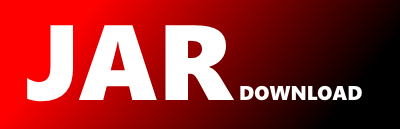
io.github.pustike.inject.impl.Binding Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pustike-inject Show documentation
Show all versions of pustike-inject Show documentation
A JSR-330 spec compliant dependency injection framework.
The newest version!
/*
* Copyright (C) 2016-2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.pustike.inject.impl;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import jakarta.inject.Provider;
import io.github.pustike.inject.BindingKey;
import io.github.pustike.inject.Scope;
/**
* Binding.
*/
final class Binding {
private final BindingKey bindingKey;
private final Provider provider;
private final Scope scope;
private final boolean multiBinder;
private Provider scopedProvider;
private boolean providerInjected;
Binding(BindingKey bindingKey, Provider provider, Scope scope) {
this.bindingKey = Objects.requireNonNull(bindingKey);
this.provider = Objects.requireNonNull(provider);
this.scope = Objects.requireNonNull(scope);
this.multiBinder = provider instanceof MultiBindingProvider;
}
Binding(BindingKey bindingKey, Provider provider, Scope scope, DefaultInjector injector) {
this(bindingKey, provider, scope);
this.postConfiguration(injector);
}
Binding(BindingKey bindingKey, List> bindingList, Scope scope, DefaultInjector injector) {
this(bindingKey, new MultiBindingProvider<>(bindingKey, bindingList), scope);
bindingList.forEach(binding -> binding.postConfiguration(injector));
}
boolean addBinding(Binding binding) {
return multiBinder && binding.multiBinder && ((MultiBindingProvider) provider)
.addBindings((MultiBindingProvider) binding.provider);
}
private void postConfiguration(DefaultInjector injector) {
if (provider instanceof InstanceProvider) {
((InstanceProvider>) provider).setInjector(injector);
this.providerInjected = true;// internal instanceProvider doesn't require any dependency injection
}
this.scopedProvider = scope.scope(bindingKey, () -> createInstance(injector));
}
void createIfEagerSingleton() {
if (multiBinder) {
((MultiBindingProvider>) provider).createIfEagerSingleton();
} else if (scope instanceof SingletonScope && scope.toString().equals(Scopes.EAGER_SINGLETON)) {
this.scopedProvider.get();
}
}
Object getInstance(BindingKey> targetKey) {
return multiBinder ? ((MultiBindingProvider>) provider).getInstance(targetKey) :
targetKey.isProviderKey() ? this.scopedProvider : this.scopedProvider.get();
}
private T createInstance(DefaultInjector injector) {
// inject dependencies into the provider instance as well!
if (!providerInjected) {
injector.injectMembers(provider);
providerInjected = true;
}
// get an instance from the provider
T newInstance = provider.get();
if (newInstance != null) {// inject members of the new instance
injector.injectMembers(bindingKey, newInstance);
}
return newInstance;
}
private static final class MultiBindingProvider implements Provider {
private final BindingKey bindingKey;
private final List> bindingList;
MultiBindingProvider(BindingKey bindingKey, List> bindingList) {
this.bindingKey = bindingKey;
this.bindingList = bindingList;
}
boolean addBindings(MultiBindingProvider provider) {
return bindingList.addAll(provider.bindingList);
}
void createIfEagerSingleton() {
bindingList.forEach(Binding::createIfEagerSingleton);
}
Collection> getInstance(BindingKey> targetKey) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy