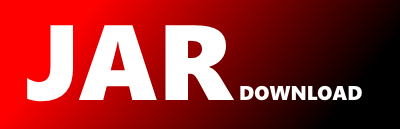
io.github.resilience4j.micronaut.bulkhead.ThreadPoolBulkheadFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resilience4j-micronaut Show documentation
Show all versions of resilience4j-micronaut Show documentation
Resilience4j is a lightweight, easy-to-use fault tolerance library designed for Java8 and functional programming
/*
* Copyright 2020 Michael Pollind
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.resilience4j.micronaut.bulkhead;
import io.github.resilience4j.bulkhead.ThreadPoolBulkhead;
import io.github.resilience4j.bulkhead.ThreadPoolBulkheadConfig;
import io.github.resilience4j.bulkhead.ThreadPoolBulkheadRegistry;
import io.github.resilience4j.bulkhead.event.BulkheadEvent;
import io.github.resilience4j.common.CompositeCustomizer;
import io.github.resilience4j.common.bulkhead.configuration.CommonThreadPoolBulkheadConfigurationProperties;
import io.github.resilience4j.common.bulkhead.configuration.ThreadPoolBulkheadConfigCustomizer;
import io.github.resilience4j.consumer.DefaultEventConsumerRegistry;
import io.github.resilience4j.consumer.EventConsumerRegistry;
import io.github.resilience4j.core.registry.CompositeRegistryEventConsumer;
import io.github.resilience4j.core.registry.RegistryEventConsumer;
import io.micronaut.context.annotation.Bean;
import io.micronaut.context.annotation.Factory;
import io.micronaut.context.annotation.Primary;
import io.micronaut.context.annotation.Requires;
import io.micronaut.core.util.StringUtils;
import jakarta.inject.Singleton;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.stream.Collectors;
import static java.util.Optional.ofNullable;
@Factory
@Requires(property = "resilience4j.thread-pool-bulkhead.enabled", value = StringUtils.TRUE, defaultValue = StringUtils.FALSE)
public class ThreadPoolBulkheadFactory {
@Bean
@ThreadPoolBulkheadQualifier
public CompositeCustomizer compositeThreadPoolBulkheadCustomizer(
List customizers) {
return new CompositeCustomizer<>(customizers);
}
@Singleton
@Requires(beans = CommonThreadPoolBulkheadConfigurationProperties.class)
public ThreadPoolBulkheadRegistry threadPoolBulkheadRegistry(
CommonThreadPoolBulkheadConfigurationProperties bulkheadConfigurationProperties,
@ThreadPoolBulkheadQualifier EventConsumerRegistry bulkheadEventConsumerRegistry,
@ThreadPoolBulkheadQualifier RegistryEventConsumer threadPoolBulkheadRegistryEventConsumer,
@ThreadPoolBulkheadQualifier CompositeCustomizer compositeThreadPoolBulkheadCustomizer) {
ThreadPoolBulkheadRegistry bulkheadRegistry = createBulkheadRegistry(
bulkheadConfigurationProperties, threadPoolBulkheadRegistryEventConsumer,
compositeThreadPoolBulkheadCustomizer);
registerEventConsumer(bulkheadRegistry, bulkheadEventConsumerRegistry,
bulkheadConfigurationProperties);
bulkheadConfigurationProperties.getBackends().forEach((name, properties) -> bulkheadRegistry
.bulkhead(name, bulkheadConfigurationProperties
.createThreadPoolBulkheadConfig(name, compositeThreadPoolBulkheadCustomizer)));
return bulkheadRegistry;
}
@Bean
@Primary
@ThreadPoolBulkheadQualifier
public RegistryEventConsumer threadPoolBulkheadRegistryEventConsumer(
Optional>> optionalRegistryEventConsumers) {
return new CompositeRegistryEventConsumer<>(
optionalRegistryEventConsumers.orElseGet(ArrayList::new));
}
@Bean
@ThreadPoolBulkheadQualifier
public EventConsumerRegistry threadPoolBulkheadEventsConsumerRegistry() {
return new DefaultEventConsumerRegistry<>();
}
/**
* Initializes a bulkhead registry.
*
* @param threadPoolBulkheadConfigurationProperties The bulkhead configuration properties.
* @param compositeThreadPoolBulkheadCustomizer the delegate of customizers
* @return a ThreadPoolBulkheadRegistry
*/
private ThreadPoolBulkheadRegistry createBulkheadRegistry(
CommonThreadPoolBulkheadConfigurationProperties threadPoolBulkheadConfigurationProperties,
RegistryEventConsumer threadPoolBulkheadRegistryEventConsumer,
CompositeCustomizer compositeThreadPoolBulkheadCustomizer) {
Map configs = threadPoolBulkheadConfigurationProperties
.getConfigs()
.entrySet()
.stream()
.collect(Collectors.toMap(Map.Entry::getKey,
entry -> threadPoolBulkheadConfigurationProperties
.createThreadPoolBulkheadConfig(entry.getValue(),
compositeThreadPoolBulkheadCustomizer, entry.getKey())));
return ThreadPoolBulkheadRegistry.of(configs, threadPoolBulkheadRegistryEventConsumer, threadPoolBulkheadConfigurationProperties.getTags());
}
/**
* Registers the post creation consumer function that registers the consumer events to the
* bulkheads.
*
* @param bulkheadRegistry The BulkHead registry.
* @param eventConsumerRegistry The event consumer registry.
*/
private void registerEventConsumer(ThreadPoolBulkheadRegistry bulkheadRegistry,
EventConsumerRegistry eventConsumerRegistry,
CommonThreadPoolBulkheadConfigurationProperties bulkheadConfigurationProperties) {
bulkheadRegistry.getEventPublisher().onEntryAdded(
event -> registerEventConsumer(eventConsumerRegistry, event.getAddedEntry(),
bulkheadConfigurationProperties));
}
private void registerEventConsumer(EventConsumerRegistry eventConsumerRegistry,
ThreadPoolBulkhead bulkHead,
CommonThreadPoolBulkheadConfigurationProperties bulkheadConfigurationProperties) {
int eventConsumerBufferSize = ofNullable(bulkheadConfigurationProperties.getBackendProperties(bulkHead.getName()))
.map(
CommonThreadPoolBulkheadConfigurationProperties.InstanceProperties::getEventConsumerBufferSize)
.orElse(100);
bulkHead.getEventPublisher().onEvent(eventConsumerRegistry.createEventConsumer(
String.join("-", ThreadPoolBulkhead.class.getSimpleName(), bulkHead.getName()),
eventConsumerBufferSize));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy