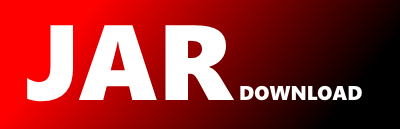
org.scenicview.view.tabs.DetailsTab Maven / Gradle / Ivy
The newest version!
/*
* Scenic View,
* Copyright (C) 2012 Jonathan Giles, Ander Ruiz, Amy Fowler
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.scenicview.view.tabs;
import javafx.scene.Node;
import javafx.scene.control.*;
import javafx.scene.image.ImageView;
import javafx.scene.input.Clipboard;
import javafx.scene.input.ClipboardContent;
import javafx.scene.layout.VBox;
import org.fxconnector.details.Detail;
import org.fxconnector.details.DetailPaneType;
import org.fxconnector.node.SVNode;
import org.scenicview.view.ContextMenuContainer;
import org.scenicview.view.DisplayUtils;
import org.scenicview.view.ScenicViewGui;
import org.scenicview.view.tabs.details.GDetailPane;
import org.scenicview.view.tabs.details.GDetailPane.RemotePropertySetter;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Consumer;
/**
*
*/
public class DetailsTab extends Tab implements ContextMenuContainer {
public static final String TAB_NAME = "Details";
final List gDetailPanes = new ArrayList<>();
public static boolean showDefaultProperties = true;
private final Consumer loader;
private final ScenicViewGui scenicView;
final VBox vbox;
Menu menu;
MenuItem dumpDetails;
public DetailsTab(final ScenicViewGui view, final Consumer loader) {
super(TAB_NAME);
this.scenicView = view;
this.loader = loader;
ScrollPane scrollPane = new ScrollPane();
scrollPane.setHbarPolicy(ScrollPane.ScrollBarPolicy.NEVER);
scrollPane.setFitToWidth(true);
vbox = new VBox();
vbox.setFillWidth(true);
scrollPane.setContent(vbox);
getStyleClass().add("all-details-pane");
setGraphic(new ImageView(DisplayUtils.getUIImage("details.png")));
setContent(scrollPane);
setClosable(false);
}
public void setShowDefaultProperties(final boolean show) {
showDefaultProperties = show;
}
public void filterProperties(final String text) {
for (final GDetailPane type : gDetailPanes) {
type.filterProperties(text);
updatedDetailPane(type);
}
}
public void updateDetails(final DetailPaneType type, final String paneName, final List details, final RemotePropertySetter setter) {
final GDetailPane pane = getPane(type, paneName);
pane.updateDetails(details, setter);
updatedDetailPane(pane);
}
private void updatedDetailPane(final GDetailPane pane) {
boolean detailVisible = false;
for (final Node gridChild : pane.gridpane.getChildren()) {
detailVisible = gridChild.isVisible();
if (detailVisible)
break;
}
pane.setExpanded(detailVisible);
pane.setManaged(detailVisible);
pane.setVisible(detailVisible);
updateDump();
}
public void updateDetail(final DetailPaneType type, final String paneName, final Detail detail) {
getPane(type, paneName).updateDetail(detail);
}
private GDetailPane getPane(final DetailPaneType type, final String paneName) {
GDetailPane pane = null;
boolean found = false;
for (GDetailPane gDetailPane : gDetailPanes) {
if (gDetailPane.type == type) {
found = true;
pane = gDetailPane;
break;
}
}
if (!found) {
pane = new GDetailPane(scenicView, type, paneName, loader);
gDetailPanes.add(pane);
vbox.getChildren().add(pane);
}
return pane;
}
@Override
public Menu getMenu() {
if (menu == null) {
menu = new Menu("Details");
// --- copy to clipboard
dumpDetails = new MenuItem("Copy Details to Clipboard");
dumpDetails.setOnAction(event -> {
final StringBuilder sb = new StringBuilder();
for (GDetailPane gDetailPane : gDetailPanes) {
sb.append(gDetailPane);
}
final Clipboard clipboard = Clipboard.getSystemClipboard();
final ClipboardContent content = new ClipboardContent();
content.putString(sb.toString());
clipboard.setContent(content);
});
updateDump();
menu.getItems().addAll(dumpDetails);
// --- show default properties
final CheckMenuItem showDefaultProperties = scenicView.buildCheckMenuItem("Show Default Properties", "Show default properties",
"Hide default properties", "showDefaultProperties", Boolean.TRUE);
showDefaultProperties.selectedProperty().addListener(arg0 -> {
setShowDefaultProperties(showDefaultProperties.isSelected());
final SVNode selected = scenicView.getSelectedNode();
scenicView.configurationUpdated();
scenicView.setSelectedNode(scenicView.activeStage, selected);
});
setShowDefaultProperties(showDefaultProperties.isSelected());
menu.getItems().addAll(showDefaultProperties);
// --- show css properties
final CheckMenuItem showCSSProperties = scenicView.buildCheckMenuItem("Show CSS Properties", "Show CSS properties", "Hide CSS properties",
"showCSSProperties", Boolean.FALSE);
showCSSProperties.selectedProperty().addListener(arg0 -> {
scenicView.configuration.setCSSPropertiesDetail(showCSSProperties.isSelected());
final SVNode selected = scenicView.getSelectedNode();
scenicView.configurationUpdated();
scenicView.setSelectedNode(scenicView.activeStage, selected);
});
scenicView.configuration.setCSSPropertiesDetail(showCSSProperties.isSelected());
menu.getItems().addAll(showCSSProperties);
}
return menu;
}
private void updateDump() {
boolean anyVisible = false;
for (GDetailPane gDetailPane : gDetailPanes) {
if (gDetailPane.isVisible()) {
anyVisible = true;
break;
}
}
dumpDetails.setDisable(!anyVisible);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy