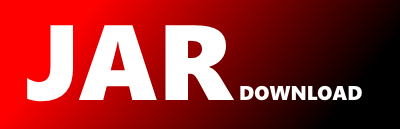
io.github.portlek.sfs.status.Topology Maven / Gradle / Ivy
package io.github.portlek.sfs.status;
import java.util.Collections;
import java.util.LinkedList;
import java.util.List;
public class Topology extends AbstractNode {
public List DataCenters;
public int Free;
public int Max;
public List Layouts;
private Topology.Stats stats;
public int getDataCenterCount() {
if (this.stats == null) {
this.computeStats();
}
if (this.stats == null) {
return 0;
}
return this.stats.dcCount;
}
public int getRackCount() {
if (this.stats == null) {
this.computeStats();
}
if (this.stats == null) {
return 0;
}
return this.stats.rackCount;
}
public int getDataNodeCount() {
if (this.stats == null) {
this.computeStats();
}
if (this.stats == null) {
return 0;
}
return this.stats.nodeCount;
}
public List getDataNodes() {
if (this.stats == null) {
this.computeStats();
}
if (this.stats == null) {
return Collections.emptyList();
}
return this.stats.nodeList;
}
@Override
public String toString() {
return "Topology{" +
"DataCenters=" + this.DataCenters +
", Free=" + this.Free +
", Max=" + this.Max +
", Layouts=" + this.Layouts +
", stats=" + this.stats +
'}';
}
private void computeStats() {
if (this.DataCenters == null) {
return;
}
this.stats = new Topology.Stats();
for (final DataCenter dc : this.DataCenters) {
this.stats.dcCount++;
if (dc.Racks == null) {
continue;
}
for (final Rack rack : dc.Racks) {
this.stats.rackCount++;
if (rack.DataNodes == null) {
continue;
}
this.stats.nodeCount += rack.DataNodes.size();
this.stats.nodeList.addAll(rack.DataNodes);
}
}
}
private class Stats {
int dcCount;
int rackCount;
int nodeCount;
List nodeList = new LinkedList<>();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy