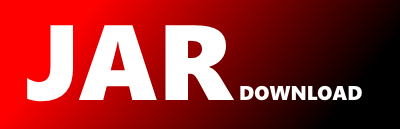
com.querydsl.core.group.guava.GTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of querydsl-guava Show documentation
Show all versions of querydsl-guava Show documentation
Utilities for creating group by factory expressions for Guava collection types
/*
* Copyright 2020, The Querydsl Team (http://www.querydsl.com/team)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.querydsl.core.group.guava;
import com.google.common.collect.HashBasedTable;
import com.google.common.collect.Table;
import com.google.common.collect.TreeBasedTable;
import com.mysema.commons.lang.Pair;
import com.querydsl.core.group.AbstractGroupExpression;
import com.querydsl.core.group.GroupCollector;
import com.querydsl.core.group.GroupExpression;
import com.querydsl.core.group.QPair;
import java.util.Comparator;
import java.util.HashMap;
import java.util.Map;
abstract class GTable>
extends AbstractGroupExpression, V>, M> {
private static final long serialVersionUID = 7106389414200843920L;
GTable(QPair, V> qpair) {
super(Table.class, qpair);
}
protected abstract M createTable();
public static GTable> create(QPair, W> expr) {
return new GTable<>(expr) {
@Override
protected Table createTable() {
return HashBasedTable.create();
}
};
}
public static , U extends Comparable super U>, W>
GTable> createSorted(QPair, W> expr) {
return new GTable<>(expr) {
@Override
protected TreeBasedTable createTable() {
return TreeBasedTable.create();
}
};
}
public static GTable> createSorted(
QPair, W> expr,
final Comparator super T> rowComparator,
final Comparator super U> columnComparator) {
return new GTable<>(expr) {
@Override
protected TreeBasedTable createTable() {
return TreeBasedTable.create(rowComparator, columnComparator);
}
};
}
@Override
public GroupCollector, V>, M> createGroupCollector() {
return new GroupCollector<>() {
private final M table = createTable();
@Override
public void add(Pair, V> pair) {
table.put(pair.getFirst().getFirst(), pair.getFirst().getSecond(), pair.getSecond());
}
@Override
public M get() {
return table;
}
};
}
static class Mixin>
extends AbstractGroupExpression, V>, RES> {
private static final long serialVersionUID = 1939989270493531116L;
private class GroupCollectorImpl implements GroupCollector, V>, RES> {
private final GroupCollector, W>, RES> groupCollector;
private final Table> rowCollectors = HashBasedTable.create();
private final Map, GroupCollector> columnCollectors =
new HashMap<>();
private final Map, GroupCollector> valueCollectors =
new HashMap<>();
GroupCollectorImpl() {
this.groupCollector = mixin.createGroupCollector();
}
@Override
public void add(Pair, V> pair) {
var first = pair.getFirst();
var rowKey = first.getFirst();
var columnKey = first.getSecond();
var rowCollector = rowCollectors.get(rowKey, columnKey);
if (rowCollector == null) {
rowCollector = rowExpression.createGroupCollector();
rowCollectors.put(rowKey, columnKey, rowCollector);
}
rowCollector.add(rowKey);
var columnCollector = columnCollectors.get(rowCollector);
if (columnCollector == null) {
columnCollector = columnExpression.createGroupCollector();
columnCollectors.put(rowCollector, columnCollector);
}
columnCollector.add(columnKey);
var valueCollector = valueCollectors.get(columnCollector);
if (valueCollector == null) {
valueCollector = valueExpression.createGroupCollector();
valueCollectors.put(columnCollector, valueCollector);
}
var second = pair.getSecond();
valueCollector.add(second);
}
@Override
public RES get() {
for (GroupCollector rowCollector : rowCollectors.values()) {
var rowKey = rowCollector.get();
var columnCollector = columnCollectors.get(rowCollector);
var columnKey = columnCollector.get();
var valueCollector = valueCollectors.get(columnCollector);
var value = valueCollector.get();
groupCollector.add(Pair.of(Pair.of(rowKey, columnKey), value));
}
return groupCollector.get();
}
}
private final GroupExpression, W>, RES> mixin;
private final GroupExpression rowExpression;
private final GroupExpression columnExpression;
private final GroupExpression valueExpression;
@SuppressWarnings({"rawtypes", "unchecked"})
Mixin(
GroupExpression rowExpression,
GroupExpression columnExpression,
GroupExpression valueExpression,
AbstractGroupExpression, W>, RES> mixin) {
super(
(Class) mixin.getType(),
QPair.create(
QPair.create(rowExpression.getExpression(), columnExpression.getExpression()),
valueExpression.getExpression()));
this.rowExpression = rowExpression;
this.columnExpression = columnExpression;
this.valueExpression = valueExpression;
this.mixin = mixin;
}
@Override
public GroupCollector, V>, RES> createGroupCollector() {
return new GroupCollectorImpl();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy