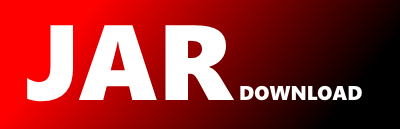
commonMain.com.github.quillraven.fleks.collection.bag.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Fleks-jvm Show documentation
Show all versions of Fleks-jvm Show documentation
A lightweight entity component system written in Kotlin.
The newest version!
package com.github.quillraven.fleks.collection
import kotlin.math.max
/**
* Creates a new [Bag] of the given [capacity] and type. Default capacity is 64.
*/
inline fun bag(capacity: Int = 64): Bag {
return Bag(arrayOfNulls(capacity))
}
/**
* A bag implementation in Kotlin containing only the necessary functions for Fleks.
*/
class Bag(
@PublishedApi
internal var values: Array
) {
var size: Int = 0
private set
val capacity: Int
get() = values.size
fun add(value: T) {
if (size == values.size) {
values = values.copyOf(max(1, size * 2))
}
values[size++] = value
}
operator fun set(index: Int, value: T) {
if (index >= values.size) {
values = values.copyOf(max(size * 2, index + 1))
}
size = max(size, index + 1)
values[index] = value
}
operator fun get(index: Int): T {
if (index < 0 || index >= size) throw IndexOutOfBoundsException("$index is not valid for bag of size $size")
return values[index] ?: throw NoSuchElementException("Bag has no value at index $index")
}
/**
* Returns the element at position [index] or null if there is no element or if [index] is out of bounds
*/
fun getOrNull(index: Int): T? = values.getOrNull(index)
fun hasNoValueAtIndex(index: Int): Boolean {
return index >= size || index < 0 || values[index] == null
}
fun hasValueAtIndex(index: Int): Boolean {
return index in 0.. Unit) {
for (i in 0 until size) {
values[i]?.let(action)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy