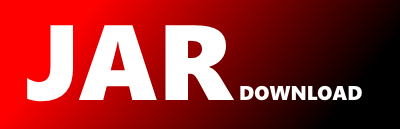
io.github.qudtlib.tools.contribute.support.tree.QuantityKindTree Maven / Gradle / Ivy
package io.github.qudtlib.tools.contribute.support.tree;
import static io.github.qudtlib.tools.contribute.support.tree.FormattingNodeVisitor.*;
import io.github.qudtlib.Qudt;
import io.github.qudtlib.model.QuantityKind;
import io.github.qudtlib.model.QudtNamespaces;
import io.github.qudtlib.model.Unit;
import java.util.*;
import java.util.stream.Collectors;
public class QuantityKindTree {
public static String formatQuantityKindForest(Collection quantityKinds) {
return formatSkosBroaderForest(makeSkosBroaderForest(quantityKinds));
}
public static String formatSkosBroaderForest(List> forest) {
StringBuilder sb = new StringBuilder();
NodeVisitor formattingVisitor =
new FormattingNodeVisitor(sb)
.nodeFormatDefault(
node -> {
StringBuilder ret = new StringBuilder();
ret.append(getShortName(node.getData()));
if (!node.getData().getExactMatches().isEmpty()) {
ret.append(" - exact matches: ")
.append(
node.getData().getExactMatches().stream()
.map(qk -> getShortName(qk))
.collect(Collectors.joining(",")));
}
return ret.toString();
});
for (Node tree : forest) {
TreeWalker.of(tree).walkDepthFirst(formattingVisitor);
}
return sb.toString();
}
public static List> makeSkosBroaderForest(
Collection quantityKinds) {
List> resultForest =
Node.forestOf(
quantityKinds,
(parentCandidate, childCandidate) ->
childCandidate.getBroaderQuantityKinds().contains(parentCandidate));
return resultForest;
}
public static List> makeCompleteSkosBroaderForestContaining(
Collection quantityKinds) {
Set transitiveHull = new HashSet<>(quantityKinds);
int hullSize = -1;
while (transitiveHull.size() > hullSize) {
hullSize = transitiveHull.size();
Set toAdd = new HashSet<>();
for (QuantityKind qk : transitiveHull) {
toAdd.addAll(qk.getBroaderQuantityKinds());
}
transitiveHull.addAll(toAdd);
}
Qudt.allQuantityKinds().stream()
.filter(
qk ->
qk.getBroaderQuantityKinds().stream()
.anyMatch(q -> transitiveHull.contains(q)))
.forEach(transitiveHull::add);
List> resultForest =
Node.forestOf(
transitiveHull,
(parentCandidate, childCandidate) ->
childCandidate.getBroaderQuantityKinds().contains(parentCandidate));
return resultForest;
}
public static List> addAssociatedUnitsToQuantityKindForest(
List> forest) {
List> qksWithUnits = new ArrayList<>();
for (Node node : forest) {
qksWithUnits.add(addUnits(node));
}
return qksWithUnits;
}
private static Node
© 2015 - 2025 Weber Informatics LLC | Privacy Policy