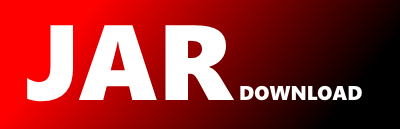
io.platin.geo.eth.Circle Maven / Gradle / Ivy
package io.platin.geo.eth;
import java.math.BigInteger;
import java.util.Arrays;
import java.util.List;
import java.util.concurrent.Callable;
import org.web3j.abi.FunctionEncoder;
import org.web3j.abi.TypeReference;
import org.web3j.abi.datatypes.DynamicArray;
import org.web3j.abi.datatypes.Function;
import org.web3j.abi.datatypes.Type;
import org.web3j.abi.datatypes.generated.Int32;
import org.web3j.crypto.Credentials;
import org.web3j.protocol.Web3j;
import org.web3j.protocol.core.RemoteCall;
import org.web3j.tx.Contract;
import org.web3j.tx.TransactionManager;
/**
* Auto generated code.
*
Do not modify!
*
Please use the web3j command line tools,
* or the org.web3j.codegen.SolidityFunctionWrapperGenerator in the
* codegen module to update.
*
*
Generated with web3j version 3.4.0.
*/
class Circle extends Contract {
private static final String BINARY = "608060405234801561001057600080fd5b5060405161052838038061052883398101604052805101805160031461003557600080fd5b805163055d4a80908290600090811061004a57fe5b9060200190602002015160030b131580156100865750805163055d4a7f19908290600090811061007657fe5b9060200190602002015160030b12155b151561009157600080fd5b8051630aba950090829060019081106100a657fe5b9060200190602002015160030b131580156100e257508051630aba94ff1990829060019081106100d257fe5b9060200190602002015160030b12155b15156100ed57600080fd5b80516301312d00908290600290811061010257fe5b9060200190602002015160030b1315801561013a575080516001908290600290811061012a57fe5b9060200190602002015160030b12155b151561014557600080fd5b80600081518110151561015457fe5b602090810290910101516000805460039290920b63ffffffff1663ffffffff1990921691909117905580518190600190811061018c57fe5b90602001906020020151600060046101000a81548163ffffffff021916908360030b63ffffffff1602179055508060028151811015156101c857fe5b90602001906020020151600060086101000a81548163ffffffff021916908360030b63ffffffff16021790555050610323806102056000396000f3006080604052600436106100a35763ffffffff7c0100000000000000000000000000000000000000000000000000000000600035041663217f435381146100a85780632c2d5381146100d65780633bc5de30146100eb5780634485e7c21461015057806346e960ec146101655780634fd7d76a1461017a578063589af69c1461018f57806367d82c9a146101a4578063d114b056146101b9578063fc291485146101ce575b600080fd5b3480156100b457600080fd5b506100bd6101e3565b60408051600392830b90920b8252519081900360200190f35b3480156100e257600080fd5b506100bd6101f8565b3480156100f757600080fd5b50610100610200565b60408051602080825283518183015283519192839290830191858101910280838360005b8381101561013c578181015183820152602001610124565b505050509050019250505060405180910390f35b34801561015c57600080fd5b506100bd6102b6565b34801561017157600080fd5b506100bd6102be565b34801561018657600080fd5b506100bd6102c3565b34801561019b57600080fd5b506100bd6102cc565b3480156101b057600080fd5b506100bd6102dd565b3480156101c557600080fd5b506100bd6102e6565b3480156101da57600080fd5b506100bd6102ee565b60005468010000000000000000900460030b81565b6301312d0081565b60408051600380825260808201909252606091829190602082018380388339505060008054835193945060030b92849250811061023957fe5b600392830b830b60209182029092010152600054825164010000000090910490910b908290600190811061026957fe5b600392830b830b6020918202909201015260005482516801000000000000000090910490910b908290600290811061029d57fe5b600392830b90920b602092830290910190910152919050565b63055d4a8081565b600181565b60005460030b81565b600054640100000000900460030b81565b63055d4a7f1981565b630aba950081565b630aba94ff19815600a165627a7a7230582004f2b9ddc46288ca5a90cdd40c71f24ab664cbe023643d24a6b5ecdd44311b3c0029";
public static final String FUNC_RADIUS = "radius";
public static final String FUNC_MAX_RADIUS = "MAX_RADIUS";
public static final String FUNC_GETDATA = "getData";
public static final String FUNC_MAX_LATITUDE = "MAX_LATITUDE";
public static final String FUNC_MIN_RADIUS = "MIN_RADIUS";
public static final String FUNC_LATITUDE = "latitude";
public static final String FUNC_LONGITUDE = "longitude";
public static final String FUNC_MIN_LATITUDE = "MIN_LATITUDE";
public static final String FUNC_MAX_LONGITUDE = "MAX_LONGITUDE";
public static final String FUNC_MIN_LONGITUDE = "MIN_LONGITUDE";
protected Circle(String contractAddress, Web3j web3j, Credentials credentials, BigInteger gasPrice, BigInteger gasLimit) {
super(BINARY, contractAddress, web3j, credentials, gasPrice, gasLimit);
}
protected Circle(String contractAddress, Web3j web3j, TransactionManager transactionManager, BigInteger gasPrice, BigInteger gasLimit) {
super(BINARY, contractAddress, web3j, transactionManager, gasPrice, gasLimit);
}
public RemoteCall radius() {
final Function function = new Function(FUNC_RADIUS,
Arrays.asList(),
Arrays.>asList(new TypeReference() {}));
return executeRemoteCallSingleValueReturn(function, BigInteger.class);
}
public RemoteCall MAX_RADIUS() {
final Function function = new Function(FUNC_MAX_RADIUS,
Arrays.asList(),
Arrays.>asList(new TypeReference() {}));
return executeRemoteCallSingleValueReturn(function, BigInteger.class);
}
public RemoteCall getData() {
final Function function = new Function(FUNC_GETDATA,
Arrays.asList(),
Arrays.>asList(new TypeReference>() {}));
return new RemoteCall(
new Callable() {
@Override
@SuppressWarnings("unchecked")
public List call() throws Exception {
List result = (List) executeCallSingleValueReturn(function, List.class);
return convertToNative(result);
}
});
}
public RemoteCall MAX_LATITUDE() {
final Function function = new Function(FUNC_MAX_LATITUDE,
Arrays.asList(),
Arrays.>asList(new TypeReference() {}));
return executeRemoteCallSingleValueReturn(function, BigInteger.class);
}
public RemoteCall MIN_RADIUS() {
final Function function = new Function(FUNC_MIN_RADIUS,
Arrays.asList(),
Arrays.>asList(new TypeReference() {}));
return executeRemoteCallSingleValueReturn(function, BigInteger.class);
}
public RemoteCall latitude() {
final Function function = new Function(FUNC_LATITUDE,
Arrays.asList(),
Arrays.>asList(new TypeReference() {}));
return executeRemoteCallSingleValueReturn(function, BigInteger.class);
}
public RemoteCall longitude() {
final Function function = new Function(FUNC_LONGITUDE,
Arrays.asList(),
Arrays.>asList(new TypeReference() {}));
return executeRemoteCallSingleValueReturn(function, BigInteger.class);
}
public RemoteCall MIN_LATITUDE() {
final Function function = new Function(FUNC_MIN_LATITUDE,
Arrays.asList(),
Arrays.>asList(new TypeReference() {}));
return executeRemoteCallSingleValueReturn(function, BigInteger.class);
}
public RemoteCall MAX_LONGITUDE() {
final Function function = new Function(FUNC_MAX_LONGITUDE,
Arrays.asList(),
Arrays.>asList(new TypeReference() {}));
return executeRemoteCallSingleValueReturn(function, BigInteger.class);
}
public RemoteCall MIN_LONGITUDE() {
final Function function = new Function(FUNC_MIN_LONGITUDE,
Arrays.asList(),
Arrays.>asList(new TypeReference() {}));
return executeRemoteCallSingleValueReturn(function, BigInteger.class);
}
public static RemoteCall deploy(Web3j web3j, Credentials credentials, BigInteger gasPrice, BigInteger gasLimit, List data) {
String encodedConstructor = FunctionEncoder.encodeConstructor(Arrays.asList(new org.web3j.abi.datatypes.DynamicArray(
org.web3j.abi.Utils.typeMap(data, org.web3j.abi.datatypes.generated.Int32.class))));
return deployRemoteCall(Circle.class, web3j, credentials, gasPrice, gasLimit, BINARY, encodedConstructor);
}
public static RemoteCall deploy(Web3j web3j, TransactionManager transactionManager, BigInteger gasPrice, BigInteger gasLimit, List data) {
String encodedConstructor = FunctionEncoder.encodeConstructor(Arrays.asList(new org.web3j.abi.datatypes.DynamicArray(
org.web3j.abi.Utils.typeMap(data, org.web3j.abi.datatypes.generated.Int32.class))));
return deployRemoteCall(Circle.class, web3j, transactionManager, gasPrice, gasLimit, BINARY, encodedConstructor);
}
public static Circle load(String contractAddress, Web3j web3j, Credentials credentials, BigInteger gasPrice, BigInteger gasLimit) {
return new Circle(contractAddress, web3j, credentials, gasPrice, gasLimit);
}
public static Circle load(String contractAddress, Web3j web3j, TransactionManager transactionManager, BigInteger gasPrice, BigInteger gasLimit) {
return new Circle(contractAddress, web3j, transactionManager, gasPrice, gasLimit);
}
}