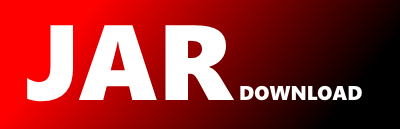
io.github.resilience4j.kotlin.timelimiter.TimeLimiter.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resilience4j-kotlin Show documentation
Show all versions of resilience4j-kotlin Show documentation
Resilience4j is a lightweight, easy-to-use fault tolerance library designed for Java8 and functional programming
/*
*
* Copyright 2019: Brad Newman
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*
*/
package io.github.resilience4j.kotlin.timelimiter
import io.github.resilience4j.kotlin.isCancellation
import io.github.resilience4j.timelimiter.TimeLimiter
import kotlinx.coroutines.TimeoutCancellationException
import kotlinx.coroutines.withTimeout
import java.util.concurrent.TimeoutException
import kotlin.coroutines.coroutineContext
/**
* Decorates and executes the given suspend function [block].
*
* This is a wrapper for [withTimeout], reading the timeout from the receiver's
* [timeLimiterConfig][TimeLimiter.getTimeLimiterConfig]. Specifically, this means:
*
* 1. On timeout, a [TimeoutException] is raised as with methods for non-suspending functions. It's derived from
* [java.util.concurrent.CancellationException] to ensure the exception is registered properly in events, and
* a [TimeLimiter] can be used safely when using multiple decorators.
* 1. When a timeout occurs, the coroutine is cancelled, rather than the thread being interrupted as with methods for
* non-suspending functions.
* 1. After the timeout, the given block can only be stopped at a cancellable suspending function call.
* 1. The `cancelRunningFuture` configuration setting is ignored - on timeout, the suspend function is always cancelled
* even if the `cancelRunningFuture` is set to `false`.
*/
suspend fun TimeLimiter.executeSuspendFunction(block: suspend () -> T): T =
try {
withTimeout(timeLimiterConfig.timeoutDuration.toMillis()) {
block().also { onSuccess() }
}
} catch (t: Throwable) {
if (isCancellation(coroutineContext, t)) {
val timeoutException = TimeLimiter.createdTimeoutExceptionWithName(name, t)
onError(timeoutException)
throw timeoutException
}
onError(t)
throw t
}
/**
* Decorates the given suspend function [block] and returns it.
*
* This uses [withTimeout], reading the timeout from the receiver's
* [timeLimiterConfig][TimeLimiter.getTimeLimiterConfig]. Specifically, this means:
*
* 1. On timeout, a [TimeoutCancellationException] is raised, rather than a TimeoutException as with methods for
* non-suspending functions.
* 1. When a timeout occurs, the coroutine is cancelled, rather than the thread being interrupted as with methods for
* non-suspending functions.
* 1. After the timeout, the given block can only be stopped at a cancellable suspending function call.
* 1. The `cancelRunningFuture` configuration setting is ignored - on timeout, the suspend function is always cancelled
* even if the `cancelRunningFuture` is set to `false`.
*/
fun TimeLimiter.decorateSuspendFunction(block: suspend () -> T): suspend () -> T = {
executeSuspendFunction(block)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy