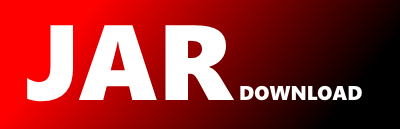
feign.Util Maven / Gradle / Ivy
/*
* Copyright 2012-2023 The Feign Authors
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package feign;
import static java.lang.String.format;
import static java.util.Objects.nonNull;
import java.io.ByteArrayOutputStream;
import java.io.Closeable;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.Reader;
import java.lang.reflect.Array;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.lang.reflect.WildcardType;
import java.nio.Buffer;
import java.nio.ByteBuffer;
import java.nio.CharBuffer;
import java.nio.charset.CharacterCodingException;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import java.util.TreeMap;
import java.util.function.Predicate;
import java.util.function.Supplier;
import java.util.stream.Stream;
/**
* Utilities, typically copied in from guava, so as to avoid dependency conflicts.
*/
public class Util {
/**
* The HTTP Content-Length header field name.
*/
public static final String CONTENT_LENGTH = "Content-Length";
/**
* The HTTP Content-Encoding header field name.
*/
public static final String CONTENT_ENCODING = "Content-Encoding";
/**
* The HTTP Accept-Encoding header field name.
*/
public static final String ACCEPT_ENCODING = "Accept-Encoding";
/**
* The HTTP Retry-After header field name.
*/
public static final String RETRY_AFTER = "Retry-After";
/**
* Value for the Content-Encoding header that indicates that GZIP encoding is in use.
*/
public static final String ENCODING_GZIP = "gzip";
/**
* Value for the Content-Encoding header that indicates that DEFLATE encoding is in use.
*/
public static final String ENCODING_DEFLATE = "deflate";
/**
* UTF-8: eight-bit UCS Transformation Format.
*/
public static final Charset UTF_8 = Charset.forName("UTF-8");
// com.google.common.base.Charsets
/**
* ISO-8859-1: ISO Latin Alphabet Number 1 (ISO-LATIN-1).
*/
public static final Charset ISO_8859_1 = Charset.forName("ISO-8859-1");
private static final int BUF_SIZE = 0x800; // 2K chars (4K bytes)
/**
* Type literal for {@code Map}.
*/
public static final Type MAP_STRING_WILDCARD =
new Types.ParameterizedTypeImpl(null, Map.class, String.class,
new Types.WildcardTypeImpl(new Type[] {Object.class}, new Type[0]));
private Util() { // no instances
}
/**
* Copy of {@code com.google.common.base.Preconditions#checkArgument}.
*/
public static void checkArgument(boolean expression,
String errorMessageTemplate,
Object... errorMessageArgs) {
if (!expression) {
throw new IllegalArgumentException(
format(errorMessageTemplate, errorMessageArgs));
}
}
/**
* Copy of {@code com.google.common.base.Preconditions#checkNotNull}.
*/
public static T checkNotNull(T reference,
String errorMessageTemplate,
Object... errorMessageArgs) {
if (reference == null) {
// If either of these parameters is null, the right thing happens anyway
throw new NullPointerException(
format(errorMessageTemplate, errorMessageArgs));
}
return reference;
}
/**
* Copy of {@code com.google.common.base.Preconditions#checkState}.
*/
public static void checkState(boolean expression,
String errorMessageTemplate,
Object... errorMessageArgs) {
if (!expression) {
throw new IllegalStateException(
format(errorMessageTemplate, errorMessageArgs));
}
}
/**
* Identifies a method as a default instance method.
*/
public static boolean isDefault(Method method) {
// Default methods are public non-abstract, non-synthetic, and non-static instance methods
// declared in an interface.
// method.isDefault() is not sufficient for our usage as it does not check
// for synthetic methods. As a result, it picks up overridden methods as well as actual default
// methods.
final int SYNTHETIC = 0x00001000;
return ((method.getModifiers()
& (Modifier.ABSTRACT | Modifier.PUBLIC | Modifier.STATIC | SYNTHETIC)) == Modifier.PUBLIC)
&& method.getDeclaringClass().isInterface();
}
/**
* Adapted from {@code com.google.common.base.Strings#emptyToNull}.
*/
public static String emptyToNull(String string) {
return string == null || string.isEmpty() ? null : string;
}
/**
* Removes values from the array that meet the criteria for removal via the supplied
* {@link Predicate} value
*/
@SuppressWarnings("unchecked")
public static T[] removeValues(T[] values, Predicate shouldRemove, Class type) {
Collection collection = new ArrayList<>(values.length);
for (T value : values) {
if (shouldRemove.negate().test(value)) {
collection.add(value);
}
}
T[] array = (T[]) Array.newInstance(type, collection.size());
return collection.toArray(array);
}
/**
* Adapted from {@code com.google.common.base.Strings#emptyToNull}.
*/
@SuppressWarnings("unchecked")
public static T[] toArray(Iterable extends T> iterable, Class type) {
Collection collection;
if (iterable instanceof Collection) {
collection = (Collection) iterable;
} else {
collection = new ArrayList<>();
for (T element : iterable) {
collection.add(element);
}
}
T[] array = (T[]) Array.newInstance(type, collection.size());
return collection.toArray(array);
}
/**
* Returns an unmodifiable collection which may be empty, but is never null.
*/
public static Collection valuesOrEmpty(Map> map, String key) {
Collection values = map.get(key);
return values != null ? values : Collections.emptyList();
}
public static void ensureClosed(Closeable closeable) {
if (closeable != null) {
try {
closeable.close();
} catch (IOException ignored) { // NOPMD
}
}
}
/**
* Moved to {@code feign.Types.resolveLastTypeParameter}
*/
@Deprecated
public static Type resolveLastTypeParameter(Type genericContext, Class> supertype)
throws IllegalStateException {
return Types.resolveLastTypeParameter(genericContext, supertype);
}
/**
* This returns well known empty values for well-known java types. This returns null for types not
* in the following list.
*
*
* - {@code [Bb]oolean}
* - {@code byte[]}
* - {@code Collection}
* - {@code Iterator}
* - {@code List}
* - {@code Map}
* - {@code Set}
*
*
*
* When {@link Feign.Builder#dismiss404() decoding HTTP 404 status}, you'll need to teach decoders
* a default empty value for a type. This method cheaply supports typical types by only looking at
* the raw type (vs type hierarchy). Decorate for sophistication.
*/
public static Object emptyValueOf(Type type) {
return EMPTIES.getOrDefault(Types.getRawType(type), () -> null).get();
}
private static final Map, Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy