPartial();
}
break;
}
case 32: {
int rawValue = input.readEnum();
localSearchMetaheuristic_ = rawValue;
break;
}
case 41: {
guidedLocalSearchLambdaCoefficient_ = input.readDouble();
break;
}
case 48: {
useDepthFirstSearch_ = input.readBool();
break;
}
case 56: {
optimizationStep_ = input.readInt64();
break;
}
case 64: {
solutionLimit_ = input.readInt64();
break;
}
case 72: {
timeLimitMs_ = input.readInt64();
break;
}
case 80: {
lnsTimeLimitMs_ = input.readInt64();
break;
}
case 88: {
useLightPropagation_ = input.readBool();
break;
}
case 96: {
fingerprintArcCostEvaluators_ = input.readBool();
break;
}
case 104: {
logSearch_ = input.readBool();
break;
}
case 113: {
savingsNeighborsRatio_ = input.readDouble();
break;
}
case 120: {
savingsAddReverseArcs_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.constraintsolver.RoutingSearchParameters.class, com.google.ortools.constraintsolver.RoutingSearchParameters.Builder.class);
}
public interface LocalSearchNeighborhoodOperatorsOrBuilder extends
// @@protoc_insertion_point(interface_extends:operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators)
com.google.protobuf.MessageOrBuilder {
/**
*
* --- Inter-route operators ---
* Operator which moves a single node to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> 3 -> [2] -> 4 -> 5
* 1 -> 3 -> 4 -> [2] -> 5
* 1 -> 2 -> 4 -> [3] -> 5
* 1 -> [4] -> 2 -> 3 -> 5
*
*
* bool use_relocate = 1;
*/
boolean getUseRelocate();
/**
*
* Operator which moves a pair of pickup and delivery nodes to another
* position where the first node of the pair must be before the second node
* on the same path.
* Possible neighbors for the path 1 -> A -> B -> 2 -> 3 (where (1, 3) are
* first and last nodes of the path and can therefore not be moved, and
* (A, B) is a pair of nodes):
* 1 -> [A] -> 2 -> [B] -> 3
* 1 -> 2 -> [A] -> [B] -> 3
*
*
* bool use_relocate_pair = 2;
*/
boolean getUseRelocatePair();
/**
*
* Relocate neighborhood which moves chains of neighbors.
* The operator starts by relocating a node n after a node m, then continues
* moving nodes which were after n as long as the "cost" added is less than
* the "cost" of the arc (m, n). If the new chain doesn't respect the domain
* of next variables, it will try reordering the nodes until it finds a
* valid path.
* Possible neighbors for path 1 -> A -> B -> C -> D -> E -> 2 (where (1, 2)
* are first and last nodes of the path and can therefore not be moved, A
* must be performed before B, and A, D and E are located at the same
* place):
* 1 -> A -> C -> [B] -> D -> E -> 2
* 1 -> A -> C -> D -> [B] -> E -> 2
* 1 -> A -> C -> D -> E -> [B] -> 2
* 1 -> A -> B -> D -> [C] -> E -> 2
* 1 -> A -> B -> D -> E -> [C] -> 2
* 1 -> A -> [D] -> [E] -> B -> C -> 2
* 1 -> A -> B -> [D] -> [E] -> C -> 2
* 1 -> A -> [E] -> B -> C -> D -> 2
* 1 -> A -> B -> [E] -> C -> D -> 2
* 1 -> A -> B -> C -> [E] -> D -> 2
* This operator is extremelly useful to move chains of nodes which are
* located at the same place (for instance nodes part of a same stop).
*
*
* bool use_relocate_neighbors = 3;
*/
boolean getUseRelocateNeighbors();
/**
*
* Operator which exchanges the positions of two nodes.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3] -> [2] -> 4 -> 5
* 1 -> [4] -> 3 -> [2] -> 5
* 1 -> 2 -> [4] -> [3] -> 5
*
*
* bool use_exchange = 4;
*/
boolean getUseExchange();
/**
*
* Operator which cross exchanges the starting chains of 2 paths, including
* exchanging the whole paths.
* First and last nodes are not moved.
* Possible neighbors for the paths 1 -> 2 -> 3 -> 4 -> 5 and 6 -> 7 -> 8
* (where (1, 5) and (6, 8) are first and last nodes of the paths and can
* therefore not be moved):
* 1 -> [7] -> 3 -> 4 -> 5 6 -> [2] -> 8
* 1 -> [7] -> 4 -> 5 6 -> [2 -> 3] -> 8
* 1 -> [7] -> 5 6 -> [2 -> 3 -> 4] -> 8
*
*
* bool use_cross = 5;
*/
boolean getUseCross();
/**
*
* Not implemented as of 10/2015.
*
*
* bool use_cross_exchange = 6;
*/
boolean getUseCrossExchange();
/**
*
* --- Intra-route operators ---
* Operator which reverves a sub-chain of a path. It is called TwoOpt
* because it breaks two arcs on the path; resulting paths are called
* two-optimal.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3 -> 2] -> 4 -> 5
* 1 -> [4 -> 3 -> 2] -> 5
* 1 -> 2 -> [4 -> 3] -> 5
*
*
* bool use_two_opt = 7;
*/
boolean getUseTwoOpt();
/**
*
* Operator which moves sub-chains of a path of length 1, 2 and 3 to another
* position in the same path.
* When the length of the sub-chain is 1, the operator simply moves a node
* to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5, for a sub-chain
* length of 2 (where (1, 5) are first and last nodes of the path and can
* therefore not be moved):
* 1 -> 4 -> [2 -> 3] -> 5
* 1 -> [3 -> 4] -> 2 -> 5
* The OR_OPT operator is a limited version of 3-Opt (breaks 3 arcs on a
* path).
*
*
* bool use_or_opt = 8;
*/
boolean getUseOrOpt();
/**
*
* Lin-Kernighan operator.
* While the accumulated local gain is positive, performs a 2-OPT or a 3-OPT
* move followed by a series of 2-OPT moves. Returns a neighbor for which
* the global gain is positive.
*
*
* bool use_lin_kernighan = 9;
*/
boolean getUseLinKernighan();
/**
*
* Sliding TSP operator.
* Uses an exact dynamic programming algorithm to solve the TSP
* corresponding to path sub-chains.
* For a subchain 1 -> 2 -> 3 -> 4 -> 5 -> 6, solves the TSP on
* nodes A, 2, 3, 4, 5, where A is a merger of nodes 1 and 6 such that
* cost(A,i) = cost(1,i) and cost(i,A) = cost(i,6).
*
*
* bool use_tsp_opt = 10;
*/
boolean getUseTspOpt();
/**
*
* --- Operators on inactive nodes ---
* Operator which inserts an inactive node into a path.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 2 -> 3 -> 4
* 1 -> 2 -> [5] -> 3 -> 4
* 1 -> 2 -> 3 -> [5] -> 4
*
*
* bool use_make_active = 11;
*/
boolean getUseMakeActive();
/**
*
* Operator which relocates a node while making an inactive one active.
* As of 3/2017, the operator is limited to two kinds of moves:
* - Relocating a node and replacing it by an inactive node.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 3 -> 5, 2 -> 4 -> 6.
* - Relocating a node and inserting an inactive node next to it.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 4 -> 3 -> 5, 2 -> 6.
*
*
* bool use_relocate_and_make_active = 21;
*/
boolean getUseRelocateAndMakeActive();
/**
*
* Operator which makes path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
*
*
* bool use_make_inactive = 12;
*/
boolean getUseMakeInactive();
/**
*
* Operator which makes a "chain" of path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
* 1 -> 4 with 2 and 3 inactive
*
*
* bool use_make_chain_inactive = 13;
*/
boolean getUseMakeChainInactive();
/**
*
* Operator which replaces an active node by an inactive one.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* bool use_swap_active = 14;
*/
boolean getUseSwapActive();
/**
*
* Operator which makes an inactive node active and an active one inactive.
* It is similar to SwapActiveOperator excepts that it tries to insert the
* inactive node in all possible positions instead of just the position of
* the node made inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 3 -> [5] -> 4 with 2 inactive
* 1 -> [5] -> 2 -> 4 with 3 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* bool use_extended_swap_active = 15;
*/
boolean getUseExtendedSwapActive();
/**
*
* Operator which makes an inactive node active and an active pair of nodes
* inactive OR makes an inactive pair of nodes active and an active node
* inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path and (2,3) is a pair
* of nodes) are:
* 1 -> [5] -> 4 with (2,3) inactive
* Possible neighbors for the path 1 -> 2 -> 3 with (4,5) inactive
* (where 1 and 3 are first and last nodes of the path and (4,5) is a pair
* of nodes) are:
* 1 -> [4] -> [5] -> 3 with 2 inactive
*
*
* bool use_node_pair_swap_active = 20;
*/
boolean getUseNodePairSwapActive();
/**
*
* --- Large neighborhood search operators ---
* Operator which relaxes two sub-chains of three consecutive arcs each.
* Each sub-chain is defined by a start node and the next three arcs. Those
* six arcs are relaxed to build a new neighbor.
* PATH_LNS explores all possible pairs of starting nodes and so defines
* n^2 neighbors, n being the number of nodes.
* Note that the two sub-chains can be part of the same path; they even may
* overlap.
*
*
* bool use_path_lns = 16;
*/
boolean getUsePathLns();
/**
*
* Operator which relaxes one entire path and all unactive nodes.
*
*
* bool use_full_path_lns = 17;
*/
boolean getUseFullPathLns();
/**
*
* TSP-base LNS.
* Randomly merges consecutive nodes until n "meta"-nodes remain and solves
* the corresponding TSP.
* This defines an "unlimited" neighborhood which must be stopped by search
* limits. To force diversification, the operator iteratively forces each
* node to serve as base of a meta-node.
*
*
* bool use_tsp_lns = 18;
*/
boolean getUseTspLns();
/**
*
* Operator which relaxes all inactive nodes and one sub-chain of six
* consecutive arcs. That way the path can be improved by inserting inactive
* nodes or swaping arcs.
*
*
* bool use_inactive_lns = 19;
*/
boolean getUseInactiveLns();
}
/**
*
* Local search neighborhood operators used to build a solutions neighborhood.
*
*
* Protobuf type {@code operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators}
*/
public static final class LocalSearchNeighborhoodOperators extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators)
LocalSearchNeighborhoodOperatorsOrBuilder {
private static final long serialVersionUID = 0L;
// Use LocalSearchNeighborhoodOperators.newBuilder() to construct.
private LocalSearchNeighborhoodOperators(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LocalSearchNeighborhoodOperators() {
useRelocate_ = false;
useRelocatePair_ = false;
useRelocateNeighbors_ = false;
useExchange_ = false;
useCross_ = false;
useCrossExchange_ = false;
useTwoOpt_ = false;
useOrOpt_ = false;
useLinKernighan_ = false;
useTspOpt_ = false;
useMakeActive_ = false;
useRelocateAndMakeActive_ = false;
useMakeInactive_ = false;
useMakeChainInactive_ = false;
useSwapActive_ = false;
useExtendedSwapActive_ = false;
useNodePairSwapActive_ = false;
usePathLns_ = false;
useFullPathLns_ = false;
useTspLns_ = false;
useInactiveLns_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LocalSearchNeighborhoodOperators(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
useRelocate_ = input.readBool();
break;
}
case 16: {
useRelocatePair_ = input.readBool();
break;
}
case 24: {
useRelocateNeighbors_ = input.readBool();
break;
}
case 32: {
useExchange_ = input.readBool();
break;
}
case 40: {
useCross_ = input.readBool();
break;
}
case 48: {
useCrossExchange_ = input.readBool();
break;
}
case 56: {
useTwoOpt_ = input.readBool();
break;
}
case 64: {
useOrOpt_ = input.readBool();
break;
}
case 72: {
useLinKernighan_ = input.readBool();
break;
}
case 80: {
useTspOpt_ = input.readBool();
break;
}
case 88: {
useMakeActive_ = input.readBool();
break;
}
case 96: {
useMakeInactive_ = input.readBool();
break;
}
case 104: {
useMakeChainInactive_ = input.readBool();
break;
}
case 112: {
useSwapActive_ = input.readBool();
break;
}
case 120: {
useExtendedSwapActive_ = input.readBool();
break;
}
case 128: {
usePathLns_ = input.readBool();
break;
}
case 136: {
useFullPathLns_ = input.readBool();
break;
}
case 144: {
useTspLns_ = input.readBool();
break;
}
case 152: {
useInactiveLns_ = input.readBool();
break;
}
case 160: {
useNodePairSwapActive_ = input.readBool();
break;
}
case 168: {
useRelocateAndMakeActive_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_LocalSearchNeighborhoodOperators_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_LocalSearchNeighborhoodOperators_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.class, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder.class);
}
public static final int USE_RELOCATE_FIELD_NUMBER = 1;
private boolean useRelocate_;
/**
*
* --- Inter-route operators ---
* Operator which moves a single node to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> 3 -> [2] -> 4 -> 5
* 1 -> 3 -> 4 -> [2] -> 5
* 1 -> 2 -> 4 -> [3] -> 5
* 1 -> [4] -> 2 -> 3 -> 5
*
*
* bool use_relocate = 1;
*/
public boolean getUseRelocate() {
return useRelocate_;
}
public static final int USE_RELOCATE_PAIR_FIELD_NUMBER = 2;
private boolean useRelocatePair_;
/**
*
* Operator which moves a pair of pickup and delivery nodes to another
* position where the first node of the pair must be before the second node
* on the same path.
* Possible neighbors for the path 1 -> A -> B -> 2 -> 3 (where (1, 3) are
* first and last nodes of the path and can therefore not be moved, and
* (A, B) is a pair of nodes):
* 1 -> [A] -> 2 -> [B] -> 3
* 1 -> 2 -> [A] -> [B] -> 3
*
*
* bool use_relocate_pair = 2;
*/
public boolean getUseRelocatePair() {
return useRelocatePair_;
}
public static final int USE_RELOCATE_NEIGHBORS_FIELD_NUMBER = 3;
private boolean useRelocateNeighbors_;
/**
*
* Relocate neighborhood which moves chains of neighbors.
* The operator starts by relocating a node n after a node m, then continues
* moving nodes which were after n as long as the "cost" added is less than
* the "cost" of the arc (m, n). If the new chain doesn't respect the domain
* of next variables, it will try reordering the nodes until it finds a
* valid path.
* Possible neighbors for path 1 -> A -> B -> C -> D -> E -> 2 (where (1, 2)
* are first and last nodes of the path and can therefore not be moved, A
* must be performed before B, and A, D and E are located at the same
* place):
* 1 -> A -> C -> [B] -> D -> E -> 2
* 1 -> A -> C -> D -> [B] -> E -> 2
* 1 -> A -> C -> D -> E -> [B] -> 2
* 1 -> A -> B -> D -> [C] -> E -> 2
* 1 -> A -> B -> D -> E -> [C] -> 2
* 1 -> A -> [D] -> [E] -> B -> C -> 2
* 1 -> A -> B -> [D] -> [E] -> C -> 2
* 1 -> A -> [E] -> B -> C -> D -> 2
* 1 -> A -> B -> [E] -> C -> D -> 2
* 1 -> A -> B -> C -> [E] -> D -> 2
* This operator is extremelly useful to move chains of nodes which are
* located at the same place (for instance nodes part of a same stop).
*
*
* bool use_relocate_neighbors = 3;
*/
public boolean getUseRelocateNeighbors() {
return useRelocateNeighbors_;
}
public static final int USE_EXCHANGE_FIELD_NUMBER = 4;
private boolean useExchange_;
/**
*
* Operator which exchanges the positions of two nodes.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3] -> [2] -> 4 -> 5
* 1 -> [4] -> 3 -> [2] -> 5
* 1 -> 2 -> [4] -> [3] -> 5
*
*
* bool use_exchange = 4;
*/
public boolean getUseExchange() {
return useExchange_;
}
public static final int USE_CROSS_FIELD_NUMBER = 5;
private boolean useCross_;
/**
*
* Operator which cross exchanges the starting chains of 2 paths, including
* exchanging the whole paths.
* First and last nodes are not moved.
* Possible neighbors for the paths 1 -> 2 -> 3 -> 4 -> 5 and 6 -> 7 -> 8
* (where (1, 5) and (6, 8) are first and last nodes of the paths and can
* therefore not be moved):
* 1 -> [7] -> 3 -> 4 -> 5 6 -> [2] -> 8
* 1 -> [7] -> 4 -> 5 6 -> [2 -> 3] -> 8
* 1 -> [7] -> 5 6 -> [2 -> 3 -> 4] -> 8
*
*
* bool use_cross = 5;
*/
public boolean getUseCross() {
return useCross_;
}
public static final int USE_CROSS_EXCHANGE_FIELD_NUMBER = 6;
private boolean useCrossExchange_;
/**
*
* Not implemented as of 10/2015.
*
*
* bool use_cross_exchange = 6;
*/
public boolean getUseCrossExchange() {
return useCrossExchange_;
}
public static final int USE_TWO_OPT_FIELD_NUMBER = 7;
private boolean useTwoOpt_;
/**
*
* --- Intra-route operators ---
* Operator which reverves a sub-chain of a path. It is called TwoOpt
* because it breaks two arcs on the path; resulting paths are called
* two-optimal.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3 -> 2] -> 4 -> 5
* 1 -> [4 -> 3 -> 2] -> 5
* 1 -> 2 -> [4 -> 3] -> 5
*
*
* bool use_two_opt = 7;
*/
public boolean getUseTwoOpt() {
return useTwoOpt_;
}
public static final int USE_OR_OPT_FIELD_NUMBER = 8;
private boolean useOrOpt_;
/**
*
* Operator which moves sub-chains of a path of length 1, 2 and 3 to another
* position in the same path.
* When the length of the sub-chain is 1, the operator simply moves a node
* to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5, for a sub-chain
* length of 2 (where (1, 5) are first and last nodes of the path and can
* therefore not be moved):
* 1 -> 4 -> [2 -> 3] -> 5
* 1 -> [3 -> 4] -> 2 -> 5
* The OR_OPT operator is a limited version of 3-Opt (breaks 3 arcs on a
* path).
*
*
* bool use_or_opt = 8;
*/
public boolean getUseOrOpt() {
return useOrOpt_;
}
public static final int USE_LIN_KERNIGHAN_FIELD_NUMBER = 9;
private boolean useLinKernighan_;
/**
*
* Lin-Kernighan operator.
* While the accumulated local gain is positive, performs a 2-OPT or a 3-OPT
* move followed by a series of 2-OPT moves. Returns a neighbor for which
* the global gain is positive.
*
*
* bool use_lin_kernighan = 9;
*/
public boolean getUseLinKernighan() {
return useLinKernighan_;
}
public static final int USE_TSP_OPT_FIELD_NUMBER = 10;
private boolean useTspOpt_;
/**
*
* Sliding TSP operator.
* Uses an exact dynamic programming algorithm to solve the TSP
* corresponding to path sub-chains.
* For a subchain 1 -> 2 -> 3 -> 4 -> 5 -> 6, solves the TSP on
* nodes A, 2, 3, 4, 5, where A is a merger of nodes 1 and 6 such that
* cost(A,i) = cost(1,i) and cost(i,A) = cost(i,6).
*
*
* bool use_tsp_opt = 10;
*/
public boolean getUseTspOpt() {
return useTspOpt_;
}
public static final int USE_MAKE_ACTIVE_FIELD_NUMBER = 11;
private boolean useMakeActive_;
/**
*
* --- Operators on inactive nodes ---
* Operator which inserts an inactive node into a path.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 2 -> 3 -> 4
* 1 -> 2 -> [5] -> 3 -> 4
* 1 -> 2 -> 3 -> [5] -> 4
*
*
* bool use_make_active = 11;
*/
public boolean getUseMakeActive() {
return useMakeActive_;
}
public static final int USE_RELOCATE_AND_MAKE_ACTIVE_FIELD_NUMBER = 21;
private boolean useRelocateAndMakeActive_;
/**
*
* Operator which relocates a node while making an inactive one active.
* As of 3/2017, the operator is limited to two kinds of moves:
* - Relocating a node and replacing it by an inactive node.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 3 -> 5, 2 -> 4 -> 6.
* - Relocating a node and inserting an inactive node next to it.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 4 -> 3 -> 5, 2 -> 6.
*
*
* bool use_relocate_and_make_active = 21;
*/
public boolean getUseRelocateAndMakeActive() {
return useRelocateAndMakeActive_;
}
public static final int USE_MAKE_INACTIVE_FIELD_NUMBER = 12;
private boolean useMakeInactive_;
/**
*
* Operator which makes path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
*
*
* bool use_make_inactive = 12;
*/
public boolean getUseMakeInactive() {
return useMakeInactive_;
}
public static final int USE_MAKE_CHAIN_INACTIVE_FIELD_NUMBER = 13;
private boolean useMakeChainInactive_;
/**
*
* Operator which makes a "chain" of path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
* 1 -> 4 with 2 and 3 inactive
*
*
* bool use_make_chain_inactive = 13;
*/
public boolean getUseMakeChainInactive() {
return useMakeChainInactive_;
}
public static final int USE_SWAP_ACTIVE_FIELD_NUMBER = 14;
private boolean useSwapActive_;
/**
*
* Operator which replaces an active node by an inactive one.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* bool use_swap_active = 14;
*/
public boolean getUseSwapActive() {
return useSwapActive_;
}
public static final int USE_EXTENDED_SWAP_ACTIVE_FIELD_NUMBER = 15;
private boolean useExtendedSwapActive_;
/**
*
* Operator which makes an inactive node active and an active one inactive.
* It is similar to SwapActiveOperator excepts that it tries to insert the
* inactive node in all possible positions instead of just the position of
* the node made inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 3 -> [5] -> 4 with 2 inactive
* 1 -> [5] -> 2 -> 4 with 3 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* bool use_extended_swap_active = 15;
*/
public boolean getUseExtendedSwapActive() {
return useExtendedSwapActive_;
}
public static final int USE_NODE_PAIR_SWAP_ACTIVE_FIELD_NUMBER = 20;
private boolean useNodePairSwapActive_;
/**
*
* Operator which makes an inactive node active and an active pair of nodes
* inactive OR makes an inactive pair of nodes active and an active node
* inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path and (2,3) is a pair
* of nodes) are:
* 1 -> [5] -> 4 with (2,3) inactive
* Possible neighbors for the path 1 -> 2 -> 3 with (4,5) inactive
* (where 1 and 3 are first and last nodes of the path and (4,5) is a pair
* of nodes) are:
* 1 -> [4] -> [5] -> 3 with 2 inactive
*
*
* bool use_node_pair_swap_active = 20;
*/
public boolean getUseNodePairSwapActive() {
return useNodePairSwapActive_;
}
public static final int USE_PATH_LNS_FIELD_NUMBER = 16;
private boolean usePathLns_;
/**
*
* --- Large neighborhood search operators ---
* Operator which relaxes two sub-chains of three consecutive arcs each.
* Each sub-chain is defined by a start node and the next three arcs. Those
* six arcs are relaxed to build a new neighbor.
* PATH_LNS explores all possible pairs of starting nodes and so defines
* n^2 neighbors, n being the number of nodes.
* Note that the two sub-chains can be part of the same path; they even may
* overlap.
*
*
* bool use_path_lns = 16;
*/
public boolean getUsePathLns() {
return usePathLns_;
}
public static final int USE_FULL_PATH_LNS_FIELD_NUMBER = 17;
private boolean useFullPathLns_;
/**
*
* Operator which relaxes one entire path and all unactive nodes.
*
*
* bool use_full_path_lns = 17;
*/
public boolean getUseFullPathLns() {
return useFullPathLns_;
}
public static final int USE_TSP_LNS_FIELD_NUMBER = 18;
private boolean useTspLns_;
/**
*
* TSP-base LNS.
* Randomly merges consecutive nodes until n "meta"-nodes remain and solves
* the corresponding TSP.
* This defines an "unlimited" neighborhood which must be stopped by search
* limits. To force diversification, the operator iteratively forces each
* node to serve as base of a meta-node.
*
*
* bool use_tsp_lns = 18;
*/
public boolean getUseTspLns() {
return useTspLns_;
}
public static final int USE_INACTIVE_LNS_FIELD_NUMBER = 19;
private boolean useInactiveLns_;
/**
*
* Operator which relaxes all inactive nodes and one sub-chain of six
* consecutive arcs. That way the path can be improved by inserting inactive
* nodes or swaping arcs.
*
*
* bool use_inactive_lns = 19;
*/
public boolean getUseInactiveLns() {
return useInactiveLns_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (useRelocate_ != false) {
output.writeBool(1, useRelocate_);
}
if (useRelocatePair_ != false) {
output.writeBool(2, useRelocatePair_);
}
if (useRelocateNeighbors_ != false) {
output.writeBool(3, useRelocateNeighbors_);
}
if (useExchange_ != false) {
output.writeBool(4, useExchange_);
}
if (useCross_ != false) {
output.writeBool(5, useCross_);
}
if (useCrossExchange_ != false) {
output.writeBool(6, useCrossExchange_);
}
if (useTwoOpt_ != false) {
output.writeBool(7, useTwoOpt_);
}
if (useOrOpt_ != false) {
output.writeBool(8, useOrOpt_);
}
if (useLinKernighan_ != false) {
output.writeBool(9, useLinKernighan_);
}
if (useTspOpt_ != false) {
output.writeBool(10, useTspOpt_);
}
if (useMakeActive_ != false) {
output.writeBool(11, useMakeActive_);
}
if (useMakeInactive_ != false) {
output.writeBool(12, useMakeInactive_);
}
if (useMakeChainInactive_ != false) {
output.writeBool(13, useMakeChainInactive_);
}
if (useSwapActive_ != false) {
output.writeBool(14, useSwapActive_);
}
if (useExtendedSwapActive_ != false) {
output.writeBool(15, useExtendedSwapActive_);
}
if (usePathLns_ != false) {
output.writeBool(16, usePathLns_);
}
if (useFullPathLns_ != false) {
output.writeBool(17, useFullPathLns_);
}
if (useTspLns_ != false) {
output.writeBool(18, useTspLns_);
}
if (useInactiveLns_ != false) {
output.writeBool(19, useInactiveLns_);
}
if (useNodePairSwapActive_ != false) {
output.writeBool(20, useNodePairSwapActive_);
}
if (useRelocateAndMakeActive_ != false) {
output.writeBool(21, useRelocateAndMakeActive_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (useRelocate_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, useRelocate_);
}
if (useRelocatePair_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, useRelocatePair_);
}
if (useRelocateNeighbors_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, useRelocateNeighbors_);
}
if (useExchange_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, useExchange_);
}
if (useCross_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, useCross_);
}
if (useCrossExchange_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(6, useCrossExchange_);
}
if (useTwoOpt_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(7, useTwoOpt_);
}
if (useOrOpt_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(8, useOrOpt_);
}
if (useLinKernighan_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, useLinKernighan_);
}
if (useTspOpt_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(10, useTspOpt_);
}
if (useMakeActive_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(11, useMakeActive_);
}
if (useMakeInactive_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(12, useMakeInactive_);
}
if (useMakeChainInactive_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, useMakeChainInactive_);
}
if (useSwapActive_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(14, useSwapActive_);
}
if (useExtendedSwapActive_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(15, useExtendedSwapActive_);
}
if (usePathLns_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(16, usePathLns_);
}
if (useFullPathLns_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(17, useFullPathLns_);
}
if (useTspLns_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(18, useTspLns_);
}
if (useInactiveLns_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(19, useInactiveLns_);
}
if (useNodePairSwapActive_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(20, useNodePairSwapActive_);
}
if (useRelocateAndMakeActive_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(21, useRelocateAndMakeActive_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators)) {
return super.equals(obj);
}
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators other = (com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators) obj;
boolean result = true;
result = result && (getUseRelocate()
== other.getUseRelocate());
result = result && (getUseRelocatePair()
== other.getUseRelocatePair());
result = result && (getUseRelocateNeighbors()
== other.getUseRelocateNeighbors());
result = result && (getUseExchange()
== other.getUseExchange());
result = result && (getUseCross()
== other.getUseCross());
result = result && (getUseCrossExchange()
== other.getUseCrossExchange());
result = result && (getUseTwoOpt()
== other.getUseTwoOpt());
result = result && (getUseOrOpt()
== other.getUseOrOpt());
result = result && (getUseLinKernighan()
== other.getUseLinKernighan());
result = result && (getUseTspOpt()
== other.getUseTspOpt());
result = result && (getUseMakeActive()
== other.getUseMakeActive());
result = result && (getUseRelocateAndMakeActive()
== other.getUseRelocateAndMakeActive());
result = result && (getUseMakeInactive()
== other.getUseMakeInactive());
result = result && (getUseMakeChainInactive()
== other.getUseMakeChainInactive());
result = result && (getUseSwapActive()
== other.getUseSwapActive());
result = result && (getUseExtendedSwapActive()
== other.getUseExtendedSwapActive());
result = result && (getUseNodePairSwapActive()
== other.getUseNodePairSwapActive());
result = result && (getUsePathLns()
== other.getUsePathLns());
result = result && (getUseFullPathLns()
== other.getUseFullPathLns());
result = result && (getUseTspLns()
== other.getUseTspLns());
result = result && (getUseInactiveLns()
== other.getUseInactiveLns());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + USE_RELOCATE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseRelocate());
hash = (37 * hash) + USE_RELOCATE_PAIR_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseRelocatePair());
hash = (37 * hash) + USE_RELOCATE_NEIGHBORS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseRelocateNeighbors());
hash = (37 * hash) + USE_EXCHANGE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseExchange());
hash = (37 * hash) + USE_CROSS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseCross());
hash = (37 * hash) + USE_CROSS_EXCHANGE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseCrossExchange());
hash = (37 * hash) + USE_TWO_OPT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseTwoOpt());
hash = (37 * hash) + USE_OR_OPT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseOrOpt());
hash = (37 * hash) + USE_LIN_KERNIGHAN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseLinKernighan());
hash = (37 * hash) + USE_TSP_OPT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseTspOpt());
hash = (37 * hash) + USE_MAKE_ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseMakeActive());
hash = (37 * hash) + USE_RELOCATE_AND_MAKE_ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseRelocateAndMakeActive());
hash = (37 * hash) + USE_MAKE_INACTIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseMakeInactive());
hash = (37 * hash) + USE_MAKE_CHAIN_INACTIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseMakeChainInactive());
hash = (37 * hash) + USE_SWAP_ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseSwapActive());
hash = (37 * hash) + USE_EXTENDED_SWAP_ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseExtendedSwapActive());
hash = (37 * hash) + USE_NODE_PAIR_SWAP_ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseNodePairSwapActive());
hash = (37 * hash) + USE_PATH_LNS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUsePathLns());
hash = (37 * hash) + USE_FULL_PATH_LNS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseFullPathLns());
hash = (37 * hash) + USE_TSP_LNS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseTspLns());
hash = (37 * hash) + USE_INACTIVE_LNS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseInactiveLns());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Local search neighborhood operators used to build a solutions neighborhood.
*
*
* Protobuf type {@code operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators)
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperatorsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_LocalSearchNeighborhoodOperators_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_LocalSearchNeighborhoodOperators_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.class, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder.class);
}
// Construct using com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
useRelocate_ = false;
useRelocatePair_ = false;
useRelocateNeighbors_ = false;
useExchange_ = false;
useCross_ = false;
useCrossExchange_ = false;
useTwoOpt_ = false;
useOrOpt_ = false;
useLinKernighan_ = false;
useTspOpt_ = false;
useMakeActive_ = false;
useRelocateAndMakeActive_ = false;
useMakeInactive_ = false;
useMakeChainInactive_ = false;
useSwapActive_ = false;
useExtendedSwapActive_ = false;
useNodePairSwapActive_ = false;
usePathLns_ = false;
useFullPathLns_ = false;
useTspLns_ = false;
useInactiveLns_ = false;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_LocalSearchNeighborhoodOperators_descriptor;
}
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators getDefaultInstanceForType() {
return com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.getDefaultInstance();
}
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators build() {
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators buildPartial() {
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators result = new com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators(this);
result.useRelocate_ = useRelocate_;
result.useRelocatePair_ = useRelocatePair_;
result.useRelocateNeighbors_ = useRelocateNeighbors_;
result.useExchange_ = useExchange_;
result.useCross_ = useCross_;
result.useCrossExchange_ = useCrossExchange_;
result.useTwoOpt_ = useTwoOpt_;
result.useOrOpt_ = useOrOpt_;
result.useLinKernighan_ = useLinKernighan_;
result.useTspOpt_ = useTspOpt_;
result.useMakeActive_ = useMakeActive_;
result.useRelocateAndMakeActive_ = useRelocateAndMakeActive_;
result.useMakeInactive_ = useMakeInactive_;
result.useMakeChainInactive_ = useMakeChainInactive_;
result.useSwapActive_ = useSwapActive_;
result.useExtendedSwapActive_ = useExtendedSwapActive_;
result.useNodePairSwapActive_ = useNodePairSwapActive_;
result.usePathLns_ = usePathLns_;
result.useFullPathLns_ = useFullPathLns_;
result.useTspLns_ = useTspLns_;
result.useInactiveLns_ = useInactiveLns_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators) {
return mergeFrom((com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators other) {
if (other == com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.getDefaultInstance()) return this;
if (other.getUseRelocate() != false) {
setUseRelocate(other.getUseRelocate());
}
if (other.getUseRelocatePair() != false) {
setUseRelocatePair(other.getUseRelocatePair());
}
if (other.getUseRelocateNeighbors() != false) {
setUseRelocateNeighbors(other.getUseRelocateNeighbors());
}
if (other.getUseExchange() != false) {
setUseExchange(other.getUseExchange());
}
if (other.getUseCross() != false) {
setUseCross(other.getUseCross());
}
if (other.getUseCrossExchange() != false) {
setUseCrossExchange(other.getUseCrossExchange());
}
if (other.getUseTwoOpt() != false) {
setUseTwoOpt(other.getUseTwoOpt());
}
if (other.getUseOrOpt() != false) {
setUseOrOpt(other.getUseOrOpt());
}
if (other.getUseLinKernighan() != false) {
setUseLinKernighan(other.getUseLinKernighan());
}
if (other.getUseTspOpt() != false) {
setUseTspOpt(other.getUseTspOpt());
}
if (other.getUseMakeActive() != false) {
setUseMakeActive(other.getUseMakeActive());
}
if (other.getUseRelocateAndMakeActive() != false) {
setUseRelocateAndMakeActive(other.getUseRelocateAndMakeActive());
}
if (other.getUseMakeInactive() != false) {
setUseMakeInactive(other.getUseMakeInactive());
}
if (other.getUseMakeChainInactive() != false) {
setUseMakeChainInactive(other.getUseMakeChainInactive());
}
if (other.getUseSwapActive() != false) {
setUseSwapActive(other.getUseSwapActive());
}
if (other.getUseExtendedSwapActive() != false) {
setUseExtendedSwapActive(other.getUseExtendedSwapActive());
}
if (other.getUseNodePairSwapActive() != false) {
setUseNodePairSwapActive(other.getUseNodePairSwapActive());
}
if (other.getUsePathLns() != false) {
setUsePathLns(other.getUsePathLns());
}
if (other.getUseFullPathLns() != false) {
setUseFullPathLns(other.getUseFullPathLns());
}
if (other.getUseTspLns() != false) {
setUseTspLns(other.getUseTspLns());
}
if (other.getUseInactiveLns() != false) {
setUseInactiveLns(other.getUseInactiveLns());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private boolean useRelocate_ ;
/**
*
* --- Inter-route operators ---
* Operator which moves a single node to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> 3 -> [2] -> 4 -> 5
* 1 -> 3 -> 4 -> [2] -> 5
* 1 -> 2 -> 4 -> [3] -> 5
* 1 -> [4] -> 2 -> 3 -> 5
*
*
* bool use_relocate = 1;
*/
public boolean getUseRelocate() {
return useRelocate_;
}
/**
*
* --- Inter-route operators ---
* Operator which moves a single node to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> 3 -> [2] -> 4 -> 5
* 1 -> 3 -> 4 -> [2] -> 5
* 1 -> 2 -> 4 -> [3] -> 5
* 1 -> [4] -> 2 -> 3 -> 5
*
*
* bool use_relocate = 1;
*/
public Builder setUseRelocate(boolean value) {
useRelocate_ = value;
onChanged();
return this;
}
/**
*
* --- Inter-route operators ---
* Operator which moves a single node to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> 3 -> [2] -> 4 -> 5
* 1 -> 3 -> 4 -> [2] -> 5
* 1 -> 2 -> 4 -> [3] -> 5
* 1 -> [4] -> 2 -> 3 -> 5
*
*
* bool use_relocate = 1;
*/
public Builder clearUseRelocate() {
useRelocate_ = false;
onChanged();
return this;
}
private boolean useRelocatePair_ ;
/**
*
* Operator which moves a pair of pickup and delivery nodes to another
* position where the first node of the pair must be before the second node
* on the same path.
* Possible neighbors for the path 1 -> A -> B -> 2 -> 3 (where (1, 3) are
* first and last nodes of the path and can therefore not be moved, and
* (A, B) is a pair of nodes):
* 1 -> [A] -> 2 -> [B] -> 3
* 1 -> 2 -> [A] -> [B] -> 3
*
*
* bool use_relocate_pair = 2;
*/
public boolean getUseRelocatePair() {
return useRelocatePair_;
}
/**
*
* Operator which moves a pair of pickup and delivery nodes to another
* position where the first node of the pair must be before the second node
* on the same path.
* Possible neighbors for the path 1 -> A -> B -> 2 -> 3 (where (1, 3) are
* first and last nodes of the path and can therefore not be moved, and
* (A, B) is a pair of nodes):
* 1 -> [A] -> 2 -> [B] -> 3
* 1 -> 2 -> [A] -> [B] -> 3
*
*
* bool use_relocate_pair = 2;
*/
public Builder setUseRelocatePair(boolean value) {
useRelocatePair_ = value;
onChanged();
return this;
}
/**
*
* Operator which moves a pair of pickup and delivery nodes to another
* position where the first node of the pair must be before the second node
* on the same path.
* Possible neighbors for the path 1 -> A -> B -> 2 -> 3 (where (1, 3) are
* first and last nodes of the path and can therefore not be moved, and
* (A, B) is a pair of nodes):
* 1 -> [A] -> 2 -> [B] -> 3
* 1 -> 2 -> [A] -> [B] -> 3
*
*
* bool use_relocate_pair = 2;
*/
public Builder clearUseRelocatePair() {
useRelocatePair_ = false;
onChanged();
return this;
}
private boolean useRelocateNeighbors_ ;
/**
*
* Relocate neighborhood which moves chains of neighbors.
* The operator starts by relocating a node n after a node m, then continues
* moving nodes which were after n as long as the "cost" added is less than
* the "cost" of the arc (m, n). If the new chain doesn't respect the domain
* of next variables, it will try reordering the nodes until it finds a
* valid path.
* Possible neighbors for path 1 -> A -> B -> C -> D -> E -> 2 (where (1, 2)
* are first and last nodes of the path and can therefore not be moved, A
* must be performed before B, and A, D and E are located at the same
* place):
* 1 -> A -> C -> [B] -> D -> E -> 2
* 1 -> A -> C -> D -> [B] -> E -> 2
* 1 -> A -> C -> D -> E -> [B] -> 2
* 1 -> A -> B -> D -> [C] -> E -> 2
* 1 -> A -> B -> D -> E -> [C] -> 2
* 1 -> A -> [D] -> [E] -> B -> C -> 2
* 1 -> A -> B -> [D] -> [E] -> C -> 2
* 1 -> A -> [E] -> B -> C -> D -> 2
* 1 -> A -> B -> [E] -> C -> D -> 2
* 1 -> A -> B -> C -> [E] -> D -> 2
* This operator is extremelly useful to move chains of nodes which are
* located at the same place (for instance nodes part of a same stop).
*
*
* bool use_relocate_neighbors = 3;
*/
public boolean getUseRelocateNeighbors() {
return useRelocateNeighbors_;
}
/**
*
* Relocate neighborhood which moves chains of neighbors.
* The operator starts by relocating a node n after a node m, then continues
* moving nodes which were after n as long as the "cost" added is less than
* the "cost" of the arc (m, n). If the new chain doesn't respect the domain
* of next variables, it will try reordering the nodes until it finds a
* valid path.
* Possible neighbors for path 1 -> A -> B -> C -> D -> E -> 2 (where (1, 2)
* are first and last nodes of the path and can therefore not be moved, A
* must be performed before B, and A, D and E are located at the same
* place):
* 1 -> A -> C -> [B] -> D -> E -> 2
* 1 -> A -> C -> D -> [B] -> E -> 2
* 1 -> A -> C -> D -> E -> [B] -> 2
* 1 -> A -> B -> D -> [C] -> E -> 2
* 1 -> A -> B -> D -> E -> [C] -> 2
* 1 -> A -> [D] -> [E] -> B -> C -> 2
* 1 -> A -> B -> [D] -> [E] -> C -> 2
* 1 -> A -> [E] -> B -> C -> D -> 2
* 1 -> A -> B -> [E] -> C -> D -> 2
* 1 -> A -> B -> C -> [E] -> D -> 2
* This operator is extremelly useful to move chains of nodes which are
* located at the same place (for instance nodes part of a same stop).
*
*
* bool use_relocate_neighbors = 3;
*/
public Builder setUseRelocateNeighbors(boolean value) {
useRelocateNeighbors_ = value;
onChanged();
return this;
}
/**
*
* Relocate neighborhood which moves chains of neighbors.
* The operator starts by relocating a node n after a node m, then continues
* moving nodes which were after n as long as the "cost" added is less than
* the "cost" of the arc (m, n). If the new chain doesn't respect the domain
* of next variables, it will try reordering the nodes until it finds a
* valid path.
* Possible neighbors for path 1 -> A -> B -> C -> D -> E -> 2 (where (1, 2)
* are first and last nodes of the path and can therefore not be moved, A
* must be performed before B, and A, D and E are located at the same
* place):
* 1 -> A -> C -> [B] -> D -> E -> 2
* 1 -> A -> C -> D -> [B] -> E -> 2
* 1 -> A -> C -> D -> E -> [B] -> 2
* 1 -> A -> B -> D -> [C] -> E -> 2
* 1 -> A -> B -> D -> E -> [C] -> 2
* 1 -> A -> [D] -> [E] -> B -> C -> 2
* 1 -> A -> B -> [D] -> [E] -> C -> 2
* 1 -> A -> [E] -> B -> C -> D -> 2
* 1 -> A -> B -> [E] -> C -> D -> 2
* 1 -> A -> B -> C -> [E] -> D -> 2
* This operator is extremelly useful to move chains of nodes which are
* located at the same place (for instance nodes part of a same stop).
*
*
* bool use_relocate_neighbors = 3;
*/
public Builder clearUseRelocateNeighbors() {
useRelocateNeighbors_ = false;
onChanged();
return this;
}
private boolean useExchange_ ;
/**
*
* Operator which exchanges the positions of two nodes.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3] -> [2] -> 4 -> 5
* 1 -> [4] -> 3 -> [2] -> 5
* 1 -> 2 -> [4] -> [3] -> 5
*
*
* bool use_exchange = 4;
*/
public boolean getUseExchange() {
return useExchange_;
}
/**
*
* Operator which exchanges the positions of two nodes.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3] -> [2] -> 4 -> 5
* 1 -> [4] -> 3 -> [2] -> 5
* 1 -> 2 -> [4] -> [3] -> 5
*
*
* bool use_exchange = 4;
*/
public Builder setUseExchange(boolean value) {
useExchange_ = value;
onChanged();
return this;
}
/**
*
* Operator which exchanges the positions of two nodes.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3] -> [2] -> 4 -> 5
* 1 -> [4] -> 3 -> [2] -> 5
* 1 -> 2 -> [4] -> [3] -> 5
*
*
* bool use_exchange = 4;
*/
public Builder clearUseExchange() {
useExchange_ = false;
onChanged();
return this;
}
private boolean useCross_ ;
/**
*
* Operator which cross exchanges the starting chains of 2 paths, including
* exchanging the whole paths.
* First and last nodes are not moved.
* Possible neighbors for the paths 1 -> 2 -> 3 -> 4 -> 5 and 6 -> 7 -> 8
* (where (1, 5) and (6, 8) are first and last nodes of the paths and can
* therefore not be moved):
* 1 -> [7] -> 3 -> 4 -> 5 6 -> [2] -> 8
* 1 -> [7] -> 4 -> 5 6 -> [2 -> 3] -> 8
* 1 -> [7] -> 5 6 -> [2 -> 3 -> 4] -> 8
*
*
* bool use_cross = 5;
*/
public boolean getUseCross() {
return useCross_;
}
/**
*
* Operator which cross exchanges the starting chains of 2 paths, including
* exchanging the whole paths.
* First and last nodes are not moved.
* Possible neighbors for the paths 1 -> 2 -> 3 -> 4 -> 5 and 6 -> 7 -> 8
* (where (1, 5) and (6, 8) are first and last nodes of the paths and can
* therefore not be moved):
* 1 -> [7] -> 3 -> 4 -> 5 6 -> [2] -> 8
* 1 -> [7] -> 4 -> 5 6 -> [2 -> 3] -> 8
* 1 -> [7] -> 5 6 -> [2 -> 3 -> 4] -> 8
*
*
* bool use_cross = 5;
*/
public Builder setUseCross(boolean value) {
useCross_ = value;
onChanged();
return this;
}
/**
*
* Operator which cross exchanges the starting chains of 2 paths, including
* exchanging the whole paths.
* First and last nodes are not moved.
* Possible neighbors for the paths 1 -> 2 -> 3 -> 4 -> 5 and 6 -> 7 -> 8
* (where (1, 5) and (6, 8) are first and last nodes of the paths and can
* therefore not be moved):
* 1 -> [7] -> 3 -> 4 -> 5 6 -> [2] -> 8
* 1 -> [7] -> 4 -> 5 6 -> [2 -> 3] -> 8
* 1 -> [7] -> 5 6 -> [2 -> 3 -> 4] -> 8
*
*
* bool use_cross = 5;
*/
public Builder clearUseCross() {
useCross_ = false;
onChanged();
return this;
}
private boolean useCrossExchange_ ;
/**
*
* Not implemented as of 10/2015.
*
*
* bool use_cross_exchange = 6;
*/
public boolean getUseCrossExchange() {
return useCrossExchange_;
}
/**
*
* Not implemented as of 10/2015.
*
*
* bool use_cross_exchange = 6;
*/
public Builder setUseCrossExchange(boolean value) {
useCrossExchange_ = value;
onChanged();
return this;
}
/**
*
* Not implemented as of 10/2015.
*
*
* bool use_cross_exchange = 6;
*/
public Builder clearUseCrossExchange() {
useCrossExchange_ = false;
onChanged();
return this;
}
private boolean useTwoOpt_ ;
/**
*
* --- Intra-route operators ---
* Operator which reverves a sub-chain of a path. It is called TwoOpt
* because it breaks two arcs on the path; resulting paths are called
* two-optimal.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3 -> 2] -> 4 -> 5
* 1 -> [4 -> 3 -> 2] -> 5
* 1 -> 2 -> [4 -> 3] -> 5
*
*
* bool use_two_opt = 7;
*/
public boolean getUseTwoOpt() {
return useTwoOpt_;
}
/**
*
* --- Intra-route operators ---
* Operator which reverves a sub-chain of a path. It is called TwoOpt
* because it breaks two arcs on the path; resulting paths are called
* two-optimal.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3 -> 2] -> 4 -> 5
* 1 -> [4 -> 3 -> 2] -> 5
* 1 -> 2 -> [4 -> 3] -> 5
*
*
* bool use_two_opt = 7;
*/
public Builder setUseTwoOpt(boolean value) {
useTwoOpt_ = value;
onChanged();
return this;
}
/**
*
* --- Intra-route operators ---
* Operator which reverves a sub-chain of a path. It is called TwoOpt
* because it breaks two arcs on the path; resulting paths are called
* two-optimal.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3 -> 2] -> 4 -> 5
* 1 -> [4 -> 3 -> 2] -> 5
* 1 -> 2 -> [4 -> 3] -> 5
*
*
* bool use_two_opt = 7;
*/
public Builder clearUseTwoOpt() {
useTwoOpt_ = false;
onChanged();
return this;
}
private boolean useOrOpt_ ;
/**
*
* Operator which moves sub-chains of a path of length 1, 2 and 3 to another
* position in the same path.
* When the length of the sub-chain is 1, the operator simply moves a node
* to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5, for a sub-chain
* length of 2 (where (1, 5) are first and last nodes of the path and can
* therefore not be moved):
* 1 -> 4 -> [2 -> 3] -> 5
* 1 -> [3 -> 4] -> 2 -> 5
* The OR_OPT operator is a limited version of 3-Opt (breaks 3 arcs on a
* path).
*
*
* bool use_or_opt = 8;
*/
public boolean getUseOrOpt() {
return useOrOpt_;
}
/**
*
* Operator which moves sub-chains of a path of length 1, 2 and 3 to another
* position in the same path.
* When the length of the sub-chain is 1, the operator simply moves a node
* to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5, for a sub-chain
* length of 2 (where (1, 5) are first and last nodes of the path and can
* therefore not be moved):
* 1 -> 4 -> [2 -> 3] -> 5
* 1 -> [3 -> 4] -> 2 -> 5
* The OR_OPT operator is a limited version of 3-Opt (breaks 3 arcs on a
* path).
*
*
* bool use_or_opt = 8;
*/
public Builder setUseOrOpt(boolean value) {
useOrOpt_ = value;
onChanged();
return this;
}
/**
*
* Operator which moves sub-chains of a path of length 1, 2 and 3 to another
* position in the same path.
* When the length of the sub-chain is 1, the operator simply moves a node
* to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5, for a sub-chain
* length of 2 (where (1, 5) are first and last nodes of the path and can
* therefore not be moved):
* 1 -> 4 -> [2 -> 3] -> 5
* 1 -> [3 -> 4] -> 2 -> 5
* The OR_OPT operator is a limited version of 3-Opt (breaks 3 arcs on a
* path).
*
*
* bool use_or_opt = 8;
*/
public Builder clearUseOrOpt() {
useOrOpt_ = false;
onChanged();
return this;
}
private boolean useLinKernighan_ ;
/**
*
* Lin-Kernighan operator.
* While the accumulated local gain is positive, performs a 2-OPT or a 3-OPT
* move followed by a series of 2-OPT moves. Returns a neighbor for which
* the global gain is positive.
*
*
* bool use_lin_kernighan = 9;
*/
public boolean getUseLinKernighan() {
return useLinKernighan_;
}
/**
*
* Lin-Kernighan operator.
* While the accumulated local gain is positive, performs a 2-OPT or a 3-OPT
* move followed by a series of 2-OPT moves. Returns a neighbor for which
* the global gain is positive.
*
*
* bool use_lin_kernighan = 9;
*/
public Builder setUseLinKernighan(boolean value) {
useLinKernighan_ = value;
onChanged();
return this;
}
/**
*
* Lin-Kernighan operator.
* While the accumulated local gain is positive, performs a 2-OPT or a 3-OPT
* move followed by a series of 2-OPT moves. Returns a neighbor for which
* the global gain is positive.
*
*
* bool use_lin_kernighan = 9;
*/
public Builder clearUseLinKernighan() {
useLinKernighan_ = false;
onChanged();
return this;
}
private boolean useTspOpt_ ;
/**
*
* Sliding TSP operator.
* Uses an exact dynamic programming algorithm to solve the TSP
* corresponding to path sub-chains.
* For a subchain 1 -> 2 -> 3 -> 4 -> 5 -> 6, solves the TSP on
* nodes A, 2, 3, 4, 5, where A is a merger of nodes 1 and 6 such that
* cost(A,i) = cost(1,i) and cost(i,A) = cost(i,6).
*
*
* bool use_tsp_opt = 10;
*/
public boolean getUseTspOpt() {
return useTspOpt_;
}
/**
*
* Sliding TSP operator.
* Uses an exact dynamic programming algorithm to solve the TSP
* corresponding to path sub-chains.
* For a subchain 1 -> 2 -> 3 -> 4 -> 5 -> 6, solves the TSP on
* nodes A, 2, 3, 4, 5, where A is a merger of nodes 1 and 6 such that
* cost(A,i) = cost(1,i) and cost(i,A) = cost(i,6).
*
*
* bool use_tsp_opt = 10;
*/
public Builder setUseTspOpt(boolean value) {
useTspOpt_ = value;
onChanged();
return this;
}
/**
*
* Sliding TSP operator.
* Uses an exact dynamic programming algorithm to solve the TSP
* corresponding to path sub-chains.
* For a subchain 1 -> 2 -> 3 -> 4 -> 5 -> 6, solves the TSP on
* nodes A, 2, 3, 4, 5, where A is a merger of nodes 1 and 6 such that
* cost(A,i) = cost(1,i) and cost(i,A) = cost(i,6).
*
*
* bool use_tsp_opt = 10;
*/
public Builder clearUseTspOpt() {
useTspOpt_ = false;
onChanged();
return this;
}
private boolean useMakeActive_ ;
/**
*
* --- Operators on inactive nodes ---
* Operator which inserts an inactive node into a path.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 2 -> 3 -> 4
* 1 -> 2 -> [5] -> 3 -> 4
* 1 -> 2 -> 3 -> [5] -> 4
*
*
* bool use_make_active = 11;
*/
public boolean getUseMakeActive() {
return useMakeActive_;
}
/**
*
* --- Operators on inactive nodes ---
* Operator which inserts an inactive node into a path.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 2 -> 3 -> 4
* 1 -> 2 -> [5] -> 3 -> 4
* 1 -> 2 -> 3 -> [5] -> 4
*
*
* bool use_make_active = 11;
*/
public Builder setUseMakeActive(boolean value) {
useMakeActive_ = value;
onChanged();
return this;
}
/**
*
* --- Operators on inactive nodes ---
* Operator which inserts an inactive node into a path.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 2 -> 3 -> 4
* 1 -> 2 -> [5] -> 3 -> 4
* 1 -> 2 -> 3 -> [5] -> 4
*
*
* bool use_make_active = 11;
*/
public Builder clearUseMakeActive() {
useMakeActive_ = false;
onChanged();
return this;
}
private boolean useRelocateAndMakeActive_ ;
/**
*
* Operator which relocates a node while making an inactive one active.
* As of 3/2017, the operator is limited to two kinds of moves:
* - Relocating a node and replacing it by an inactive node.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 3 -> 5, 2 -> 4 -> 6.
* - Relocating a node and inserting an inactive node next to it.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 4 -> 3 -> 5, 2 -> 6.
*
*
* bool use_relocate_and_make_active = 21;
*/
public boolean getUseRelocateAndMakeActive() {
return useRelocateAndMakeActive_;
}
/**
*
* Operator which relocates a node while making an inactive one active.
* As of 3/2017, the operator is limited to two kinds of moves:
* - Relocating a node and replacing it by an inactive node.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 3 -> 5, 2 -> 4 -> 6.
* - Relocating a node and inserting an inactive node next to it.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 4 -> 3 -> 5, 2 -> 6.
*
*
* bool use_relocate_and_make_active = 21;
*/
public Builder setUseRelocateAndMakeActive(boolean value) {
useRelocateAndMakeActive_ = value;
onChanged();
return this;
}
/**
*
* Operator which relocates a node while making an inactive one active.
* As of 3/2017, the operator is limited to two kinds of moves:
* - Relocating a node and replacing it by an inactive node.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 3 -> 5, 2 -> 4 -> 6.
* - Relocating a node and inserting an inactive node next to it.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 4 -> 3 -> 5, 2 -> 6.
*
*
* bool use_relocate_and_make_active = 21;
*/
public Builder clearUseRelocateAndMakeActive() {
useRelocateAndMakeActive_ = false;
onChanged();
return this;
}
private boolean useMakeInactive_ ;
/**
*
* Operator which makes path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
*
*
* bool use_make_inactive = 12;
*/
public boolean getUseMakeInactive() {
return useMakeInactive_;
}
/**
*
* Operator which makes path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
*
*
* bool use_make_inactive = 12;
*/
public Builder setUseMakeInactive(boolean value) {
useMakeInactive_ = value;
onChanged();
return this;
}
/**
*
* Operator which makes path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
*
*
* bool use_make_inactive = 12;
*/
public Builder clearUseMakeInactive() {
useMakeInactive_ = false;
onChanged();
return this;
}
private boolean useMakeChainInactive_ ;
/**
*
* Operator which makes a "chain" of path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
* 1 -> 4 with 2 and 3 inactive
*
*
* bool use_make_chain_inactive = 13;
*/
public boolean getUseMakeChainInactive() {
return useMakeChainInactive_;
}
/**
*
* Operator which makes a "chain" of path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
* 1 -> 4 with 2 and 3 inactive
*
*
* bool use_make_chain_inactive = 13;
*/
public Builder setUseMakeChainInactive(boolean value) {
useMakeChainInactive_ = value;
onChanged();
return this;
}
/**
*
* Operator which makes a "chain" of path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
* 1 -> 4 with 2 and 3 inactive
*
*
* bool use_make_chain_inactive = 13;
*/
public Builder clearUseMakeChainInactive() {
useMakeChainInactive_ = false;
onChanged();
return this;
}
private boolean useSwapActive_ ;
/**
*
* Operator which replaces an active node by an inactive one.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* bool use_swap_active = 14;
*/
public boolean getUseSwapActive() {
return useSwapActive_;
}
/**
*
* Operator which replaces an active node by an inactive one.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* bool use_swap_active = 14;
*/
public Builder setUseSwapActive(boolean value) {
useSwapActive_ = value;
onChanged();
return this;
}
/**
*
* Operator which replaces an active node by an inactive one.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* bool use_swap_active = 14;
*/
public Builder clearUseSwapActive() {
useSwapActive_ = false;
onChanged();
return this;
}
private boolean useExtendedSwapActive_ ;
/**
*
* Operator which makes an inactive node active and an active one inactive.
* It is similar to SwapActiveOperator excepts that it tries to insert the
* inactive node in all possible positions instead of just the position of
* the node made inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 3 -> [5] -> 4 with 2 inactive
* 1 -> [5] -> 2 -> 4 with 3 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* bool use_extended_swap_active = 15;
*/
public boolean getUseExtendedSwapActive() {
return useExtendedSwapActive_;
}
/**
*
* Operator which makes an inactive node active and an active one inactive.
* It is similar to SwapActiveOperator excepts that it tries to insert the
* inactive node in all possible positions instead of just the position of
* the node made inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 3 -> [5] -> 4 with 2 inactive
* 1 -> [5] -> 2 -> 4 with 3 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* bool use_extended_swap_active = 15;
*/
public Builder setUseExtendedSwapActive(boolean value) {
useExtendedSwapActive_ = value;
onChanged();
return this;
}
/**
*
* Operator which makes an inactive node active and an active one inactive.
* It is similar to SwapActiveOperator excepts that it tries to insert the
* inactive node in all possible positions instead of just the position of
* the node made inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 3 -> [5] -> 4 with 2 inactive
* 1 -> [5] -> 2 -> 4 with 3 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* bool use_extended_swap_active = 15;
*/
public Builder clearUseExtendedSwapActive() {
useExtendedSwapActive_ = false;
onChanged();
return this;
}
private boolean useNodePairSwapActive_ ;
/**
*
* Operator which makes an inactive node active and an active pair of nodes
* inactive OR makes an inactive pair of nodes active and an active node
* inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path and (2,3) is a pair
* of nodes) are:
* 1 -> [5] -> 4 with (2,3) inactive
* Possible neighbors for the path 1 -> 2 -> 3 with (4,5) inactive
* (where 1 and 3 are first and last nodes of the path and (4,5) is a pair
* of nodes) are:
* 1 -> [4] -> [5] -> 3 with 2 inactive
*
*
* bool use_node_pair_swap_active = 20;
*/
public boolean getUseNodePairSwapActive() {
return useNodePairSwapActive_;
}
/**
*
* Operator which makes an inactive node active and an active pair of nodes
* inactive OR makes an inactive pair of nodes active and an active node
* inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path and (2,3) is a pair
* of nodes) are:
* 1 -> [5] -> 4 with (2,3) inactive
* Possible neighbors for the path 1 -> 2 -> 3 with (4,5) inactive
* (where 1 and 3 are first and last nodes of the path and (4,5) is a pair
* of nodes) are:
* 1 -> [4] -> [5] -> 3 with 2 inactive
*
*
* bool use_node_pair_swap_active = 20;
*/
public Builder setUseNodePairSwapActive(boolean value) {
useNodePairSwapActive_ = value;
onChanged();
return this;
}
/**
*
* Operator which makes an inactive node active and an active pair of nodes
* inactive OR makes an inactive pair of nodes active and an active node
* inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path and (2,3) is a pair
* of nodes) are:
* 1 -> [5] -> 4 with (2,3) inactive
* Possible neighbors for the path 1 -> 2 -> 3 with (4,5) inactive
* (where 1 and 3 are first and last nodes of the path and (4,5) is a pair
* of nodes) are:
* 1 -> [4] -> [5] -> 3 with 2 inactive
*
*
* bool use_node_pair_swap_active = 20;
*/
public Builder clearUseNodePairSwapActive() {
useNodePairSwapActive_ = false;
onChanged();
return this;
}
private boolean usePathLns_ ;
/**
*
* --- Large neighborhood search operators ---
* Operator which relaxes two sub-chains of three consecutive arcs each.
* Each sub-chain is defined by a start node and the next three arcs. Those
* six arcs are relaxed to build a new neighbor.
* PATH_LNS explores all possible pairs of starting nodes and so defines
* n^2 neighbors, n being the number of nodes.
* Note that the two sub-chains can be part of the same path; they even may
* overlap.
*
*
* bool use_path_lns = 16;
*/
public boolean getUsePathLns() {
return usePathLns_;
}
/**
*
* --- Large neighborhood search operators ---
* Operator which relaxes two sub-chains of three consecutive arcs each.
* Each sub-chain is defined by a start node and the next three arcs. Those
* six arcs are relaxed to build a new neighbor.
* PATH_LNS explores all possible pairs of starting nodes and so defines
* n^2 neighbors, n being the number of nodes.
* Note that the two sub-chains can be part of the same path; they even may
* overlap.
*
*
* bool use_path_lns = 16;
*/
public Builder setUsePathLns(boolean value) {
usePathLns_ = value;
onChanged();
return this;
}
/**
*
* --- Large neighborhood search operators ---
* Operator which relaxes two sub-chains of three consecutive arcs each.
* Each sub-chain is defined by a start node and the next three arcs. Those
* six arcs are relaxed to build a new neighbor.
* PATH_LNS explores all possible pairs of starting nodes and so defines
* n^2 neighbors, n being the number of nodes.
* Note that the two sub-chains can be part of the same path; they even may
* overlap.
*
*
* bool use_path_lns = 16;
*/
public Builder clearUsePathLns() {
usePathLns_ = false;
onChanged();
return this;
}
private boolean useFullPathLns_ ;
/**
*
* Operator which relaxes one entire path and all unactive nodes.
*
*
* bool use_full_path_lns = 17;
*/
public boolean getUseFullPathLns() {
return useFullPathLns_;
}
/**
*
* Operator which relaxes one entire path and all unactive nodes.
*
*
* bool use_full_path_lns = 17;
*/
public Builder setUseFullPathLns(boolean value) {
useFullPathLns_ = value;
onChanged();
return this;
}
/**
*
* Operator which relaxes one entire path and all unactive nodes.
*
*
* bool use_full_path_lns = 17;
*/
public Builder clearUseFullPathLns() {
useFullPathLns_ = false;
onChanged();
return this;
}
private boolean useTspLns_ ;
/**
*
* TSP-base LNS.
* Randomly merges consecutive nodes until n "meta"-nodes remain and solves
* the corresponding TSP.
* This defines an "unlimited" neighborhood which must be stopped by search
* limits. To force diversification, the operator iteratively forces each
* node to serve as base of a meta-node.
*
*
* bool use_tsp_lns = 18;
*/
public boolean getUseTspLns() {
return useTspLns_;
}
/**
*
* TSP-base LNS.
* Randomly merges consecutive nodes until n "meta"-nodes remain and solves
* the corresponding TSP.
* This defines an "unlimited" neighborhood which must be stopped by search
* limits. To force diversification, the operator iteratively forces each
* node to serve as base of a meta-node.
*
*
* bool use_tsp_lns = 18;
*/
public Builder setUseTspLns(boolean value) {
useTspLns_ = value;
onChanged();
return this;
}
/**
*
* TSP-base LNS.
* Randomly merges consecutive nodes until n "meta"-nodes remain and solves
* the corresponding TSP.
* This defines an "unlimited" neighborhood which must be stopped by search
* limits. To force diversification, the operator iteratively forces each
* node to serve as base of a meta-node.
*
*
* bool use_tsp_lns = 18;
*/
public Builder clearUseTspLns() {
useTspLns_ = false;
onChanged();
return this;
}
private boolean useInactiveLns_ ;
/**
*
* Operator which relaxes all inactive nodes and one sub-chain of six
* consecutive arcs. That way the path can be improved by inserting inactive
* nodes or swaping arcs.
*
*
* bool use_inactive_lns = 19;
*/
public boolean getUseInactiveLns() {
return useInactiveLns_;
}
/**
*
* Operator which relaxes all inactive nodes and one sub-chain of six
* consecutive arcs. That way the path can be improved by inserting inactive
* nodes or swaping arcs.
*
*
* bool use_inactive_lns = 19;
*/
public Builder setUseInactiveLns(boolean value) {
useInactiveLns_ = value;
onChanged();
return this;
}
/**
*
* Operator which relaxes all inactive nodes and one sub-chain of six
* consecutive arcs. That way the path can be improved by inserting inactive
* nodes or swaping arcs.
*
*
* bool use_inactive_lns = 19;
*/
public Builder clearUseInactiveLns() {
useInactiveLns_ = false;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators)
}
// @@protoc_insertion_point(class_scope:operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators)
private static final com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators();
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public LocalSearchNeighborhoodOperators parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LocalSearchNeighborhoodOperators(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int FIRST_SOLUTION_STRATEGY_FIELD_NUMBER = 1;
private int firstSolutionStrategy_;
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
*/
public int getFirstSolutionStrategyValue() {
return firstSolutionStrategy_;
}
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
*/
public com.google.ortools.constraintsolver.FirstSolutionStrategy.Value getFirstSolutionStrategy() {
com.google.ortools.constraintsolver.FirstSolutionStrategy.Value result = com.google.ortools.constraintsolver.FirstSolutionStrategy.Value.valueOf(firstSolutionStrategy_);
return result == null ? com.google.ortools.constraintsolver.FirstSolutionStrategy.Value.UNRECOGNIZED : result;
}
public static final int USE_FILTERED_FIRST_SOLUTION_STRATEGY_FIELD_NUMBER = 2;
private boolean useFilteredFirstSolutionStrategy_;
/**
*
* These are advanced first solutions strategy settings which should not be
* modified unless you know what you are doing.
* Use filtered version of first solution strategy if available.
*
*
* bool use_filtered_first_solution_strategy = 2;
*/
public boolean getUseFilteredFirstSolutionStrategy() {
return useFilteredFirstSolutionStrategy_;
}
public static final int SAVINGS_NEIGHBORS_RATIO_FIELD_NUMBER = 14;
private double savingsNeighborsRatio_;
/**
*
* Parameters specific to the Savings first solution heuristic.
* Ratio (between 0 and 1) of neighbors to consider for each node when
* constructing the savings. If <= 0 or greater than 1, its value is
* considered to be 1.0.
*
*
* double savings_neighbors_ratio = 14;
*/
public double getSavingsNeighborsRatio() {
return savingsNeighborsRatio_;
}
public static final int SAVINGS_ADD_REVERSE_ARCS_FIELD_NUMBER = 15;
private boolean savingsAddReverseArcs_;
/**
*
* Add savings related to reverse arcs when finding the nearest neighbors
* of the nodes.
*
*
* bool savings_add_reverse_arcs = 15;
*/
public boolean getSavingsAddReverseArcs() {
return savingsAddReverseArcs_;
}
public static final int LOCAL_SEARCH_OPERATORS_FIELD_NUMBER = 3;
private com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators localSearchOperators_;
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public boolean hasLocalSearchOperators() {
return localSearchOperators_ != null;
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators getLocalSearchOperators() {
return localSearchOperators_ == null ? com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.getDefaultInstance() : localSearchOperators_;
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperatorsOrBuilder getLocalSearchOperatorsOrBuilder() {
return getLocalSearchOperators();
}
public static final int LOCAL_SEARCH_METAHEURISTIC_FIELD_NUMBER = 4;
private int localSearchMetaheuristic_;
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
*/
public int getLocalSearchMetaheuristicValue() {
return localSearchMetaheuristic_;
}
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
*/
public com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value getLocalSearchMetaheuristic() {
com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value result = com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value.valueOf(localSearchMetaheuristic_);
return result == null ? com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value.UNRECOGNIZED : result;
}
public static final int GUIDED_LOCAL_SEARCH_LAMBDA_COEFFICIENT_FIELD_NUMBER = 5;
private double guidedLocalSearchLambdaCoefficient_;
/**
*
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Lambda coefficient used to penalize arc costs when GUIDED_LOCAL_SEARCH is
* used.
*
*
* double guided_local_search_lambda_coefficient = 5;
*/
public double getGuidedLocalSearchLambdaCoefficient() {
return guidedLocalSearchLambdaCoefficient_;
}
public static final int USE_DEPTH_FIRST_SEARCH_FIELD_NUMBER = 6;
private boolean useDepthFirstSearch_;
/**
*
* --- Search control ---
* If true, the solver should use depth-first search rather than local search
* to solve the problem.
*
*
* bool use_depth_first_search = 6;
*/
public boolean getUseDepthFirstSearch() {
return useDepthFirstSearch_;
}
public static final int OPTIMIZATION_STEP_FIELD_NUMBER = 7;
private long optimizationStep_;
/**
*
* Minimum step by which the solution must be improved in local search.
*
*
* int64 optimization_step = 7;
*/
public long getOptimizationStep() {
return optimizationStep_;
}
public static final int SOLUTION_LIMIT_FIELD_NUMBER = 8;
private long solutionLimit_;
/**
*
* -- Search limits --
* Limit to the number of solutions generated during the search.
*
*
* int64 solution_limit = 8;
*/
public long getSolutionLimit() {
return solutionLimit_;
}
public static final int TIME_LIMIT_MS_FIELD_NUMBER = 9;
private long timeLimitMs_;
/**
*
* Limit in milliseconds to the time spent in the search.
*
*
* int64 time_limit_ms = 9;
*/
public long getTimeLimitMs() {
return timeLimitMs_;
}
public static final int LNS_TIME_LIMIT_MS_FIELD_NUMBER = 10;
private long lnsTimeLimitMs_;
/**
*
* Limit in milliseconds to the time spent in the completion search for each
* local search neighbor.
*
*
* int64 lns_time_limit_ms = 10;
*/
public long getLnsTimeLimitMs() {
return lnsTimeLimitMs_;
}
public static final int USE_LIGHT_PROPAGATION_FIELD_NUMBER = 11;
private boolean useLightPropagation_;
/**
*
* --- Propagation control ---
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Use constraints with light propagation in routing model. Extra propagation
* is only necessary when using depth-first search or for models which
* require strong propagation to finalize the value of secondary variables.
* Changing this setting to true will slow down the search in most cases and
* increase memory consumption in all cases.
*
*
* bool use_light_propagation = 11;
*/
public boolean getUseLightPropagation() {
return useLightPropagation_;
}
public static final int FINGERPRINT_ARC_COST_EVALUATORS_FIELD_NUMBER = 12;
private boolean fingerprintArcCostEvaluators_;
/**
*
* --- Miscellaneous ---
* Some of these are advanced settings which should not be modified unless you
* know what you are doing.
* If true, arc cost evaluators will be fingerprinted. The fingerprint will
* be used when computing vehicle cost equivalent classes, otherwise the
* address of the evaluator will be used.
*
*
* bool fingerprint_arc_cost_evaluators = 12;
*/
public boolean getFingerprintArcCostEvaluators() {
return fingerprintArcCostEvaluators_;
}
public static final int LOG_SEARCH_FIELD_NUMBER = 13;
private boolean logSearch_;
/**
*
* Activates search logging. For each solution found during the search, the
* following will be displayed: its objective value, the maximum objective
* value since the beginning of the search, the elapsed time since the
* beginning of the search, the number of branches explored in the search
* tree, the number of failures in the search tree, the depth of the search
* tree, the number of local search neighbors explored, the number of local
* search neighbors filtered by local search filters, the number of local
* search neighbors accepted, the total memory used and the percentage of the
* search done.
*
*
* bool log_search = 13;
*/
public boolean getLogSearch() {
return logSearch_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (firstSolutionStrategy_ != com.google.ortools.constraintsolver.FirstSolutionStrategy.Value.AUTOMATIC.getNumber()) {
output.writeEnum(1, firstSolutionStrategy_);
}
if (useFilteredFirstSolutionStrategy_ != false) {
output.writeBool(2, useFilteredFirstSolutionStrategy_);
}
if (localSearchOperators_ != null) {
output.writeMessage(3, getLocalSearchOperators());
}
if (localSearchMetaheuristic_ != com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value.AUTOMATIC.getNumber()) {
output.writeEnum(4, localSearchMetaheuristic_);
}
if (guidedLocalSearchLambdaCoefficient_ != 0D) {
output.writeDouble(5, guidedLocalSearchLambdaCoefficient_);
}
if (useDepthFirstSearch_ != false) {
output.writeBool(6, useDepthFirstSearch_);
}
if (optimizationStep_ != 0L) {
output.writeInt64(7, optimizationStep_);
}
if (solutionLimit_ != 0L) {
output.writeInt64(8, solutionLimit_);
}
if (timeLimitMs_ != 0L) {
output.writeInt64(9, timeLimitMs_);
}
if (lnsTimeLimitMs_ != 0L) {
output.writeInt64(10, lnsTimeLimitMs_);
}
if (useLightPropagation_ != false) {
output.writeBool(11, useLightPropagation_);
}
if (fingerprintArcCostEvaluators_ != false) {
output.writeBool(12, fingerprintArcCostEvaluators_);
}
if (logSearch_ != false) {
output.writeBool(13, logSearch_);
}
if (savingsNeighborsRatio_ != 0D) {
output.writeDouble(14, savingsNeighborsRatio_);
}
if (savingsAddReverseArcs_ != false) {
output.writeBool(15, savingsAddReverseArcs_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (firstSolutionStrategy_ != com.google.ortools.constraintsolver.FirstSolutionStrategy.Value.AUTOMATIC.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, firstSolutionStrategy_);
}
if (useFilteredFirstSolutionStrategy_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, useFilteredFirstSolutionStrategy_);
}
if (localSearchOperators_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getLocalSearchOperators());
}
if (localSearchMetaheuristic_ != com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value.AUTOMATIC.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, localSearchMetaheuristic_);
}
if (guidedLocalSearchLambdaCoefficient_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(5, guidedLocalSearchLambdaCoefficient_);
}
if (useDepthFirstSearch_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(6, useDepthFirstSearch_);
}
if (optimizationStep_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(7, optimizationStep_);
}
if (solutionLimit_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(8, solutionLimit_);
}
if (timeLimitMs_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(9, timeLimitMs_);
}
if (lnsTimeLimitMs_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(10, lnsTimeLimitMs_);
}
if (useLightPropagation_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(11, useLightPropagation_);
}
if (fingerprintArcCostEvaluators_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(12, fingerprintArcCostEvaluators_);
}
if (logSearch_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, logSearch_);
}
if (savingsNeighborsRatio_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(14, savingsNeighborsRatio_);
}
if (savingsAddReverseArcs_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(15, savingsAddReverseArcs_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ortools.constraintsolver.RoutingSearchParameters)) {
return super.equals(obj);
}
com.google.ortools.constraintsolver.RoutingSearchParameters other = (com.google.ortools.constraintsolver.RoutingSearchParameters) obj;
boolean result = true;
result = result && firstSolutionStrategy_ == other.firstSolutionStrategy_;
result = result && (getUseFilteredFirstSolutionStrategy()
== other.getUseFilteredFirstSolutionStrategy());
result = result && (
java.lang.Double.doubleToLongBits(getSavingsNeighborsRatio())
== java.lang.Double.doubleToLongBits(
other.getSavingsNeighborsRatio()));
result = result && (getSavingsAddReverseArcs()
== other.getSavingsAddReverseArcs());
result = result && (hasLocalSearchOperators() == other.hasLocalSearchOperators());
if (hasLocalSearchOperators()) {
result = result && getLocalSearchOperators()
.equals(other.getLocalSearchOperators());
}
result = result && localSearchMetaheuristic_ == other.localSearchMetaheuristic_;
result = result && (
java.lang.Double.doubleToLongBits(getGuidedLocalSearchLambdaCoefficient())
== java.lang.Double.doubleToLongBits(
other.getGuidedLocalSearchLambdaCoefficient()));
result = result && (getUseDepthFirstSearch()
== other.getUseDepthFirstSearch());
result = result && (getOptimizationStep()
== other.getOptimizationStep());
result = result && (getSolutionLimit()
== other.getSolutionLimit());
result = result && (getTimeLimitMs()
== other.getTimeLimitMs());
result = result && (getLnsTimeLimitMs()
== other.getLnsTimeLimitMs());
result = result && (getUseLightPropagation()
== other.getUseLightPropagation());
result = result && (getFingerprintArcCostEvaluators()
== other.getFingerprintArcCostEvaluators());
result = result && (getLogSearch()
== other.getLogSearch());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FIRST_SOLUTION_STRATEGY_FIELD_NUMBER;
hash = (53 * hash) + firstSolutionStrategy_;
hash = (37 * hash) + USE_FILTERED_FIRST_SOLUTION_STRATEGY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseFilteredFirstSolutionStrategy());
hash = (37 * hash) + SAVINGS_NEIGHBORS_RATIO_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getSavingsNeighborsRatio()));
hash = (37 * hash) + SAVINGS_ADD_REVERSE_ARCS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSavingsAddReverseArcs());
if (hasLocalSearchOperators()) {
hash = (37 * hash) + LOCAL_SEARCH_OPERATORS_FIELD_NUMBER;
hash = (53 * hash) + getLocalSearchOperators().hashCode();
}
hash = (37 * hash) + LOCAL_SEARCH_METAHEURISTIC_FIELD_NUMBER;
hash = (53 * hash) + localSearchMetaheuristic_;
hash = (37 * hash) + GUIDED_LOCAL_SEARCH_LAMBDA_COEFFICIENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getGuidedLocalSearchLambdaCoefficient()));
hash = (37 * hash) + USE_DEPTH_FIRST_SEARCH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseDepthFirstSearch());
hash = (37 * hash) + OPTIMIZATION_STEP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOptimizationStep());
hash = (37 * hash) + SOLUTION_LIMIT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSolutionLimit());
hash = (37 * hash) + TIME_LIMIT_MS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimeLimitMs());
hash = (37 * hash) + LNS_TIME_LIMIT_MS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLnsTimeLimitMs());
hash = (37 * hash) + USE_LIGHT_PROPAGATION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseLightPropagation());
hash = (37 * hash) + FINGERPRINT_ARC_COST_EVALUATORS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getFingerprintArcCostEvaluators());
hash = (37 * hash) + LOG_SEARCH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getLogSearch());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ortools.constraintsolver.RoutingSearchParameters prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Parameters defining the search used to solve vehicle routing problems.
*
*
* Protobuf type {@code operations_research.RoutingSearchParameters}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:operations_research.RoutingSearchParameters)
com.google.ortools.constraintsolver.RoutingSearchParametersOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.constraintsolver.RoutingSearchParameters.class, com.google.ortools.constraintsolver.RoutingSearchParameters.Builder.class);
}
// Construct using com.google.ortools.constraintsolver.RoutingSearchParameters.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
firstSolutionStrategy_ = 0;
useFilteredFirstSolutionStrategy_ = false;
savingsNeighborsRatio_ = 0D;
savingsAddReverseArcs_ = false;
if (localSearchOperatorsBuilder_ == null) {
localSearchOperators_ = null;
} else {
localSearchOperators_ = null;
localSearchOperatorsBuilder_ = null;
}
localSearchMetaheuristic_ = 0;
guidedLocalSearchLambdaCoefficient_ = 0D;
useDepthFirstSearch_ = false;
optimizationStep_ = 0L;
solutionLimit_ = 0L;
timeLimitMs_ = 0L;
lnsTimeLimitMs_ = 0L;
useLightPropagation_ = false;
fingerprintArcCostEvaluators_ = false;
logSearch_ = false;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_descriptor;
}
public com.google.ortools.constraintsolver.RoutingSearchParameters getDefaultInstanceForType() {
return com.google.ortools.constraintsolver.RoutingSearchParameters.getDefaultInstance();
}
public com.google.ortools.constraintsolver.RoutingSearchParameters build() {
com.google.ortools.constraintsolver.RoutingSearchParameters result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.ortools.constraintsolver.RoutingSearchParameters buildPartial() {
com.google.ortools.constraintsolver.RoutingSearchParameters result = new com.google.ortools.constraintsolver.RoutingSearchParameters(this);
result.firstSolutionStrategy_ = firstSolutionStrategy_;
result.useFilteredFirstSolutionStrategy_ = useFilteredFirstSolutionStrategy_;
result.savingsNeighborsRatio_ = savingsNeighborsRatio_;
result.savingsAddReverseArcs_ = savingsAddReverseArcs_;
if (localSearchOperatorsBuilder_ == null) {
result.localSearchOperators_ = localSearchOperators_;
} else {
result.localSearchOperators_ = localSearchOperatorsBuilder_.build();
}
result.localSearchMetaheuristic_ = localSearchMetaheuristic_;
result.guidedLocalSearchLambdaCoefficient_ = guidedLocalSearchLambdaCoefficient_;
result.useDepthFirstSearch_ = useDepthFirstSearch_;
result.optimizationStep_ = optimizationStep_;
result.solutionLimit_ = solutionLimit_;
result.timeLimitMs_ = timeLimitMs_;
result.lnsTimeLimitMs_ = lnsTimeLimitMs_;
result.useLightPropagation_ = useLightPropagation_;
result.fingerprintArcCostEvaluators_ = fingerprintArcCostEvaluators_;
result.logSearch_ = logSearch_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ortools.constraintsolver.RoutingSearchParameters) {
return mergeFrom((com.google.ortools.constraintsolver.RoutingSearchParameters)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ortools.constraintsolver.RoutingSearchParameters other) {
if (other == com.google.ortools.constraintsolver.RoutingSearchParameters.getDefaultInstance()) return this;
if (other.firstSolutionStrategy_ != 0) {
setFirstSolutionStrategyValue(other.getFirstSolutionStrategyValue());
}
if (other.getUseFilteredFirstSolutionStrategy() != false) {
setUseFilteredFirstSolutionStrategy(other.getUseFilteredFirstSolutionStrategy());
}
if (other.getSavingsNeighborsRatio() != 0D) {
setSavingsNeighborsRatio(other.getSavingsNeighborsRatio());
}
if (other.getSavingsAddReverseArcs() != false) {
setSavingsAddReverseArcs(other.getSavingsAddReverseArcs());
}
if (other.hasLocalSearchOperators()) {
mergeLocalSearchOperators(other.getLocalSearchOperators());
}
if (other.localSearchMetaheuristic_ != 0) {
setLocalSearchMetaheuristicValue(other.getLocalSearchMetaheuristicValue());
}
if (other.getGuidedLocalSearchLambdaCoefficient() != 0D) {
setGuidedLocalSearchLambdaCoefficient(other.getGuidedLocalSearchLambdaCoefficient());
}
if (other.getUseDepthFirstSearch() != false) {
setUseDepthFirstSearch(other.getUseDepthFirstSearch());
}
if (other.getOptimizationStep() != 0L) {
setOptimizationStep(other.getOptimizationStep());
}
if (other.getSolutionLimit() != 0L) {
setSolutionLimit(other.getSolutionLimit());
}
if (other.getTimeLimitMs() != 0L) {
setTimeLimitMs(other.getTimeLimitMs());
}
if (other.getLnsTimeLimitMs() != 0L) {
setLnsTimeLimitMs(other.getLnsTimeLimitMs());
}
if (other.getUseLightPropagation() != false) {
setUseLightPropagation(other.getUseLightPropagation());
}
if (other.getFingerprintArcCostEvaluators() != false) {
setFingerprintArcCostEvaluators(other.getFingerprintArcCostEvaluators());
}
if (other.getLogSearch() != false) {
setLogSearch(other.getLogSearch());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.ortools.constraintsolver.RoutingSearchParameters parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.ortools.constraintsolver.RoutingSearchParameters) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int firstSolutionStrategy_ = 0;
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
*/
public int getFirstSolutionStrategyValue() {
return firstSolutionStrategy_;
}
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
*/
public Builder setFirstSolutionStrategyValue(int value) {
firstSolutionStrategy_ = value;
onChanged();
return this;
}
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
*/
public com.google.ortools.constraintsolver.FirstSolutionStrategy.Value getFirstSolutionStrategy() {
com.google.ortools.constraintsolver.FirstSolutionStrategy.Value result = com.google.ortools.constraintsolver.FirstSolutionStrategy.Value.valueOf(firstSolutionStrategy_);
return result == null ? com.google.ortools.constraintsolver.FirstSolutionStrategy.Value.UNRECOGNIZED : result;
}
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
*/
public Builder setFirstSolutionStrategy(com.google.ortools.constraintsolver.FirstSolutionStrategy.Value value) {
if (value == null) {
throw new NullPointerException();
}
firstSolutionStrategy_ = value.getNumber();
onChanged();
return this;
}
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
*/
public Builder clearFirstSolutionStrategy() {
firstSolutionStrategy_ = 0;
onChanged();
return this;
}
private boolean useFilteredFirstSolutionStrategy_ ;
/**
*
* These are advanced first solutions strategy settings which should not be
* modified unless you know what you are doing.
* Use filtered version of first solution strategy if available.
*
*
* bool use_filtered_first_solution_strategy = 2;
*/
public boolean getUseFilteredFirstSolutionStrategy() {
return useFilteredFirstSolutionStrategy_;
}
/**
*
* These are advanced first solutions strategy settings which should not be
* modified unless you know what you are doing.
* Use filtered version of first solution strategy if available.
*
*
* bool use_filtered_first_solution_strategy = 2;
*/
public Builder setUseFilteredFirstSolutionStrategy(boolean value) {
useFilteredFirstSolutionStrategy_ = value;
onChanged();
return this;
}
/**
*
* These are advanced first solutions strategy settings which should not be
* modified unless you know what you are doing.
* Use filtered version of first solution strategy if available.
*
*
* bool use_filtered_first_solution_strategy = 2;
*/
public Builder clearUseFilteredFirstSolutionStrategy() {
useFilteredFirstSolutionStrategy_ = false;
onChanged();
return this;
}
private double savingsNeighborsRatio_ ;
/**
*
* Parameters specific to the Savings first solution heuristic.
* Ratio (between 0 and 1) of neighbors to consider for each node when
* constructing the savings. If <= 0 or greater than 1, its value is
* considered to be 1.0.
*
*
* double savings_neighbors_ratio = 14;
*/
public double getSavingsNeighborsRatio() {
return savingsNeighborsRatio_;
}
/**
*
* Parameters specific to the Savings first solution heuristic.
* Ratio (between 0 and 1) of neighbors to consider for each node when
* constructing the savings. If <= 0 or greater than 1, its value is
* considered to be 1.0.
*
*
* double savings_neighbors_ratio = 14;
*/
public Builder setSavingsNeighborsRatio(double value) {
savingsNeighborsRatio_ = value;
onChanged();
return this;
}
/**
*
* Parameters specific to the Savings first solution heuristic.
* Ratio (between 0 and 1) of neighbors to consider for each node when
* constructing the savings. If <= 0 or greater than 1, its value is
* considered to be 1.0.
*
*
* double savings_neighbors_ratio = 14;
*/
public Builder clearSavingsNeighborsRatio() {
savingsNeighborsRatio_ = 0D;
onChanged();
return this;
}
private boolean savingsAddReverseArcs_ ;
/**
*
* Add savings related to reverse arcs when finding the nearest neighbors
* of the nodes.
*
*
* bool savings_add_reverse_arcs = 15;
*/
public boolean getSavingsAddReverseArcs() {
return savingsAddReverseArcs_;
}
/**
*
* Add savings related to reverse arcs when finding the nearest neighbors
* of the nodes.
*
*
* bool savings_add_reverse_arcs = 15;
*/
public Builder setSavingsAddReverseArcs(boolean value) {
savingsAddReverseArcs_ = value;
onChanged();
return this;
}
/**
*
* Add savings related to reverse arcs when finding the nearest neighbors
* of the nodes.
*
*
* bool savings_add_reverse_arcs = 15;
*/
public Builder clearSavingsAddReverseArcs() {
savingsAddReverseArcs_ = false;
onChanged();
return this;
}
private com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators localSearchOperators_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperatorsOrBuilder> localSearchOperatorsBuilder_;
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public boolean hasLocalSearchOperators() {
return localSearchOperatorsBuilder_ != null || localSearchOperators_ != null;
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators getLocalSearchOperators() {
if (localSearchOperatorsBuilder_ == null) {
return localSearchOperators_ == null ? com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.getDefaultInstance() : localSearchOperators_;
} else {
return localSearchOperatorsBuilder_.getMessage();
}
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public Builder setLocalSearchOperators(com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators value) {
if (localSearchOperatorsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
localSearchOperators_ = value;
onChanged();
} else {
localSearchOperatorsBuilder_.setMessage(value);
}
return this;
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public Builder setLocalSearchOperators(
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder builderForValue) {
if (localSearchOperatorsBuilder_ == null) {
localSearchOperators_ = builderForValue.build();
onChanged();
} else {
localSearchOperatorsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public Builder mergeLocalSearchOperators(com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators value) {
if (localSearchOperatorsBuilder_ == null) {
if (localSearchOperators_ != null) {
localSearchOperators_ =
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.newBuilder(localSearchOperators_).mergeFrom(value).buildPartial();
} else {
localSearchOperators_ = value;
}
onChanged();
} else {
localSearchOperatorsBuilder_.mergeFrom(value);
}
return this;
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public Builder clearLocalSearchOperators() {
if (localSearchOperatorsBuilder_ == null) {
localSearchOperators_ = null;
onChanged();
} else {
localSearchOperators_ = null;
localSearchOperatorsBuilder_ = null;
}
return this;
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder getLocalSearchOperatorsBuilder() {
onChanged();
return getLocalSearchOperatorsFieldBuilder().getBuilder();
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperatorsOrBuilder getLocalSearchOperatorsOrBuilder() {
if (localSearchOperatorsBuilder_ != null) {
return localSearchOperatorsBuilder_.getMessageOrBuilder();
} else {
return localSearchOperators_ == null ?
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.getDefaultInstance() : localSearchOperators_;
}
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperatorsOrBuilder>
getLocalSearchOperatorsFieldBuilder() {
if (localSearchOperatorsBuilder_ == null) {
localSearchOperatorsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperatorsOrBuilder>(
getLocalSearchOperators(),
getParentForChildren(),
isClean());
localSearchOperators_ = null;
}
return localSearchOperatorsBuilder_;
}
private int localSearchMetaheuristic_ = 0;
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
*/
public int getLocalSearchMetaheuristicValue() {
return localSearchMetaheuristic_;
}
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
*/
public Builder setLocalSearchMetaheuristicValue(int value) {
localSearchMetaheuristic_ = value;
onChanged();
return this;
}
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
*/
public com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value getLocalSearchMetaheuristic() {
com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value result = com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value.valueOf(localSearchMetaheuristic_);
return result == null ? com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value.UNRECOGNIZED : result;
}
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
*/
public Builder setLocalSearchMetaheuristic(com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value value) {
if (value == null) {
throw new NullPointerException();
}
localSearchMetaheuristic_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
*/
public Builder clearLocalSearchMetaheuristic() {
localSearchMetaheuristic_ = 0;
onChanged();
return this;
}
private double guidedLocalSearchLambdaCoefficient_ ;
/**
*
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Lambda coefficient used to penalize arc costs when GUIDED_LOCAL_SEARCH is
* used.
*
*
* double guided_local_search_lambda_coefficient = 5;
*/
public double getGuidedLocalSearchLambdaCoefficient() {
return guidedLocalSearchLambdaCoefficient_;
}
/**
*
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Lambda coefficient used to penalize arc costs when GUIDED_LOCAL_SEARCH is
* used.
*
*
* double guided_local_search_lambda_coefficient = 5;
*/
public Builder setGuidedLocalSearchLambdaCoefficient(double value) {
guidedLocalSearchLambdaCoefficient_ = value;
onChanged();
return this;
}
/**
*
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Lambda coefficient used to penalize arc costs when GUIDED_LOCAL_SEARCH is
* used.
*
*
* double guided_local_search_lambda_coefficient = 5;
*/
public Builder clearGuidedLocalSearchLambdaCoefficient() {
guidedLocalSearchLambdaCoefficient_ = 0D;
onChanged();
return this;
}
private boolean useDepthFirstSearch_ ;
/**
*
* --- Search control ---
* If true, the solver should use depth-first search rather than local search
* to solve the problem.
*
*
* bool use_depth_first_search = 6;
*/
public boolean getUseDepthFirstSearch() {
return useDepthFirstSearch_;
}
/**
*
* --- Search control ---
* If true, the solver should use depth-first search rather than local search
* to solve the problem.
*
*
* bool use_depth_first_search = 6;
*/
public Builder setUseDepthFirstSearch(boolean value) {
useDepthFirstSearch_ = value;
onChanged();
return this;
}
/**
*
* --- Search control ---
* If true, the solver should use depth-first search rather than local search
* to solve the problem.
*
*
* bool use_depth_first_search = 6;
*/
public Builder clearUseDepthFirstSearch() {
useDepthFirstSearch_ = false;
onChanged();
return this;
}
private long optimizationStep_ ;
/**
*
* Minimum step by which the solution must be improved in local search.
*
*
* int64 optimization_step = 7;
*/
public long getOptimizationStep() {
return optimizationStep_;
}
/**
*
* Minimum step by which the solution must be improved in local search.
*
*
* int64 optimization_step = 7;
*/
public Builder setOptimizationStep(long value) {
optimizationStep_ = value;
onChanged();
return this;
}
/**
*
* Minimum step by which the solution must be improved in local search.
*
*
* int64 optimization_step = 7;
*/
public Builder clearOptimizationStep() {
optimizationStep_ = 0L;
onChanged();
return this;
}
private long solutionLimit_ ;
/**
*
* -- Search limits --
* Limit to the number of solutions generated during the search.
*
*
* int64 solution_limit = 8;
*/
public long getSolutionLimit() {
return solutionLimit_;
}
/**
*
* -- Search limits --
* Limit to the number of solutions generated during the search.
*
*
* int64 solution_limit = 8;
*/
public Builder setSolutionLimit(long value) {
solutionLimit_ = value;
onChanged();
return this;
}
/**
*
* -- Search limits --
* Limit to the number of solutions generated during the search.
*
*
* int64 solution_limit = 8;
*/
public Builder clearSolutionLimit() {
solutionLimit_ = 0L;
onChanged();
return this;
}
private long timeLimitMs_ ;
/**
*
* Limit in milliseconds to the time spent in the search.
*
*
* int64 time_limit_ms = 9;
*/
public long getTimeLimitMs() {
return timeLimitMs_;
}
/**
*
* Limit in milliseconds to the time spent in the search.
*
*
* int64 time_limit_ms = 9;
*/
public Builder setTimeLimitMs(long value) {
timeLimitMs_ = value;
onChanged();
return this;
}
/**
*
* Limit in milliseconds to the time spent in the search.
*
*
* int64 time_limit_ms = 9;
*/
public Builder clearTimeLimitMs() {
timeLimitMs_ = 0L;
onChanged();
return this;
}
private long lnsTimeLimitMs_ ;
/**
*
* Limit in milliseconds to the time spent in the completion search for each
* local search neighbor.
*
*
* int64 lns_time_limit_ms = 10;
*/
public long getLnsTimeLimitMs() {
return lnsTimeLimitMs_;
}
/**
*
* Limit in milliseconds to the time spent in the completion search for each
* local search neighbor.
*
*
* int64 lns_time_limit_ms = 10;
*/
public Builder setLnsTimeLimitMs(long value) {
lnsTimeLimitMs_ = value;
onChanged();
return this;
}
/**
*
* Limit in milliseconds to the time spent in the completion search for each
* local search neighbor.
*
*
* int64 lns_time_limit_ms = 10;
*/
public Builder clearLnsTimeLimitMs() {
lnsTimeLimitMs_ = 0L;
onChanged();
return this;
}
private boolean useLightPropagation_ ;
/**
*
* --- Propagation control ---
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Use constraints with light propagation in routing model. Extra propagation
* is only necessary when using depth-first search or for models which
* require strong propagation to finalize the value of secondary variables.
* Changing this setting to true will slow down the search in most cases and
* increase memory consumption in all cases.
*
*
* bool use_light_propagation = 11;
*/
public boolean getUseLightPropagation() {
return useLightPropagation_;
}
/**
*
* --- Propagation control ---
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Use constraints with light propagation in routing model. Extra propagation
* is only necessary when using depth-first search or for models which
* require strong propagation to finalize the value of secondary variables.
* Changing this setting to true will slow down the search in most cases and
* increase memory consumption in all cases.
*
*
* bool use_light_propagation = 11;
*/
public Builder setUseLightPropagation(boolean value) {
useLightPropagation_ = value;
onChanged();
return this;
}
/**
*
* --- Propagation control ---
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Use constraints with light propagation in routing model. Extra propagation
* is only necessary when using depth-first search or for models which
* require strong propagation to finalize the value of secondary variables.
* Changing this setting to true will slow down the search in most cases and
* increase memory consumption in all cases.
*
*
* bool use_light_propagation = 11;
*/
public Builder clearUseLightPropagation() {
useLightPropagation_ = false;
onChanged();
return this;
}
private boolean fingerprintArcCostEvaluators_ ;
/**
*
* --- Miscellaneous ---
* Some of these are advanced settings which should not be modified unless you
* know what you are doing.
* If true, arc cost evaluators will be fingerprinted. The fingerprint will
* be used when computing vehicle cost equivalent classes, otherwise the
* address of the evaluator will be used.
*
*
* bool fingerprint_arc_cost_evaluators = 12;
*/
public boolean getFingerprintArcCostEvaluators() {
return fingerprintArcCostEvaluators_;
}
/**
*
* --- Miscellaneous ---
* Some of these are advanced settings which should not be modified unless you
* know what you are doing.
* If true, arc cost evaluators will be fingerprinted. The fingerprint will
* be used when computing vehicle cost equivalent classes, otherwise the
* address of the evaluator will be used.
*
*
* bool fingerprint_arc_cost_evaluators = 12;
*/
public Builder setFingerprintArcCostEvaluators(boolean value) {
fingerprintArcCostEvaluators_ = value;
onChanged();
return this;
}
/**
*
* --- Miscellaneous ---
* Some of these are advanced settings which should not be modified unless you
* know what you are doing.
* If true, arc cost evaluators will be fingerprinted. The fingerprint will
* be used when computing vehicle cost equivalent classes, otherwise the
* address of the evaluator will be used.
*
*
* bool fingerprint_arc_cost_evaluators = 12;
*/
public Builder clearFingerprintArcCostEvaluators() {
fingerprintArcCostEvaluators_ = false;
onChanged();
return this;
}
private boolean logSearch_ ;
/**
*
* Activates search logging. For each solution found during the search, the
* following will be displayed: its objective value, the maximum objective
* value since the beginning of the search, the elapsed time since the
* beginning of the search, the number of branches explored in the search
* tree, the number of failures in the search tree, the depth of the search
* tree, the number of local search neighbors explored, the number of local
* search neighbors filtered by local search filters, the number of local
* search neighbors accepted, the total memory used and the percentage of the
* search done.
*
*
* bool log_search = 13;
*/
public boolean getLogSearch() {
return logSearch_;
}
/**
*
* Activates search logging. For each solution found during the search, the
* following will be displayed: its objective value, the maximum objective
* value since the beginning of the search, the elapsed time since the
* beginning of the search, the number of branches explored in the search
* tree, the number of failures in the search tree, the depth of the search
* tree, the number of local search neighbors explored, the number of local
* search neighbors filtered by local search filters, the number of local
* search neighbors accepted, the total memory used and the percentage of the
* search done.
*
*
* bool log_search = 13;
*/
public Builder setLogSearch(boolean value) {
logSearch_ = value;
onChanged();
return this;
}
/**
*
* Activates search logging. For each solution found during the search, the
* following will be displayed: its objective value, the maximum objective
* value since the beginning of the search, the elapsed time since the
* beginning of the search, the number of branches explored in the search
* tree, the number of failures in the search tree, the depth of the search
* tree, the number of local search neighbors explored, the number of local
* search neighbors filtered by local search filters, the number of local
* search neighbors accepted, the total memory used and the percentage of the
* search done.
*
*
* bool log_search = 13;
*/
public Builder clearLogSearch() {
logSearch_ = false;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:operations_research.RoutingSearchParameters)
}
// @@protoc_insertion_point(class_scope:operations_research.RoutingSearchParameters)
private static final com.google.ortools.constraintsolver.RoutingSearchParameters DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ortools.constraintsolver.RoutingSearchParameters();
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RoutingSearchParameters parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RoutingSearchParameters(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.google.ortools.constraintsolver.RoutingSearchParameters getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy