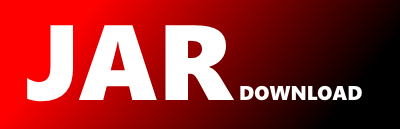
org.usf.inspect.naming.DirContextTracker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of inspect-core Show documentation
Show all versions of inspect-core Show documentation
INtegrated System Performance Evaluation and Communication Tracking core libray
package org.usf.inspect.naming;
import static java.net.URI.create;
import static java.util.Objects.nonNull;
import static org.usf.inspect.core.ExceptionInfo.mainCauseException;
import static org.usf.inspect.core.Helper.threadName;
import static org.usf.inspect.core.Session.appendSessionStage;
import static org.usf.inspect.core.StageTracker.call;
import static org.usf.inspect.core.StageTracker.exec;
import static org.usf.inspect.naming.NamingAction.ATTRIB;
import static org.usf.inspect.naming.NamingAction.CONNECTION;
import static org.usf.inspect.naming.NamingAction.DISCONNECTION;
import static org.usf.inspect.naming.NamingAction.LIST;
import static org.usf.inspect.naming.NamingAction.LOOKUP;
import static org.usf.inspect.naming.NamingAction.SEARCH;
import java.util.ArrayList;
import javax.naming.Name;
import javax.naming.NameClassPair;
import javax.naming.NamingEnumeration;
import javax.naming.NamingException;
import javax.naming.directory.Attributes;
import javax.naming.directory.DirContext;
import javax.naming.directory.SearchControls;
import javax.naming.directory.SearchResult;
import org.usf.inspect.core.NamingRequest;
import org.usf.inspect.core.NamingRequestStage;
import org.usf.inspect.core.SafeCallable;
import org.usf.inspect.core.StageTracker.StageConsumer;
import lombok.RequiredArgsConstructor;
import lombok.experimental.Delegate;
/**
*
* @author u$f
*
*/
@RequiredArgsConstructor
public class DirContextTracker implements DirContext {
@Delegate
private DirContext ctx;
private NamingRequest req;
DirContextTracker connection(SafeCallable supplier) throws T {
ctx = call(supplier, (s,e,c,t)-> {
req = new NamingRequest();
req.setStart(s);
if(nonNull(t)) {
req.setEnd(e);
}
var url = create(c.getEnvironment().get(PROVIDER_URL).toString());
req.setProtocol(url.getScheme());
req.setHost(url.getHost());
req.setPort(url.getPort());
req.setUser(c.getEnvironment().get(SECURITY_PRINCIPAL).toString());
req.setThreadName(threadName());
req.setActions(new ArrayList<>());
appendAction(CONNECTION).accept(s, e, c, t);
appendSessionStage(req);
});
return this;
}
@Override
public Object lookup(Name name) throws NamingException {
return call(()-> ctx.lookup(name), appendAction(LOOKUP, name.toString()));
}
@Override
public Object lookup(String name) throws NamingException {
return call(()-> ctx.lookup(name), appendAction(LOOKUP, name));
}
@Override
public NamingEnumeration list(Name name) throws NamingException {
return call(()-> ctx.list(name), appendAction(LIST, name.toString()));
}
@Override
public NamingEnumeration list(String name) throws NamingException {
return call(()-> ctx.list(name), appendAction(LIST, name));
}
@Override
public Attributes getAttributes(Name name) throws NamingException {
return call(()-> ctx.getAttributes(name), appendAction(ATTRIB, name.toString()));
}
@Override
public Attributes getAttributes(String name) throws NamingException {
return call(()-> ctx.getAttributes(name), appendAction(ATTRIB, name));
}
@Override
public Attributes getAttributes(Name name, String[] attrIds) throws NamingException {
return call(()-> ctx.getAttributes(name, attrIds), appendAction(ATTRIB, name.toString()));
}
@Override
public Attributes getAttributes(String name, String[] attrIds) throws NamingException {
return call(()-> ctx.getAttributes(name, attrIds), appendAction(ATTRIB, name));
}
@Override
public NamingEnumeration search(Name name, Attributes matchingAttributes, String[] attributesToReturn) throws NamingException {
return call(()-> ctx.search(name, matchingAttributes, attributesToReturn), appendAction(SEARCH, name.toString()));
}
@Override
public NamingEnumeration search(String name, Attributes matchingAttributes, String[] attributesToReturn) throws NamingException {
return call(()-> ctx.search(name, matchingAttributes, attributesToReturn), appendAction(SEARCH, name));
}
@Override
public NamingEnumeration search(Name name, Attributes matchingAttributes) throws NamingException {
return call(()-> ctx.search(name, matchingAttributes), appendAction(SEARCH, name.toString()));
}
@Override
public NamingEnumeration search(String name, Attributes matchingAttributes) throws NamingException {
return call(()-> ctx.search(name, matchingAttributes), appendAction(SEARCH, name));
}
@Override
public NamingEnumeration search(Name name, String filter, SearchControls cons) throws NamingException {
return call(()-> ctx.search(name, filter, cons), appendAction(SEARCH, name.toString()));
}
@Override
public NamingEnumeration search(String name, String filter, SearchControls cons) throws NamingException {
return call(()-> ctx.search(name, filter, cons), appendAction(SEARCH, name));
}
@Override
public NamingEnumeration search(Name name, String filterExpr, Object[] filterArgs, SearchControls cons) throws NamingException {
return call(()-> ctx.search(name, filterExpr, cons), appendAction(SEARCH, name.toString()));
}
@Override
public NamingEnumeration search(String name, String filterExpr, Object[] filterArgs, SearchControls cons) throws NamingException {
return call(()-> ctx.search(name, filterExpr, cons), appendAction(SEARCH, name));
}
@Override
public void close() throws NamingException {
exec(ctx::close, (s,e,v,t)->{
appendAction(DISCONNECTION).accept(s, e, v, t);
req.setEnd(e);
});
}
StageConsumer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy