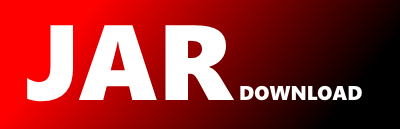
play.i18n.Messages Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of framework Show documentation
Show all versions of framework Show documentation
RePlay is a fork of the Play1 framework, created by Codeborne.
package play.i18n;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import play.Play;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.util.*;
import java.util.function.Function;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* I18n Helper
*
* translation are defined as properties in /conf/messages.locale files with locale being the i18n country code
* fr, en, fr_FR
*
*
* # /conf/messages.fr
* hello=Bonjour, %s !
*
*
* {@code
* Messages.get( "hello", "World"); // => "Bonjour, World !"
* }
*
*/
public class Messages {
private static final Logger logger = LoggerFactory.getLogger(Messages.class);
private static final Object[] NO_ARGS = new Object[] { null };
public static Properties defaults = new Properties();
public static Map locales = new HashMap<>();
private static final Pattern recursive = Pattern.compile("&\\{(.*?)}");
/**
* Given a message code, translate it using current locale. If there is no message in the current locale for the
* given key, the key is returned.
*
* @param key
* the message code
* @param args
* optional message format arguments
* @return translated message
*/
public static String get(Object key, Object... args) {
return getMessage(Lang.get(), key, args);
}
/**
* Return several messages for a locale
*
* @param locale
* the locale code, e.g. fr, fr_FR
* @param keys
* the keys to get messages from. Wildcards can be used at the end: {'title', 'login.*'}
* @return messages as a {@link Properties java.util.Properties}
*/
public static Properties find(String locale, Set keys) {
Properties result = new Properties();
Properties all = all(locale);
// Expand the set for wildcards
Set wildcards = new HashSet<>();
for (String key : keys) {
if (key.endsWith("*"))
wildcards.add(key);
}
for (String key : wildcards) {
keys.remove(key);
String start = key.substring(0, key.length() - 1);
for (Object key2 : all.keySet()) {
if (((String) key2).startsWith(start)) {
keys.add((String) key2);
}
}
}
// Build the result
for (Object key : all.keySet()) {
if (keys.contains(key)) {
result.put(key, all.get(key));
}
}
return result;
}
public static String getMessage(String locale, Object key, Object... args) {
return getMessage(locale, (k) -> k.toString(), key, args);
}
public static String getMessage(@Nonnull String locale, @Nonnull Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy