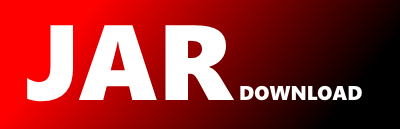
io.github.qudtlib.tools.contribute.support.tree.TreeWalker Maven / Gradle / Ivy
package io.github.qudtlib.tools.contribute.support.tree;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.Objects;
public class TreeWalker {
private Node root;
private Comparator> iterationOrderComparator = null;
public TreeWalker(Node root) {
Objects.requireNonNull(root);
this.root = root;
}
public TreeWalker sorted(Comparator> comparator) {
this.iterationOrderComparator = comparator;
return this;
}
public static TreeWalker of(Node root) {
return new TreeWalker(root);
}
public void walkDepthFirst(NodeVisitor visitor) {
walkDepthFirstInternal(root, visitor, 0, 0, 0);
}
private void walkDepthFirstInternal(
Node node, NodeVisitor visitor, int depth, int siblings, int orderInSiblings) {
NodeVisitor.NodeAndPositionInTree nodeAndPositionInTree =
new NodeVisitor.NodeAndPositionInTree<>(node, depth, siblings, orderInSiblings);
visitor.enter(nodeAndPositionInTree);
List> children = new ArrayList<>(node.getChildren());
if (this.iterationOrderComparator != null) {
children.sort(this.iterationOrderComparator);
}
for (int i = 0; i < children.size(); i++) {
walkDepthFirstInternal(children.get(i), visitor, depth + 1, children.size() - 1, i);
}
visitor.exit(nodeAndPositionInTree);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy