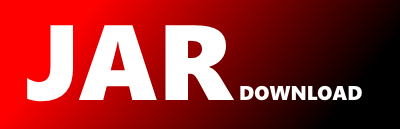
com.reandroid.utils.collection.EmptyList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ARSCLib Show documentation
Show all versions of ARSCLib Show documentation
Android binary resources read/write library
/*
* Copyright (C) 2022 github.com/REAndroid
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.reandroid.utils.collection;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
public class EmptyList implements List, EmptyItem {
@Override
public int size() {
return 0;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public boolean contains(Object o) {
return false;
}
@Override
public Iterator iterator() {
return EmptyIterator.of();
}
@Override
public Object[] toArray() {
return new Object[0];
}
@Override
public T1[] toArray(T1[] t1s) {
return t1s;
}
@Override
public boolean add(T t) {
throw new IllegalArgumentException("Empty list");
}
@Override
public boolean remove(Object o) {
return false;
}
@Override
public boolean containsAll(Collection> collection) {
return false;
}
@Override
public boolean addAll(Collection extends T> collection) {
throw new IllegalArgumentException("Empty list");
}
@Override
public boolean addAll(int i, Collection extends T> collection) {
throw new IllegalArgumentException("Empty list");
}
@Override
public boolean removeAll(Collection> collection) {
return false;
}
@Override
public boolean retainAll(Collection> collection) {
throw new IllegalArgumentException("Empty list");
}
@Override
public void clear() {
}
@Override
public T get(int i) {
throw new IllegalArgumentException("Empty list");
}
@Override
public T set(int i, T t) {
throw new IllegalArgumentException("Empty list");
}
@Override
public void add(int i, T t) {
throw new IllegalArgumentException("Empty list");
}
@Override
public T remove(int i) {
throw new IllegalArgumentException("Empty list");
}
@Override
public int indexOf(Object o) {
return -1;
}
@Override
public int lastIndexOf(Object o) {
return -1;
}
@Override
public ListIterator listIterator() {
return EmptyIterator.of();
}
@Override
public ListIterator listIterator(int i) {
return EmptyIterator.of();
}
@Override
public List subList(int i, int i1) {
throw new IllegalArgumentException("Empty list");
}
public static EmptyList of(){
return (EmptyList) INS;
}
private static final EmptyList> INS = new EmptyList<>();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy