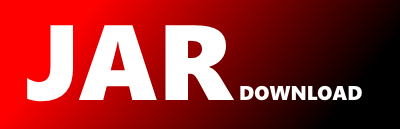
com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BQPaymentsServiceGrpc Maven / Gradle / Ivy
The newest version!
package com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.42.1)",
comments = "Source: v10/api/bq_payments_service.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class BQPaymentsServiceGrpc {
private BQPaymentsServiceGrpc() {}
public static final String SERVICE_NAME = "com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BQPaymentsService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getExchangePaymentsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExchangePayments",
requestType = com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.ExchangePaymentsRequest.class,
responseType = com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getExchangePaymentsMethod() {
io.grpc.MethodDescriptor getExchangePaymentsMethod;
if ((getExchangePaymentsMethod = BQPaymentsServiceGrpc.getExchangePaymentsMethod) == null) {
synchronized (BQPaymentsServiceGrpc.class) {
if ((getExchangePaymentsMethod = BQPaymentsServiceGrpc.getExchangePaymentsMethod) == null) {
BQPaymentsServiceGrpc.getExchangePaymentsMethod = getExchangePaymentsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExchangePayments"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.ExchangePaymentsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments.getDefaultInstance()))
.setSchemaDescriptor(new BQPaymentsServiceMethodDescriptorSupplier("ExchangePayments"))
.build();
}
}
}
return getExchangePaymentsMethod;
}
private static volatile io.grpc.MethodDescriptor getInitiatePaymentsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "InitiatePayments",
requestType = com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.InitiatePaymentsRequest.class,
responseType = com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getInitiatePaymentsMethod() {
io.grpc.MethodDescriptor getInitiatePaymentsMethod;
if ((getInitiatePaymentsMethod = BQPaymentsServiceGrpc.getInitiatePaymentsMethod) == null) {
synchronized (BQPaymentsServiceGrpc.class) {
if ((getInitiatePaymentsMethod = BQPaymentsServiceGrpc.getInitiatePaymentsMethod) == null) {
BQPaymentsServiceGrpc.getInitiatePaymentsMethod = getInitiatePaymentsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "InitiatePayments"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.InitiatePaymentsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments.getDefaultInstance()))
.setSchemaDescriptor(new BQPaymentsServiceMethodDescriptorSupplier("InitiatePayments"))
.build();
}
}
}
return getInitiatePaymentsMethod;
}
private static volatile io.grpc.MethodDescriptor getRetrievePaymentsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "RetrievePayments",
requestType = com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.RetrievePaymentsRequest.class,
responseType = com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getRetrievePaymentsMethod() {
io.grpc.MethodDescriptor getRetrievePaymentsMethod;
if ((getRetrievePaymentsMethod = BQPaymentsServiceGrpc.getRetrievePaymentsMethod) == null) {
synchronized (BQPaymentsServiceGrpc.class) {
if ((getRetrievePaymentsMethod = BQPaymentsServiceGrpc.getRetrievePaymentsMethod) == null) {
BQPaymentsServiceGrpc.getRetrievePaymentsMethod = getRetrievePaymentsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "RetrievePayments"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.RetrievePaymentsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments.getDefaultInstance()))
.setSchemaDescriptor(new BQPaymentsServiceMethodDescriptorSupplier("RetrievePayments"))
.build();
}
}
}
return getRetrievePaymentsMethod;
}
private static volatile io.grpc.MethodDescriptor getUpdatePaymentsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "UpdatePayments",
requestType = com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.UpdatePaymentsRequest.class,
responseType = com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getUpdatePaymentsMethod() {
io.grpc.MethodDescriptor getUpdatePaymentsMethod;
if ((getUpdatePaymentsMethod = BQPaymentsServiceGrpc.getUpdatePaymentsMethod) == null) {
synchronized (BQPaymentsServiceGrpc.class) {
if ((getUpdatePaymentsMethod = BQPaymentsServiceGrpc.getUpdatePaymentsMethod) == null) {
BQPaymentsServiceGrpc.getUpdatePaymentsMethod = getUpdatePaymentsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "UpdatePayments"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.UpdatePaymentsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments.getDefaultInstance()))
.setSchemaDescriptor(new BQPaymentsServiceMethodDescriptorSupplier("UpdatePayments"))
.build();
}
}
}
return getUpdatePaymentsMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static BQPaymentsServiceStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public BQPaymentsServiceStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new BQPaymentsServiceStub(channel, callOptions);
}
};
return BQPaymentsServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static BQPaymentsServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public BQPaymentsServiceBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new BQPaymentsServiceBlockingStub(channel, callOptions);
}
};
return BQPaymentsServiceBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static BQPaymentsServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public BQPaymentsServiceFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new BQPaymentsServiceFutureStub(channel, callOptions);
}
};
return BQPaymentsServiceFutureStub.newStub(factory, channel);
}
/**
*/
public static abstract class BQPaymentsServiceImplBase implements io.grpc.BindableService {
/**
*/
public void exchangePayments(com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.ExchangePaymentsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExchangePaymentsMethod(), responseObserver);
}
/**
*/
public void initiatePayments(com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.InitiatePaymentsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getInitiatePaymentsMethod(), responseObserver);
}
/**
*/
public void retrievePayments(com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.RetrievePaymentsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getRetrievePaymentsMethod(), responseObserver);
}
/**
*/
public void updatePayments(com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.UpdatePaymentsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getUpdatePaymentsMethod(), responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getExchangePaymentsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.ExchangePaymentsRequest,
com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments>(
this, METHODID_EXCHANGE_PAYMENTS)))
.addMethod(
getInitiatePaymentsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.InitiatePaymentsRequest,
com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments>(
this, METHODID_INITIATE_PAYMENTS)))
.addMethod(
getRetrievePaymentsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.RetrievePaymentsRequest,
com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments>(
this, METHODID_RETRIEVE_PAYMENTS)))
.addMethod(
getUpdatePaymentsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.UpdatePaymentsRequest,
com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments>(
this, METHODID_UPDATE_PAYMENTS)))
.build();
}
}
/**
*/
public static final class BQPaymentsServiceStub extends io.grpc.stub.AbstractAsyncStub {
private BQPaymentsServiceStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected BQPaymentsServiceStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new BQPaymentsServiceStub(channel, callOptions);
}
/**
*/
public void exchangePayments(com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.ExchangePaymentsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getExchangePaymentsMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void initiatePayments(com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.InitiatePaymentsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getInitiatePaymentsMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void retrievePayments(com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.RetrievePaymentsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getRetrievePaymentsMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void updatePayments(com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.UpdatePaymentsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getUpdatePaymentsMethod(), getCallOptions()), request, responseObserver);
}
}
/**
*/
public static final class BQPaymentsServiceBlockingStub extends io.grpc.stub.AbstractBlockingStub {
private BQPaymentsServiceBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected BQPaymentsServiceBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new BQPaymentsServiceBlockingStub(channel, callOptions);
}
/**
*/
public com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments exchangePayments(com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.ExchangePaymentsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getExchangePaymentsMethod(), getCallOptions(), request);
}
/**
*/
public com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments initiatePayments(com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.InitiatePaymentsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getInitiatePaymentsMethod(), getCallOptions(), request);
}
/**
*/
public com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments retrievePayments(com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.RetrievePaymentsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getRetrievePaymentsMethod(), getCallOptions(), request);
}
/**
*/
public com.redhat.mercury.merchandisingloan.v10.PaymentsOuterClass.Payments updatePayments(com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.UpdatePaymentsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getUpdatePaymentsMethod(), getCallOptions(), request);
}
}
/**
*/
public static final class BQPaymentsServiceFutureStub extends io.grpc.stub.AbstractFutureStub {
private BQPaymentsServiceFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected BQPaymentsServiceFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new BQPaymentsServiceFutureStub(channel, callOptions);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture exchangePayments(
com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.ExchangePaymentsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getExchangePaymentsMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture initiatePayments(
com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.InitiatePaymentsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getInitiatePaymentsMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture retrievePayments(
com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.RetrievePaymentsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getRetrievePaymentsMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture updatePayments(
com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.UpdatePaymentsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getUpdatePaymentsMethod(), getCallOptions()), request);
}
}
private static final int METHODID_EXCHANGE_PAYMENTS = 0;
private static final int METHODID_INITIATE_PAYMENTS = 1;
private static final int METHODID_RETRIEVE_PAYMENTS = 2;
private static final int METHODID_UPDATE_PAYMENTS = 3;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final BQPaymentsServiceImplBase serviceImpl;
private final int methodId;
MethodHandlers(BQPaymentsServiceImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_EXCHANGE_PAYMENTS:
serviceImpl.exchangePayments((com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.ExchangePaymentsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_INITIATE_PAYMENTS:
serviceImpl.initiatePayments((com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.InitiatePaymentsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_RETRIEVE_PAYMENTS:
serviceImpl.retrievePayments((com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.RetrievePaymentsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_UPDATE_PAYMENTS:
serviceImpl.updatePayments((com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.UpdatePaymentsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
private static abstract class BQPaymentsServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
BQPaymentsServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.redhat.mercury.merchandisingloan.v10.api.bqpaymentsservice.BqPaymentsService.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("BQPaymentsService");
}
}
private static final class BQPaymentsServiceFileDescriptorSupplier
extends BQPaymentsServiceBaseDescriptorSupplier {
BQPaymentsServiceFileDescriptorSupplier() {}
}
private static final class BQPaymentsServiceMethodDescriptorSupplier
extends BQPaymentsServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
BQPaymentsServiceMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (BQPaymentsServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new BQPaymentsServiceFileDescriptorSupplier())
.addMethod(getExchangePaymentsMethod())
.addMethod(getInitiatePaymentsMethod())
.addMethod(getRetrievePaymentsMethod())
.addMethod(getUpdatePaymentsMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy