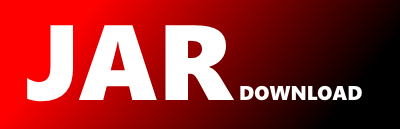
com.reandroid.arsc.array.CompoundItemArray Maven / Gradle / Ivy
/*
* Copyright (C) 2022 github.com/REAndroid
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.reandroid.arsc.array;
import com.reandroid.arsc.base.BlockArray;
import com.reandroid.arsc.value.*;
import com.reandroid.json.JSONConvert;
import com.reandroid.json.JSONArray;
import java.util.Comparator;
public abstract class CompoundItemArray
extends BlockArray implements JSONConvert, Comparator {
public CompoundItemArray(){
super();
}
public void sort(){
super.sort(this);
updateCountToHeader();
}
@Override
public T createNext(){
T resValueMap = super.createNext();
updateCountToHeader();
return resValueMap;
}
private void updateCountToHeader(){
EntryHeaderMap headerMap = getEntryHeaderMap();
headerMap.setValuesCount(childesCount());
}
private EntryHeaderMap getEntryHeaderMap(){
ResTableMapEntry mapEntry = getParent(ResTableMapEntry.class);
assert mapEntry != null;
return mapEntry.getHeader();
}
public AttributeDataFormat[] getFormats(){
ResValueMap formatsMap = getByType(AttributeType.FORMATS);
if(formatsMap != null){
return AttributeDataFormat.decodeValueTypes(
formatsMap.getData() & 0xff);
}
return null;
}
public boolean containsType(AttributeType attributeType){
for(T valueMap : getChildes()){
if(attributeType == valueMap.getAttributeType()){
return true;
}
}
return false;
}
public T getOrCreateType(AttributeType attributeType){
if(attributeType == null){
return null;
}
T valueMap = getByType(attributeType);
if(valueMap == null){
valueMap = createNext();
valueMap.setAttributeType(attributeType);
}
return valueMap;
}
public T getByType(AttributeType attributeType){
if(attributeType == null){
return null;
}
for(T valueMap : getChildes()){
if(attributeType == valueMap.getAttributeType()){
return valueMap;
}
}
return null;
}
public T getByName(int name){
for(T resValueMap : getChildes()){
if(resValueMap != null && name == resValueMap.getName()){
return resValueMap;
}
}
return null;
}
@Override
protected void onRefreshed() {
}
public void onRemoved(){
for(T resValueMap : getChildes()){
resValueMap.onRemoved();
}
}
@Override
public void clearChildes(){
this.onRemoved();
super.clearChildes();
}
@Override
public JSONArray toJson() {
JSONArray jsonArray=new JSONArray();
if(isNull()){
return jsonArray;
}
T[] childes = getChildes();
for(int i = 0; i < childes.length; i++){
jsonArray.put(i, childes[i].toJson());
}
return jsonArray;
}
@Override
public void fromJson(JSONArray json){
clearChildes();
if(json==null){
return;
}
int count=json.length();
ensureSize(count);
for(int i=0;i mapArray){
if(mapArray == null || mapArray == this){
return;
}
clearChildes();
int count = mapArray.childesCount();
ensureSize(count);
for(int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy