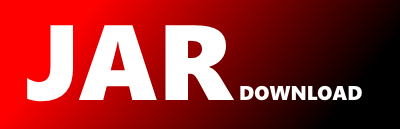
io.github.palexdev.mfxcore.settings.Settings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of materialfx-all Show documentation
Show all versions of materialfx-all Show documentation
Material Design/Modern components for JavaFX, now packed as a single Jar
The newest version!
/*
* Copyright (C) 2023 Parisi Alessandro - [email protected]
* This file is part of MaterialFX (https://github.com/palexdev/MaterialFX)
*
* MaterialFX is free software: you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation; either version 3 of the License,
* or (at your option) any later version.
*
* MaterialFX is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with MaterialFX. If not, see .
*/
package io.github.palexdev.mfxcore.settings;
import java.util.*;
import java.util.prefs.PreferenceChangeListener;
import java.util.prefs.Preferences;
public abstract class Settings {
//================================================================================
// Properties
//================================================================================
protected final Preferences prefs;
protected static final Map, Set>> settingsDB = new HashMap<>();
//================================================================================
// Constructors
//================================================================================
protected Settings() {
prefs = init();
}
//================================================================================
// Abstract Methods
//================================================================================
protected abstract String node();
//================================================================================
// Methods
//================================================================================
protected Preferences init() {
if (prefs == null) return Preferences.userRoot().node(node());
return prefs;
}
protected StringSetting registerString(String name, String description, String defaultValue) {
StringSetting setting = StringSetting.of(name, description, defaultValue, this);
Set> set = settingsDB.computeIfAbsent(getClass(), c -> new LinkedHashSet<>());
set.add(setting);
return setting;
}
protected BooleanSetting registerBoolean(String name, String description, boolean defaultValue) {
BooleanSetting setting = BooleanSetting.of(name, description, defaultValue, this);
Set> set = settingsDB.computeIfAbsent(getClass(), c -> new LinkedHashSet<>());
set.add(setting);
return setting;
}
protected NumberSetting registerDouble(String name, String description, double defaultValue) {
NumberSetting setting = NumberSetting.forDouble(name, description, defaultValue, this);
Set> set = settingsDB.computeIfAbsent(getClass(), c -> new LinkedHashSet<>());
set.add(setting);
return setting;
}
protected NumberSetting registerFloat(String name, String description, float defaultValue) {
NumberSetting setting = NumberSetting.forFloat(name, description, defaultValue, this);
Set> set = settingsDB.computeIfAbsent(getClass(), c -> new LinkedHashSet<>());
set.add(setting);
return setting;
}
protected NumberSetting registerInteger(String name, String description, int defaultValue) {
NumberSetting setting = NumberSetting.forInteger(name, description, defaultValue, this);
Set> set = settingsDB.computeIfAbsent(getClass(), c -> new LinkedHashSet<>());
set.add(setting);
return setting;
}
protected NumberSetting registerLong(String name, String description, long defaultValue) {
NumberSetting setting = NumberSetting.forLong(name, description, defaultValue, this);
Set> set = settingsDB.computeIfAbsent(getClass(), c -> new LinkedHashSet<>());
set.add(setting);
return setting;
}
public void onChange(PreferenceChangeListener pcl) {
prefs.addPreferenceChangeListener(pcl);
}
public void removeOnChange(PreferenceChangeListener pcl) {
prefs.removePreferenceChangeListener(pcl);
}
public void reset() {
Optional.ofNullable(settingsDB.get(getClass()))
.ifPresent(s -> s.forEach(Setting::reset));
}
public static void reset(Class extends Settings> klass) {
Optional.ofNullable(settingsDB.get(klass))
.ifPresent(s -> s.forEach(Setting::reset));
}
public static void resetAll() {
settingsDB.values().stream()
.flatMap(Collection::stream)
.forEach(Setting::reset);
}
//================================================================================
// Getters/Setters
//================================================================================
protected Preferences prefs() {
return prefs;
}
public static Set> getSettings(Class extends Settings> c) {
return Optional.ofNullable(settingsDB.get(c))
.map(Collections::unmodifiableSet)
.orElse(Collections.emptySet());
}
public static Map, Set>> getSettingsDB() {
return Collections.unmodifiableMap(settingsDB);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy