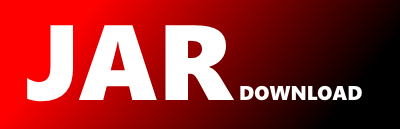
io.github.portlek.smartinventory.Icon Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of SmartInventory Show documentation
Show all versions of SmartInventory Show documentation
An API for creating unique inventories for Bukkit servers.
/*
* MIT License
*
* Copyright (c) 2020 Hasan Demirtaş
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*
*/
package io.github.portlek.smartinventory;
import io.github.portlek.smartinventory.event.abs.ClickEvent;
import io.github.portlek.smartinventory.event.abs.DragEvent;
import io.github.portlek.smartinventory.event.abs.IconEvent;
import io.github.portlek.smartinventory.event.abs.SmartEvent;
import io.github.portlek.smartinventory.icon.BasicIcon;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import java.util.function.Predicate;
import org.bukkit.Material;
import org.bukkit.inventory.ItemStack;
import org.jetbrains.annotations.NotNull;
/**
* a class that holds the {@link ItemStack} to put the given inventory.
*/
public interface Icon {
/**
* an empty {@link Icon} instance.
*/
Icon EMPTY = Icon.from(new ItemStack(Material.AIR));
/**
* creates a simple icon from the given {@link ItemStack}.
*
* @param item the item to create.
*
* @return a simple icon instance.
*/
@NotNull
static Icon from(@NotNull final ItemStack item) {
return new BasicIcon(item);
}
/**
* creates a simple icon from the given {@link ItemStack} with a {@link ClickEvent}.
*
* @param item the item to create.
* @param consumer the consumer to run.
* @param requirements the requirements to check.
*
* @return a simple icon instance.
*/
@SafeVarargs
@NotNull
static Icon click(@NotNull final ItemStack item, @NotNull final Consumer consumer,
@NotNull final Predicate... requirements) {
return Icon.from(item)
.whenClick(consumer, Arrays.asList(requirements));
}
/**
* creates a simple icon from the given {@link ItemStack} with a {@link DragEvent}.
*
* @param item the item to create.
* @param consumer the consumer to run.
* @param requirements the requirements to check.
*
* @return a simple icon instance.
*/
@SafeVarargs
@NotNull
static Icon drag(@NotNull final ItemStack item, @NotNull final Consumer consumer,
@NotNull final Predicate... requirements) {
return Icon.from(item)
.whenDrag(consumer, Arrays.asList(requirements));
}
/**
* creates a simple icon from the given {@link ItemStack} with {@link SmartEvent#cancel()} interaction.
*
* @param item the item to create.
*
* @return a simple icon instance.
*/
@NotNull
static Icon cancel(@NotNull final ItemStack item) {
return Icon.from(item)
.whenInteract(SmartEvent::cancel);
}
/**
* adds the given {@link IconEvent} to the icon's handles.
*
* @param consumer the consumer to add.
*
* @return {@code this}, for chained calls.
*/
@NotNull
default Icon whenInteract(@NotNull final Consumer consumer) {
return this.whenInteract(consumer, Collections.emptyList());
}
/**
* adds the given {@link IconEvent} with the requirements to the icon's handles.
*
* @param consumer the consumer to add.
* @param requirements the requirements to add.
*
* @return {@code this}, for chained calls.
*/
@NotNull
default Icon whenInteract(@NotNull final Consumer consumer,
@NotNull final List> requirements) {
return this.handle(IconEvent.class, consumer, requirements);
}
/**
* adds the given {@link DragEvent} to the icon's handles.
*
* @param consumer the consumer to add.
*
* @return {@code this}, for chained calls.
*/
@NotNull
default Icon whenDrag(@NotNull final Consumer consumer) {
return this.whenDrag(consumer, Collections.emptyList());
}
/**
* adds the given {@link DragEvent} with the requirements to the icon's handles.
*
* @param consumer the consumer to add.
* @param requirements the requirements to add.
*
* @return {@code this}, for chained calls.
*/
@NotNull
default Icon whenDrag(@NotNull final Consumer consumer,
@NotNull final List> requirements) {
return this.handle(DragEvent.class, consumer, requirements);
}
/**
* adds the given {@link ClickEvent} to the icon's handles.
*
* @param consumer the consumer to add.
*
* @return {@code this}, for chained calls.
*/
@NotNull
default Icon whenClick(@NotNull final Consumer consumer) {
return this.whenClick(consumer, Collections.emptyList());
}
/**
* adds the given {@link ClickEvent} with the requirements to the icon's handles.
*
* @param consumer the consumer to add.
* @param requirements the requirements to add.
*
* @return {@code this}, for chained calls.
*/
@NotNull
default Icon whenClick(@NotNull final Consumer consumer,
@NotNull final List> requirements) {
return this.handle(ClickEvent.class, consumer, requirements);
}
/**
* add the given event and requirements to the icon's handles.
*
* @param clazz the class to determine the type of the event.
* @param consumer the consumer to add.
* @param requirements the requirements to add.
* @param type of the event.
*
* @return {@code this}, for chained calls.
*/
@NotNull
default Icon handle(@NotNull final Class clazz, @NotNull final Consumer consumer,
@NotNull final List> requirements) {
return this.handle(Handle.from(clazz, consumer, requirements));
}
/**
* obtains the icon's {@link ItemStack}.
*
* @return the icon's item.
*/
@NotNull
ItemStack getItem();
/**
* calculates and returns the item of the icon.
* tests the {@code canSee} with the given contents, and if it returns {@code true},
* returns {@link #getItem()} else, returns the fallback.
*
* @param contents the contents to calculate.
*
* @return the calculated item.
*/
@NotNull
ItemStack calculateItem(@NotNull InventoryContents contents);
/**
* accepts the upcoming event for all of the handles.
*
* @param event the event to accept.
* @param type of the event.
*/
void accept(@NotNull T event);
/**
* adds the given handle into the icon's handle list.
*
* @param handle the handle to add.
* @param type of the event.
*
* @return {@code this}, for chained calls.
*/
@NotNull Icon handle(@NotNull Handle handle);
/**
* adds all the given handles into the icon's handle list.
*
* @param handles the handles to add.
*
* @return {@code this}, for chained calls.
*/
@NotNull
Icon handles(@NotNull Collection> handles);
/**
* sets the canSee value of the icon to the given predicate.
*
* @param predicate the predicate to set.
*
* @return {@code this}, for chained calls.
*/
@NotNull
Icon canSee(@NotNull Predicate predicate);
/**
* sets the canUse value of the icon to the given predicate.
*
* @param predicate the predicate to set.
*
* @return {@code this}, for chained calls.
*/
@NotNull
Icon canUse(@NotNull Predicate predicate);
/**
* sets the fallback item of the icon to the given item.
*
* @param fallback the fallback to set.
*
* @return {@code this}, for chained calls.
*/
@NotNull
Icon fallback(@NotNull ItemStack fallback);
/**
* sets the item of the icon to the given item.
*
* @param item the item to set.
*
* @return {@code this}, for chained calls.
*/
@NotNull
Icon item(@NotNull ItemStack item);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy