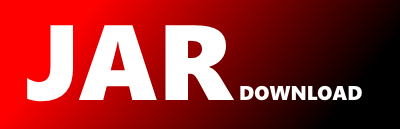
io.github.rcarlosdasilva.kits.net.http.HttpStatusCode Maven / Gradle / Ivy
package io.github.rcarlosdasilva.kits.net.http;
/**
* HTTP STATUS CODE
*
* @author Dean Zhao ([email protected])
*/
public class HttpStatusCode {
// 1xx Informational
/**
* {@code 100 Continue}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.2.1
*/
public static final int CONTINUE = 100;
/**
* {@code 101 Switching Protocols}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.2.2
*/
public static final int SWITCHING_PROTOCOLS = 101;
/**
* {@code 102 Processing}.
*
* @see WebDAV
*/
public static final int PROCESSING = 102;
/**
* {@code 103 Checkpoint}.
*
* @see A
* proposal for supporting resumable POST/PUT HTTP requests in
* HTTP/1.0
*/
public static final int CHECKPOINT = 103;
// 2xx Success
/**
* {@code 200 OK}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.3.1
*/
public static final int OK = 200;
/**
* {@code 201 Created}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.3.2
*/
public static final int CREATED = 201;
/**
* {@code 202 Accepted}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.3.3
*/
public static final int ACCEPTED = 202;
/**
* {@code 203 Non-Authoritative Information}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.3.4
*/
public static final int NON_AUTHORITATIVE_INFORMATION = 203;
/**
* {@code 204 No Content}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.3.5
*/
public static final int NO_CONTENT = 204;
/**
* {@code 205 Reset Content}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.3.6
*/
public static final int RESET_CONTENT = 205;
/**
* {@code 206 Partial Content}.
*
* @see HTTP/1.1:
* Range Requests, section 4.1
*/
public static final int PARTIAL_CONTENT = 206;
/**
* {@code 207 Multi-Status}.
*
* @see WebDAV
*/
public static final int MULTI_STATUS = 207;
/**
* {@code 208 Already Reported}.
*
* @see WebDAV
* Binding Extensions
*/
public static final int ALREADY_REPORTED = 208;
/**
* {@code 226 IM Used}.
*
* @see Delta
* encoding in HTTP
*/
public static final int IM_USED = 226;
// 3xx Redirection
/**
* {@code 300 Multiple Choices}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.4.1
*/
public static final int MULTIPLE_CHOICES = 300;
/**
* {@code 301 Moved Permanently}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.4.2
*/
public static final int MOVED_PERMANENTLY = 301;
/**
* {@code 302 Found}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.4.3
*/
public static final int FOUND = 302;
/**
* {@code 302 Moved Temporarily}.
*
* @see HTTP/1.0,
* section 9.3
* @deprecated In favor of {@link #FOUND} which will be returned from
* {@code HttpStatus.valueOf(302)}
*/
@Deprecated
public static final int MOVED_TEMPORARILY = 302;
/**
* {@code 303 See Other}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.4.4
*/
public static final int SEE_OTHER = 303;
/**
* {@code 304 Not Modified}.
*
* @see HTTP/1.1:
* Conditional Requests, section 4.1
*/
public static final int NOT_MODIFIED = 304;
/**
* {@code 305 Use Proxy}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.4.5
* @deprecated due to security concerns regarding in-band configuration of a
* proxy
*/
@Deprecated
public static final int USE_PROXY = 305;
/**
* {@code 307 Temporary Redirect}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.4.7
*/
public static final int TEMPORARY_REDIRECT = 307;
/**
* {@code 308 Permanent Redirect}.
*
* @see RFC 7238
*/
public static final int PERMANENT_REDIRECT = 308;
// --- 4xx Client Error ---
/**
* {@code 400 Bad Request}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.5.1
*/
public static final int BAD_REQUEST = 400;
/**
* {@code 401 Unauthorized}.
*
* @see HTTP/1.1:
* Authentication, section 3.1
*/
public static final int UNAUTHORIZED = 401;
/**
* {@code 402 Payment Required}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.5.2
*/
public static final int PAYMENT_REQUIRED = 402;
/**
* {@code 403 Forbidden}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.5.3
*/
public static final int FORBIDDEN = 403;
/**
* {@code 404 Not Found}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.5.4
*/
public static final int NOT_FOUND = 404;
/**
* {@code 405 Method Not Allowed}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.5.5
*/
public static final int METHOD_NOT_ALLOWED = 405;
/**
* {@code 406 Not Acceptable}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.5.6
*/
public static final int NOT_ACCEPTABLE = 406;
/**
* {@code 407 Proxy Authentication Required}.
*
* @see HTTP/1.1:
* Authentication, section 3.2
*/
public static final int PROXY_AUTHENTICATION_REQUIRED = 407;
/**
* {@code 408 Request Timeout}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.5.7
*/
public static final int REQUEST_TIMEOUT = 408;
/**
* {@code 409 Conflict}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.5.8
*/
public static final int CONFLICT = 409;
/**
* {@code 410 Gone}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.5.9
*/
public static final int GONE = 410;
/**
* {@code 411 Length Required}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.5.10
*/
public static final int LENGTH_REQUIRED = 411;
/**
* {@code 412 Precondition failed}.
*
* @see HTTP/1.1:
* Conditional Requests, section 4.2
*/
public static final int PRECONDITION_FAILED = 412;
/**
* {@code 413 Payload Too Large}.
*
* @since 4.1
* @see HTTP/1.1:
* Semantics and Content, section 6.5.11
*/
public static final int PAYLOAD_TOO_LARGE = 413;
/**
* {@code 413 Request Entity Too Large}.
*
* @see HTTP/1.1,
* section 10.4.14
* @deprecated In favor of {@link #PAYLOAD_TOO_LARGE} which will be returned
* from {@code HttpStatus.valueOf(413)}
*/
@Deprecated
public static final int REQUEST_ENTITY_TOO_LARGE = 413;
/**
* {@code 414 URI Too Long}.
*
* @since 4.1
* @see HTTP/1.1:
* Semantics and Content, section 6.5.12
*/
public static final int URI_TOO_LONG = 414;
/**
* {@code 414 Request-URI Too Long}.
*
* @see HTTP/1.1,
* section 10.4.15
* @deprecated In favor of {@link #URI_TOO_LONG} which will be returned from
* {@code HttpStatus.valueOf(414)}
*/
@Deprecated
public static final int REQUEST_URI_TOO_LONG = 414;
/**
* {@code 415 Unsupported Media Type}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.5.13
*/
public static final int UNSUPPORTED_MEDIA_TYPE = 415;
/**
* {@code 416 Requested Range Not Satisfiable}.
*
* @see HTTP/1.1:
* Range Requests, section 4.4
*/
public static final int REQUESTED_RANGE_NOT_SATISFIABLE = 416;
/**
* {@code 417 Expectation Failed}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.5.14
*/
public static final int EXPECTATION_FAILED = 417;
/**
* {@code 418 I'm a teapot}.
*
* @see HTCPCP/1.0
*/
public static final int I_AM_A_TEAPOT = 418;
/**
* @deprecated See WebDAV
* Draft Changes
*/
@Deprecated
public static final int INSUFFICIENT_SPACE_ON_RESOURCE = 419;
/**
* @deprecated See WebDAV
* Draft Changes
*/
@Deprecated
public static final int METHOD_FAILURE = 420;
/**
* @deprecated See WebDAV
* Draft Changes
*/
@Deprecated
public static final int DESTINATION_LOCKED = 421;
/**
* {@code 422 Unprocessable Entity}.
*
* @see WebDAV
*/
public static final int UNPROCESSABLE_ENTITY = 422;
/**
* {@code 423 Locked}.
*
* @see WebDAV
*/
public static final int LOCKED = 423;
/**
* {@code 424 Failed Dependency}.
*
* @see WebDAV
*/
public static final int FAILED_DEPENDENCY = 424;
/**
* {@code 426 Upgrade Required}.
*
* @see Upgrading to
* TLS Within HTTP/1.1
*/
public static final int UPGRADE_REQUIRED = 426;
/**
* {@code 428 Precondition Required}.
*
* @see Additional HTTP
* Status Codes
*/
public static final int PRECONDITION_REQUIRED = 428;
/**
* {@code 429 Too Many Requests}.
*
* @see Additional HTTP
* Status Codes
*/
public static final int TOO_MANY_REQUESTS = 429;
/**
* {@code 431 Request Header Fields Too Large}.
*
* @see Additional HTTP
* Status Codes
*/
public static final int REQUEST_HEADER_FIELDS_TOO_LARGE = 431;
// --- 5xx Server Error ---
/**
* {@code 500 Internal Server Error}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.6.1
*/
public static final int INTERNAL_SERVER_ERROR = 500;
/**
* {@code 501 Not Implemented}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.6.2
*/
public static final int NOT_IMPLEMENTED = 501;
/**
* {@code 502 Bad Gateway}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.6.3
*/
public static final int BAD_GATEWAY = 502;
/**
* {@code 503 Service Unavailable}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.6.4
*/
public static final int SERVICE_UNAVAILABLE = 503;
/**
* {@code 504 Gateway Timeout}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.6.5
*/
public static final int GATEWAY_TIMEOUT = 504;
/**
* {@code 505 HTTP Version Not Supported}.
*
* @see HTTP/1.1:
* Semantics and Content, section 6.6.6
*/
public static final int HTTP_VERSION_NOT_SUPPORTED = 505;
/**
* {@code 506 Variant Also Negotiates}
*
* @see Transparent
* Content Negotiation
*/
public static final int VARIANT_ALSO_NEGOTIATES = 506;
/**
* {@code 507 Insufficient Storage}
*
* @see WebDAV
*/
public static final int INSUFFICIENT_STORAGE = 507;
/**
* {@code 508 Loop Detected}
*
* @see WebDAV
* Binding Extensions
*/
public static final int LOOP_DETECTED = 508;
/**
* {@code 509 Bandwidth Limit Exceeded}.
*/
public static final int BANDWIDTH_LIMIT_EXCEEDED = 509;
/**
* {@code 510 Not Extended}
*
* @see HTTP Extension
* Framework
*/
public static final int NOT_EXTENDED = 510;
/**
* {@code 511 Network Authentication Required}.
*
* @see Additional HTTP
* Status Codes
*/
public static final int NETWORK_AUTHENTICATION_REQUIRED = 511;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy