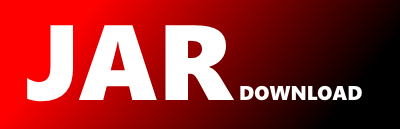
io.github.palexdev.mfxeffects.enums.ElevationLevel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of materialfx-all Show documentation
Show all versions of materialfx-all Show documentation
Material Design/Modern components for JavaFX, now packed as a single Jar
The newest version!
/*
* Copyright (C) 2023 Parisi Alessandro - [email protected]
* This file is part of MaterialFX (https://github.com/palexdev/MaterialFX)
*
* MaterialFX is free software: you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation; either version 3 of the License,
* or (at your option) any later version.
*
* MaterialFX is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with MaterialFX. If not, see .
*/
package io.github.palexdev.mfxeffects.enums;
import io.github.palexdev.mfxeffects.animations.Animations.KeyFrames;
import io.github.palexdev.mfxeffects.animations.Animations.TimelineBuilder;
import io.github.palexdev.mfxeffects.animations.motion.Motion;
import javafx.animation.Interpolator;
import javafx.scene.effect.BlurType;
import javafx.scene.effect.DropShadow;
import javafx.scene.paint.Color;
import java.util.Arrays;
/**
* Enumerator which defines 6 levels of {@code DropShadow} effects from {@code LEVEL0} to {@code LEVEL5}.
*/
public enum ElevationLevel {
// 0dp
LEVEL0(Color.rgb(0, 0, 0, 0), 0, 0, 0, 0),
// 1dp
LEVEL1(3, 0.12, 0, 2),
// 3dp
LEVEL2(8, 0.16, 0, 3),
// 6dp
LEVEL3(18, 0.19, 0, 3),
// 8dp
LEVEL4(14, 0.25, 0, 5),
// 12dp
LEVEL5(16, 0.30, 0, 7);
private final Color color;
private final double radius;
private final double spread;
private final double offsetX;
private final double offsetY;
ElevationLevel(double radius, double spread, double offsetX, double offsetY) {
this(Color.rgb(0, 0, 0, 0.20), radius, spread, offsetX, offsetY);
}
ElevationLevel(Color color, double radius, double spread, double offsetX, double offsetY) {
this.color = color;
this.radius = radius;
this.spread = spread;
this.offsetX = offsetX;
this.offsetY = offsetY;
}
public Color getColor() {
return color;
}
public double getRadius() {
return radius;
}
public double getSpread() {
return spread;
}
public double getOffsetX() {
return offsetX;
}
public double getOffsetY() {
return offsetY;
}
/**
* Retrieves the next {@code DepthLevel} associated with {@code this} enumerator.
*
* @return The next {@code DepthLevel}
*/
public ElevationLevel next() {
return values()[(this.ordinal() + 1) % values().length];
}
public void animateTo(DropShadow current, ElevationLevel next) {
Interpolator i = Motion.EASE;
TimelineBuilder.build()
.add(KeyFrames.of(1, current.offsetXProperty(), next.getOffsetX(), i))
.add(KeyFrames.of(1, current.offsetYProperty(), next.getOffsetY(), i))
.add(KeyFrames.of(250, current.radiusProperty(), next.getRadius(), i))
.add(KeyFrames.of(250, current.spreadProperty(), next.getSpread(), i))
.getAnimation().play();
}
public DropShadow toShadow() {
return new DropShadow(
BlurType.GAUSSIAN, getColor(),
getRadius(), getSpread(),
getOffsetX(), getOffsetY()
);
}
/**
* Attempts to get the corresponding {@code DepthLevel} of the given {@link DropShadow} effect.
* If the given effect is not recognized as being generated by this class the {@code null} is returned.
*
* Uses {@link #levelEqualsShadow(ElevationLevel, DropShadow)} to check for equality.
*/
public static ElevationLevel from(DropShadow shadow) {
return Arrays.stream(values()).filter(depthLevel -> levelEqualsShadow(depthLevel, shadow))
.findFirst()
.orElse(null);
}
/**
* Checks if the given {@link DropShadow} effect is equal to the given {@code DepthLevel}.
*/
public static boolean levelEqualsShadow(ElevationLevel level, DropShadow shadow) {
return level.color.equals(shadow.getColor()) &&
level.offsetX == shadow.getOffsetX() &&
level.offsetY == shadow.getOffsetY() &&
level.radius == shadow.getRadius() &&
level.spread == shadow.getSpread();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy