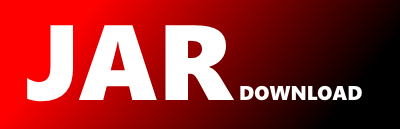
io.github.qudtlib.support.index.MultivaluePatriciaTrie Maven / Gradle / Ivy
package io.github.qudtlib.support.index;
import java.util.*;
import java.util.stream.Collectors;
import org.apache.commons.collections4.trie.PatriciaTrie;
public class MultivaluePatriciaTrie {
private final PatriciaTrie> delegate = new PatriciaTrie<>();
public MultivaluePatriciaTrie() {}
public void put(String key, V value) {
delegate.compute(
key,
(k, values) -> {
if (values == null) {
values = new HashSet<>();
}
values.add(value);
return values;
});
}
public Set getByPrefixMatch(String prefix) {
return delegate.prefixMap(prefix).values().stream()
.flatMap(Set::stream)
.collect(Collectors.toSet());
}
public Set get(String k) {
Set set = delegate.get(k);
if (set == null) {
return Set.of();
}
return set.stream().collect(Collectors.toUnmodifiableSet());
}
public void clear() {
delegate.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy