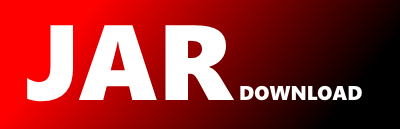
com.querydsl.core.group.GroupBy Maven / Gradle / Ivy
/*
* Copyright 2015, The Querydsl Team (http://www.querydsl.com/team)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.querydsl.core.group;
import com.mysema.commons.lang.Pair;
import com.querydsl.core.ResultTransformer;
import com.querydsl.core.types.Expression;
import com.querydsl.core.types.Projections;
import java.math.MathContext;
import java.util.Comparator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.SortedMap;
import java.util.SortedSet;
/**
* {@code GroupBy} provides factory methods for {@link ResultTransformer} and {@link
* GroupExpression} creation
*
* Example: Group employees by department
*
*
{@code
* query.transform(
* GroupBy.groupBy(employee.department)
* .as(GroupBy.list(Projections.tuple(employee.id, employee.firstName, employee.lastName))))
* }
*
* @author sasa
* @author tiwe
*/
public class GroupBy {
/**
* Create a new GroupByBuilder for the given key expression
*
* @param key key for aggregation
* @return builder for further specification
*/
public static GroupByBuilder groupBy(Expression key) {
return new GroupByBuilder<>(key);
}
/**
* Create a new GroupByBuilder for the given key expressions
*
* @param keys keys for aggregation
* @return builder for further specification
*/
public static GroupByBuilder> groupBy(Expression>... keys) {
return new GroupByBuilder<>(Projections.list(keys));
}
/**
* Create a new aggregating min expression
*
* @param expression expression for which the minimum value will be used in the group by
* projection
* @return wrapper expression
*/
public static > AbstractGroupExpression min(
Expression expression) {
return new GMin<>(expression);
}
/**
* Create a new aggregating sum expression
*
* @param expression expression a for which the accumulated sum will be used in the group by
* projection
* @return wrapper expression
*/
public static AbstractGroupExpression sum(Expression expression) {
return new GSum<>(expression);
}
/**
* Create a new aggregating avg expression, uses default MathContext.DECIMAL128 for average
* calculation
*
* @param expression expression for which the accumulated average value will be used in the group
* by projection
* @return wrapper expression
*/
public static AbstractGroupExpression avg(Expression expression) {
return new GAvg<>(expression);
}
/**
* Create a new aggregating avg expression with a user-provided MathContext
*
* @param expression expression for which the accumulated average value will be used in the group
* by projection
* @param mathContext mathContext for average calculation
* @return wrapper expression
*/
public static AbstractGroupExpression avg(
Expression expression, MathContext mathContext) {
return new GAvg<>(expression, mathContext);
}
/**
* Create a new aggregating max expression
*
* @param expression expression for which the maximum value will be used in the group by
* projection
* @return wrapper expression
*/
public static > AbstractGroupExpression max(
Expression expression) {
return new GMax<>(expression);
}
/**
* Create a new aggregating list expression
*
* @param expression values for this expression will be accumulated into a list
* @return wrapper expression
*/
public static AbstractGroupExpression> list(Expression expression) {
return new GList<>(expression);
}
/**
* Create a new aggregating list expression
*
* @param groupExpression values for this expression will be accumulated into a list
* @param
* @param
* @return wrapper expression
*/
public static AbstractGroupExpression> list(
GroupExpression groupExpression) {
return new MixinGroupExpression<>(groupExpression, new GList<>(groupExpression));
}
/**
* Create a new aggregating set expression using a backing LinkedHashSet
*
* @param expression values for this expression will be accumulated into a set
* @return wrapper expression
*/
public static AbstractGroupExpression> set(Expression expression) {
return GSet.createLinked(expression);
}
/**
* Create a new aggregating set expression using a backing LinkedHashSet
*
* @param groupExpression values for this expression will be accumulated into a set
* @param
* @param
* @return wrapper expression
*/
public static GroupExpression> set(GroupExpression groupExpression) {
return new MixinGroupExpression<>(groupExpression, GSet.createLinked(groupExpression));
}
/**
* Create a new aggregating set expression using a backing TreeSet
*
* @param expression values for this expression will be accumulated into a set
* @return wrapper expression
*/
public static >
AbstractGroupExpression> sortedSet(Expression expression) {
return GSet.createSorted(expression);
}
/**
* Create a new aggregating set expression using a backing TreeSet
*
* @param groupExpression values for this expression will be accumulated into a set
* @return wrapper expression
*/
public static > GroupExpression> sortedSet(
GroupExpression groupExpression) {
return new MixinGroupExpression<>(groupExpression, GSet.createSorted(groupExpression));
}
/**
* Create a new aggregating set expression using a backing TreeSet using the given comparator
*
* @param expression values for this expression will be accumulated into a set
* @param comparator comparator of the created TreeSet instance
* @return wrapper expression
*/
public static AbstractGroupExpression> sortedSet(
Expression expression, Comparator super E> comparator) {
return GSet.createSorted(expression, comparator);
}
/**
* Create a new aggregating set expression using a backing TreeSet using the given comparator
*
* @param groupExpression values for this expression will be accumulated into a set
* @param comparator comparator of the created TreeSet instance
* @return wrapper expression
*/
public static GroupExpression> sortedSet(
GroupExpression groupExpression, Comparator super F> comparator) {
return new MixinGroupExpression<>(
groupExpression, GSet.createSorted(groupExpression, comparator));
}
/**
* Create a new aggregating map expression using a backing LinkedHashMap
*
* @param key key for the map entries
* @param value value for the map entries
* @return wrapper expression
*/
public static AbstractGroupExpression, Map> map(
Expression key, Expression value) {
return GMap.createLinked(QPair.create(key, value));
}
/**
* Create a new aggregating map expression using a backing LinkedHashMap
*
* @param key key for the map entries
* @param value value for the map entries
* @return wrapper expression
*/
public static AbstractGroupExpression, Map> map(
GroupExpression key, Expression value) {
return map(key, new GOne<>(value));
}
/**
* Create a new aggregating map expression using a backing LinkedHashMap
*
* @param key key for the map entries
* @param value value for the map entries
* @return wrapper expression
*/
public static AbstractGroupExpression, Map> map(
Expression key, GroupExpression value) {
return map(new GOne<>(key), value);
}
/**
* Create a new aggregating map expression using a backing LinkedHashMap
*
* @param key key for the map entries
* @param value value for the map entries
* @return wrapper expression
*/
public static AbstractGroupExpression, Map> map(
GroupExpression key, GroupExpression value) {
return new GMap.Mixin<>(key, value, GMap.createLinked(QPair.create(key, value)));
}
/**
* Create a new aggregating map expression using a backing TreeMap
*
* @param key key for the map entries
* @param value value for the map entries
* @return wrapper expression
*/
public static , V>
AbstractGroupExpression, SortedMap> sortedMap(
Expression key, Expression value) {
return GMap.createSorted(QPair.create(key, value));
}
/**
* Create a new aggregating map expression using a backing TreeMap
*
* @param key key for the map entries
* @param value value for the map entries
* @return wrapper expression
*/
public static >
AbstractGroupExpression, SortedMap> sortedMap(
GroupExpression key, Expression value) {
return sortedMap(key, new GOne<>(value));
}
/**
* Create a new aggregating map expression using a backing TreeMap
*
* @param key key for the map entries
* @param value value for the map entries
* @return wrapper expression
*/
public static , V, U>
AbstractGroupExpression, SortedMap> sortedMap(
Expression key, GroupExpression value) {
return sortedMap(new GOne<>(key), value);
}
/**
* Create a new aggregating map expression using a backing TreeMap
*
* @param key key for the map entries
* @param value value for the map entries
* @return wrapper expression
*/
public static , U>
AbstractGroupExpression, SortedMap> sortedMap(
GroupExpression key, GroupExpression value) {
return new GMap.Mixin<>(key, value, GMap.createSorted(QPair.create(key, value)));
}
/**
* Create a new aggregating map expression using a backing TreeMap using the given comparator
*
* @param key key for the map entries
* @param value value for the map entries
* @param comparator comparator for the created TreeMap instances
* @return wrapper expression
*/
public static AbstractGroupExpression, SortedMap> sortedMap(
Expression key, Expression value, Comparator super K> comparator) {
return GMap.createSorted(QPair.create(key, value), comparator);
}
/**
* Create a new aggregating map expression using a backing TreeMap using the given comparator
*
* @param key key for the map entries
* @param value value for the map entries
* @param comparator comparator for the created TreeMap instances
* @return wrapper expression
*/
public static AbstractGroupExpression, SortedMap> sortedMap(
GroupExpression key, Expression value, Comparator super T> comparator) {
return sortedMap(key, new GOne<>(value), comparator);
}
/**
* Create a new aggregating map expression using a backing TreeMap using the given comparator
*
* @param key key for the map entries
* @param value value for the map entries
* @param comparator comparator for the created TreeMap instances
* @return wrapper expression
*/
public static AbstractGroupExpression, SortedMap> sortedMap(
Expression key, GroupExpression value, Comparator super K> comparator) {
return sortedMap(new GOne<>(key), value, comparator);
}
/**
* Create a new aggregating map expression using a backing TreeMap using the given comparator
*
* @param key key for the map entries
* @param value value for the map entries
* @param comparator comparator for the created TreeMap instances
* @return wrapper expression
*/
public static AbstractGroupExpression, SortedMap> sortedMap(
GroupExpression key, GroupExpression value, Comparator super T> comparator) {
return new GMap.Mixin<>(key, value, GMap.createSorted(QPair.create(key, value), comparator));
}
protected GroupBy() {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy