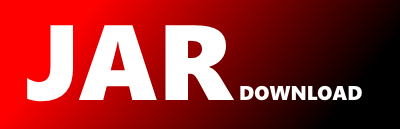
io.github.peakc.impl.AliyunCloudStorageService Maven / Gradle / Ivy
package io.github.peakc.impl;
import com.aliyun.oss.OSSClient;
import com.aliyun.oss.model.DownloadFileRequest;
import com.aliyun.oss.model.SimplifiedObjectMeta;
import io.github.peakc.StorageConfig;
import io.github.peakc.StorageService;
import lombok.Getter;
import org.apache.commons.io.FileUtils;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.InputStream;
import java.net.URL;
import java.util.Date;
/**
* 阿里云存储
*/
public class AliyunCloudStorageService extends StorageService {
@Getter
private OSSClient client;
public AliyunCloudStorageService(StorageConfig config, boolean inner) {
this.config = config;
if (inner) {
this.config.setAliyunDomain(config.getAliyunDomainInner());
this.config.setAliyunEndPoint(config.getAliyunEndPointInner());
}
//初始化
init();
}
private void init() {
client = new OSSClient(config.getAliyunEndPoint(), config.getAliyunAccessKeyId(), config.getAliyunAccessKeySecret());
}
private String getFullUrl(Long expirSeconds, String objectName) {
// 设置URL过期时间为1小时。
Date expiration = new Date(System.currentTimeMillis() + expirSeconds * 1000);
// 生成以GET方法访问的签名URL,访客可以直接通过浏览器访问相关内容。
URL url = client.generatePresignedUrl(config.getAliyunBucketName(), objectName, expiration);
return url.toString();
}
@Override
public void deleteFile(String path) {
client.deleteObject(config.getAliyunBucketName(), path);
}
@Override
public String getFullPath(String path) {
long expirSeconds = 3600L;
if (null != config.getAliyunExpirSeconds() && 0 != config.getAliyunExpirSeconds()) {
expirSeconds = config.getAliyunExpirSeconds();
}
return getFullUrl(expirSeconds, path);
}
@Override
public void downloadFile(String key, String downloadFile) {
this.downloadFile(config.getAliyunBucketName(), key, downloadFile);
}
@Override
public void downloadFile(String bucketName, String key, String downloadFile) {
try {
FileUtils.forceMkdirParent(new File(downloadFile));
DownloadFileRequest downloadFileRequest = new DownloadFileRequest(bucketName, key, downloadFile, 102400L);
client.downloadFile(downloadFileRequest);
} catch (Throwable throwable) {
throw new RuntimeException("下载失败:", throwable);
}
}
@Override
public String upload(byte[] data, String path) {
return upload(new ByteArrayInputStream(data), path);
}
@Override
public String upload(InputStream inputStream, String path) {
try {
client.putObject(config.getAliyunBucketName(), path, inputStream);
} catch (Exception e) {
throw new RuntimeException("上传文件失败,请检查配置信息", e);
}
return path;
}
@Override
public String uploadSuffix(byte[] data, String suffix) {
return upload(data, getPath(config.getAliyunPrefix(), suffix));
}
@Override
public String uploadSuffix(InputStream inputStream, String suffix) {
return upload(inputStream, getPath(config.getAliyunPrefix(), suffix));
}
@Override
public long getFileLength(String key) {
long size = 0;
SimplifiedObjectMeta objectMeta = client.getSimplifiedObjectMeta(config.getAliyunBucketName(), key);
if (null != objectMeta) {
size = objectMeta.getSize();
}
return size;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy