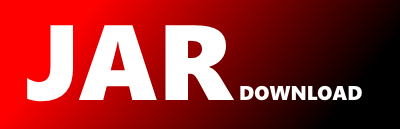
io.github.resilience4j.feign.FallbackDecorator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resilience4j-feign Show documentation
Show all versions of resilience4j-feign Show documentation
Resilience4j is a lightweight, easy-to-use fault tolerance library designed for Java8 and functional programming
/*
*
* Copyright 2018
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*
*
*/
package io.github.resilience4j.feign;
import static java.util.Objects.requireNonNull;
import java.lang.reflect.Method;
import java.util.function.Predicate;
import feign.InvocationHandlerFactory.MethodHandler;
import feign.Target;
import io.vavr.CheckedFunction1;
/**
* Decorator that calls a fallback in the case that an exception is thrown.
*/
class FallbackDecorator implements FeignDecorator {
private final T fallback;
private Predicate filter;
/**
* Creates a fallback that will be called for every {@link Exception}.
*/
public FallbackDecorator(T fallback) {
this(fallback, ex -> true);
}
/**
* Creates a fallback that will only be called for the specified {@link Exception}.
*/
public FallbackDecorator(T fallback, Class extends Exception> filter) {
this(fallback, filter::isInstance);
requireNonNull(filter, "Filter cannot be null!");
}
/**
* Creates a fallback that will only be called if the specified {@link Predicate} returns
* true
.
*/
public FallbackDecorator(T fallback, Predicate filter) {
this.fallback = requireNonNull(fallback, "Fallback cannot be null!");
this.filter = requireNonNull(filter, "Filter cannot be null!");
}
/**
* Calls the fallback if the invocationCall throws an {@link Exception}.
*
* @throws IllegalArgumentException if the fallback object does not have a corresponding
* fallback method.
*/
@Override
public CheckedFunction1
© 2015 - 2024 Weber Informatics LLC | Privacy Policy