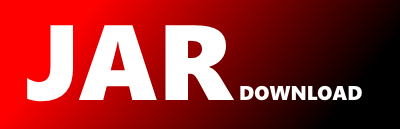
io.github.resilience4j.core.SupplierUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resilience4j-core Show documentation
Show all versions of resilience4j-core Show documentation
Resilience4j is a lightweight, easy-to-use fault tolerance library designed for Java8 and functional programming
package io.github.resilience4j.core;
import java.util.function.BiFunction;
import java.util.function.Function;
import java.util.function.Supplier;
public class SupplierUtils {
private SupplierUtils(){}
/**
* Returns a composed function that first applies the Supplier and then applies
* the resultHandler.
*
* @param return type of callable
* @param return type of handler
* @param resultHandler the function applied after supplier
* @return a function composed of supplier and resultHandler
*/
public static Supplier andThen(Supplier supplier, Function resultHandler){
return () -> resultHandler.apply(supplier.get());
}
/**
* Returns a composed function that first applies the Supplier and then applies
* {@linkplain BiFunction} {@code after} to the result.
*
* @param return type of after
* @param handler the function applied after supplier
* @return a function composed of supplier and handler
*/
public static Supplier andThen(Supplier supplier, BiFunction handler){
return () -> {
try{
T result = supplier.get();
return handler.apply(result, null);
}catch (Exception exception){
return handler.apply(null, exception);
}
};
}
/**
* Returns a composed function that first executes the Supplier and optionally recovers from an exception.
*
* @param return type of after
* @param exceptionHandler the exception handler
* @return a function composed of supplier and exceptionHandler
*/
public static Supplier recover(Supplier supplier, Function exceptionHandler){
return () -> {
try{
return supplier.get();
}catch (Exception exception){
return exceptionHandler.apply(exception);
}
};
}
/**
* Returns a composed function that first applies the Supplier and then applies
* either the resultHandler or exceptionHandler.
*
* @param return type of after
* @param resultHandler the function applied after Supplier was successful
* @param exceptionHandler the function applied after Supplier has failed
* @return a function composed of supplier and handler
*/
public static Supplier andThen(Supplier supplier, Function resultHandler, Function exceptionHandler){
return () -> {
try{
T result = supplier.get();
return resultHandler.apply(result);
}catch (Exception exception){
return exceptionHandler.apply(exception);
}
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy