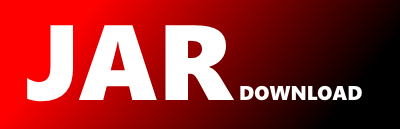
io.github.resilience4j.core.SupplierUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resilience4j-core Show documentation
Show all versions of resilience4j-core Show documentation
Resilience4j is a lightweight, easy-to-use fault tolerance library designed for Java8 and functional programming
/*
*
* Copyright 2020: Robert Winkler
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*
*/
package io.github.resilience4j.core;
import java.util.List;
import java.util.function.*;
public class SupplierUtils {
private SupplierUtils() {
}
/**
* Returns a composed Supplier that first applies the Supplier and then applies the
* resultHandler.
*
* @param return type of callable
* @param return type of handler
* @param supplier the supplier
* @param resultHandler the function applied after supplier
* @return a function composed of supplier and resultHandler
*/
public static Supplier andThen(Supplier supplier, Function resultHandler) {
return () -> resultHandler.apply(supplier.get());
}
/**
* Returns a composed Supplier that first applies the Supplier and then applies {@linkplain
* BiFunction} {@code after} to the result.
*
* @param return type of after
* @param supplier the supplier
* @param handler the function applied after supplier
* @return a function composed of supplier and handler
*/
public static Supplier andThen(Supplier supplier,
BiFunction handler) {
return () -> {
try {
T result = supplier.get();
return handler.apply(result, null);
} catch (Exception exception) {
return handler.apply(null, exception);
}
};
}
/**
* Returns a composed Supplier that first executes the Supplier and optionally recovers from a specific result.
*
* @param return type of after
* @param supplier the supplier
* @param resultPredicate the result predicate
* @param resultHandler the result handler
* @return a function composed of supplier and exceptionHandler
*/
public static Supplier recover(Supplier supplier,
Predicate resultPredicate, UnaryOperator resultHandler) {
return () -> {
T result = supplier.get();
if(resultPredicate.test(result)){
return resultHandler.apply(result);
}
return result;
};
}
/**
* Returns a composed function that first executes the Supplier and optionally recovers from an
* exception.
*
* @param return type of after
* @param supplier the supplier
* @param exceptionHandler the exception handler
* @return a function composed of supplier and exceptionHandler
*/
public static Supplier recover(Supplier supplier,
Function exceptionHandler) {
return () -> {
try {
return supplier.get();
} catch (Exception exception) {
return exceptionHandler.apply(exception);
}
};
}
/**
* Returns a composed function that first executes the Supplier and optionally recovers from an
* exception.
*
* @param return type of after
* @param supplier the supplier which should be recovered from a certain exception
* @param exceptionTypes the specific exception types that should be recovered
* @param exceptionHandler the exception handler
* @return a function composed of supplier and exceptionHandler
*/
public static Supplier recover(Supplier supplier,
List> exceptionTypes,
Function exceptionHandler) {
return () -> {
try {
return supplier.get();
} catch (Exception exception) {
if(exceptionTypes.stream().anyMatch(exceptionType -> exceptionType.isAssignableFrom(exception.getClass()))){
return exceptionHandler.apply(exception);
}else{
throw exception;
}
}
};
}
/**
* Returns a composed function that first executes the Supplier and optionally recovers from an
* exception.
*
* @param return type of after
* @param supplier the supplier which should be recovered from a certain exception
* @param exceptionType the specific exception type that should be recovered
* @param exceptionHandler the exception handler
* @return a function composed of supplier and exceptionHandler
*/
public static Supplier recover(Supplier supplier,
Class exceptionType,
Function exceptionHandler) {
return () -> {
try {
return supplier.get();
} catch (RuntimeException exception) {
if(exceptionType.isAssignableFrom(exception.getClass())) {
return exceptionHandler.apply(exception);
}else{
throw exception;
}
}
};
}
/**
* Returns a composed function that first applies the Supplier and then applies either the
* resultHandler or exceptionHandler.
*
* @param return type of after
* @param supplier the supplier which should be recovered from a certain exception
* @param resultHandler the function applied after Supplier was successful
* @param exceptionHandler the function applied after Supplier has failed
* @return a function composed of supplier and handler
*/
public static Supplier andThen(Supplier supplier, Function resultHandler,
Function exceptionHandler) {
return () -> {
try {
T result = supplier.get();
return resultHandler.apply(result);
} catch (Exception exception) {
return exceptionHandler.apply(exception);
}
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy