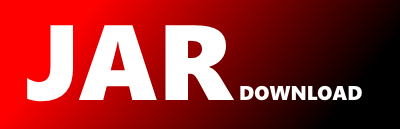
com.penglecode.codeforce.common.support.MapLambdaBuilder Maven / Gradle / Ivy
The newest version!
package com.penglecode.codeforce.common.support;
import org.springframework.util.Assert;
import org.springframework.util.ObjectUtils;
import java.lang.reflect.Field;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.function.Supplier;
/**
* 基于JAVA8方法引用(lambda的一种特殊形式)方式来快速通过普通JavaBean构造Map的通用Builder
* 使用示例:
* Account account = ObjectLambdaBuilder.of(Account::new)
* .with(Account::setAcctNo, "27182821221122219")
* .with(Account::setCustNo, "71283828182823291929")
* .with(Account::setIdCode, null)
* .with(Account::setCustName, "阿三")
* .with(Account::setMobile, "13812345678")
* .build();
* System.out.println(account);
*
* Map map = MapLambdaBuilder.of(account)
* .with(Account::getAcctNo)
* .with(Account::getCustNo)
* .with(Account::getCustName)
* .with(Account::getIdCode, "61283291929382912X")
* .with(Account::getMobile)
* .build();
* System.out.println(map);
*
* @author pengpeng
* @version 1.0
*/
@SuppressWarnings("unchecked")
public class MapLambdaBuilder {
private final Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy