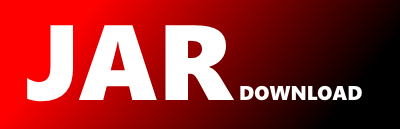
feign.metrics5.MeteredAsyncClient Maven / Gradle / Ivy
The newest version!
/*
* Copyright © 2012 The Feign Authors ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package feign.metrics5;
import feign.AsyncClient;
import feign.FeignException;
import feign.Request;
import feign.Request.Options;
import feign.RequestTemplate;
import feign.Response;
import io.dropwizard.metrics5.MetricRegistry;
import io.dropwizard.metrics5.Timer;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
/** Warp feign {@link AsyncClient} with metrics. */
public class MeteredAsyncClient extends BaseMeteredClient implements AsyncClient
© 2015 - 2025 Weber Informatics LLC | Privacy Policy