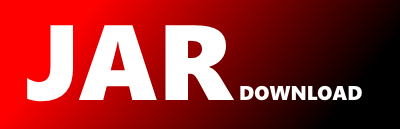
io.github.palexdev.mfxeffects.animations.motion.Cubic Maven / Gradle / Ivy
Show all versions of materialfx-all Show documentation
/*
* Copyright (C) 2023 Parisi Alessandro - [email protected]
* This file is part of MaterialFX (https://github.com/palexdev/MaterialFX)
*
* MaterialFX is free software: you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation; either version 3 of the License,
* or (at your option) any later version.
*
* MaterialFX is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with MaterialFX. If not, see .
*/
package io.github.palexdev.mfxeffects.animations.motion;
import io.github.palexdev.mfxeffects.animations.base.Curve;
/**
* A cubic polynomial mapping of the unit interval.
*
* Implements third-order Bézier curves.
*
* Video examples of various curves based on this:
* - Fast Linear To Slow Ease In
*
- Ease
*
- Ease In
*
- Ease In Sine
*
- Ease In Quad
*
- Ease In Cubic
*
- Ease In Quart
*
- Ease In Quint
*
- Ease In Expo
*
- Ease In Circ
*
- Ease In Back
*
- Ease Out
*
- Ease Out Sine
*
- Ease Out Quad
*
- Ease Out Cubic
*
- Ease Out Quart
*
- Ease Out Quint
*
- Ease Out Expo
*
- Ease Out Circ
*
- Ease Out Back
*
- Ease In Out
*
- Ease In Out Sine
*
- Ease In Out Quad
*
- Ease In Out Expo
*
- Ease In Out Circ
*
- Ease In Out Back
*
- Fast Out Slow In
*
- Slow Middle
*/
public class Cubic extends Curve {
private final double x1;
private final double y1;
private final double x2;
private final double y2;
private static final double CUBIC_ERROR_BOUND = 0.001;
public Cubic(double x1, double y1, double x2, double y2) {
this.x1 = x1;
this.y1 = y1;
this.x2 = x2;
this.y2 = y2;
}
double elevateCubic(double a, double b, double m) {
return 3 * a * (1 - m) * (1 - m) * m +
3 * b * (1 - m) * m * m +
m * m * m;
}
@Override
public double curve(double t) {
double start = 0.0;
double end = 1.0;
while (true) {
final double midpoint = (start + end) / 2;
final double estimate = elevateCubic(x1, x2, midpoint);
if (Math.abs(t - estimate) < CUBIC_ERROR_BOUND) {
return elevateCubic(y1, y2, midpoint);
}
if (estimate < t) {
start = midpoint;
} else {
end = midpoint;
}
}
}
}