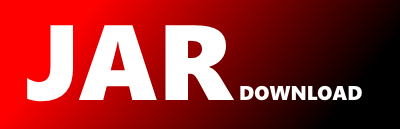
io.github.openunirest.request.HttpClientHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unirest-java Show documentation
Show all versions of unirest-java Show documentation
Simplified, lightweight HTTP client library
The newest version!
package io.github.openunirest.request;
import io.github.openunirest.http.HttpResponse;
import io.github.openunirest.http.async.Callback;
import io.github.openunirest.http.async.CallbackFuture;
import io.github.openunirest.http.async.utils.AsyncIdleConnectionMonitorThread;
import io.github.openunirest.http.exceptions.UnirestException;
import io.github.openunirest.http.options.Option;
import io.github.openunirest.http.options.Options;
import io.github.openunirest.http.utils.ClientFactory;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpRequestBase;
import org.apache.http.client.methods.HttpUriRequest;
import org.apache.http.concurrent.FutureCallback;
import org.apache.http.impl.nio.client.CloseableHttpAsyncClient;
import java.util.Objects;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
class HttpClientHelper {
public static HttpResponse request(HttpRequest request,
Function> transformer) {
HttpRequestBase requestObj = RequestPrep.prepareRequest(request, false);
HttpClient client = ClientFactory.getHttpClient(); // The
// DefaultHttpClient
// is thread-safe
org.apache.http.HttpResponse response;
try {
response = client.execute(requestObj);
HttpResponse httpResponse = transformer.apply(response);
requestObj.releaseConnection();
return httpResponse;
} catch (Exception e) {
throw new UnirestException(e);
} finally {
requestObj.releaseConnection();
}
}
public static CompletableFuture> requestAsync(HttpRequest httpRequest, Function> transformer) {
return requestAsync(httpRequest, transformer, new CompletableFuture<>());
}
public static CompletableFuture> requestAsync(HttpRequest request, Function> transformer, Callback callback) {
return requestAsync(request, transformer, CallbackFuture.wrap(callback));
}
public static CompletableFuture> requestAsync(HttpRequest request,
Function> transformer,
CompletableFuture> callback) {
Objects.requireNonNull(callback);
HttpUriRequest requestObj = RequestPrep.prepareRequest(request, true);
asyncClient()
.execute(requestObj, new FutureCallback() {
@Override
public void completed(org.apache.http.HttpResponse httpResponse) {
callback.complete(transformer.apply(httpResponse));
}
@Override
public void failed(Exception e) {
callback.completeExceptionally(e);
}
@Override
public void cancelled() {
callback.completeExceptionally(new UnirestException("canceled"));
}
});
return callback;
}
private static CloseableHttpAsyncClient asyncClient() {
CloseableHttpAsyncClient asyncHttpClient = ClientFactory.getAsyncHttpClient();
if (!asyncHttpClient.isRunning()) {
tryStart(asyncHttpClient);
}
return asyncHttpClient;
}
private static synchronized void tryStart(CloseableHttpAsyncClient asyncHttpClient) {
if (!asyncHttpClient.isRunning()) {
asyncHttpClient.start();
AsyncIdleConnectionMonitorThread asyncIdleConnectionMonitorThread = (AsyncIdleConnectionMonitorThread) Options.getOption(Option.ASYNC_MONITOR);
asyncIdleConnectionMonitorThread.tryStart();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy