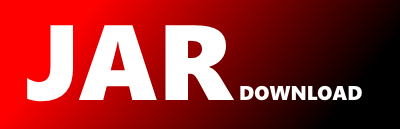
io.github.resilience4j.core.CallableUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resilience4j-core Show documentation
Show all versions of resilience4j-core Show documentation
Resilience4j is a lightweight, easy-to-use fault tolerance library designed for Java8 and functional programming
package io.github.resilience4j.core;
import java.util.concurrent.Callable;
import java.util.function.BiFunction;
import java.util.function.Function;
public class CallableUtils {
private CallableUtils(){}
/**
* Returns a composed function that first applies the Callable and then applies
* the resultHandler.
*
* @param return type of callable
* @param return type of handler
* @param resultHandler the function applied after callable
* @return a function composed of supplier and resultHandler
*/
public static Callable andThen(Callable callable, Function resultHandler){
return () -> resultHandler.apply(callable.call());
}
/**
* Returns a composed function that first applies the Callable and then applies
* {@linkplain BiFunction} {@code after} to the result.
*
* @param return type of callable
* @param return type of handler
* @param handler the function applied after callable
* @return a function composed of supplier and handler
*/
public static Callable andThen(Callable callable, BiFunction handler){
return () -> {
try{
T result = callable.call();
return handler.apply(result, null);
}catch (Exception exception){
return handler.apply(null, exception);
}
};
}
/**
* Returns a composed function that first applies the Callable and then applies
* either the resultHandler or exceptionHandler.
*
* @param return type of callable
* @param return type of resultHandler and exceptionHandler
* @param resultHandler the function applied after callable was successful
* @param exceptionHandler the function applied after callable has failed
* @return a function composed of supplier and handler
*/
public static Callable andThen(Callable callable, Function resultHandler, Function exceptionHandler){
return () -> {
try{
T result = callable.call();
return resultHandler.apply(result);
}catch (Exception exception){
return exceptionHandler.apply(exception);
}
};
}
/**
* Returns a composed function that first executes the Callable and optionally recovers from an exception.
*
* @param return type of after
* @param exceptionHandler the exception handler
* @return a function composed of callable and exceptionHandler
*/
public static Callable recover(Callable callable, Function exceptionHandler){
return () -> {
try{
return callable.call();
}catch (Exception exception){
return exceptionHandler.apply(exception);
}
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy