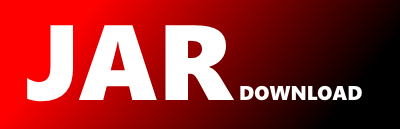
io.github.resilience4j.feign.FeignDecorators Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resilience4j-feign Show documentation
Show all versions of resilience4j-feign Show documentation
Resilience4j is a lightweight, easy-to-use fault tolerance library designed for Java8 and functional programming
/*
*
* Copyright 2018
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*
*
*/
package io.github.resilience4j.feign;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Predicate;
import feign.InvocationHandlerFactory.MethodHandler;
import feign.Target;
import io.github.resilience4j.circuitbreaker.CircuitBreaker;
import io.github.resilience4j.ratelimiter.RateLimiter;
import io.vavr.CheckedFunction1;
/**
* Builder to help build stacked decorators.
*
*
* {
* @code
* CircuitBreaker circuitBreaker = CircuitBreaker.ofDefaults("backendName");
* RateLimiter rateLimiter = RateLimiter.ofDefaults("backendName");
* FeignDecorators decorators = FeignDecorators.builder()
* .withCircuitBreaker(circuitBreaker)
* .withRateLimiter(rateLimiter)
* .build();
* MyService myService = Resilience4jFeign.builder(decorators).target(MyService.class, "http://localhost:8080/");
* }
*
*
* The order in which decorators are applied correspond to the order in which they are declared. For
* example, calling {@link FeignDecorators.Builder#withFallback(Object)} before
* {@link FeignDecorators.Builder#withCircuitBreaker(CircuitBreaker)} would mean that the fallback
* is called when the HTTP request fails, but would no longer be reachable if the CircuitBreaker
* were open. However, reversing the order would mean that the fallback is called both when the HTTP
* request fails and when the CircuitBreaker is open.
* So be wary of this when designing your "resilience" strategy.
*/
public class FeignDecorators implements FeignDecorator {
private final List decorators;
private FeignDecorators(List decorators) {
this.decorators = decorators;
}
@Override
public CheckedFunction1
© 2015 - 2024 Weber Informatics LLC | Privacy Policy