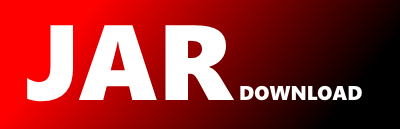
io.github.resilience4j.ratelimiter.VavrRateLimiter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resilience4j-vavr Show documentation
Show all versions of resilience4j-vavr Show documentation
Resilience4j is a lightweight, easy-to-use fault tolerance library designed for Java8 and functional programming
/*
*
* Copyright 2020: KrnSaurabh
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*
*/
package io.github.resilience4j.ratelimiter;
import io.vavr.CheckedFunction0;
import io.vavr.CheckedFunction1;
import io.vavr.CheckedRunnable;
import io.vavr.control.Either;
import io.vavr.control.Try;
import java.util.function.Function;
import java.util.function.Supplier;
import static io.github.resilience4j.ratelimiter.RateLimiter.waitForPermission;
public interface VavrRateLimiter {
/**
* Creates a supplier which is restricted by a RateLimiter.
*
* @param rateLimiter the RateLimiter
* @param supplier the original supplier
* @param the type of results supplied supplier
* @return a supplier which is restricted by a RateLimiter.
*/
static CheckedFunction0 decorateCheckedSupplier(RateLimiter rateLimiter, CheckedFunction0 supplier) {
return decorateCheckedSupplier(rateLimiter, 1, supplier);
}
/**
* Creates a supplier which is restricted by a RateLimiter.
*
* @param rateLimiter the RateLimiter
* @param permits number of permits that this call requires
* @param supplier the original supplier
* @param the type of results supplied supplier
* @return a supplier which is restricted by a RateLimiter.
*/
static CheckedFunction0 decorateCheckedSupplier(RateLimiter rateLimiter, int permits,
CheckedFunction0 supplier) {
return () -> {
waitForPermission(rateLimiter, permits);
try {
T result = supplier.apply();
rateLimiter.onResult(result);
return result;
} catch (Exception exception) {
rateLimiter.onError(exception);
throw exception;
}
};
}
/**
* Creates a runnable which is restricted by a RateLimiter.
*
* @param rateLimiter the RateLimiter
* @param runnable the original runnable
* @return a runnable which is restricted by a RateLimiter.
*/
static CheckedRunnable decorateCheckedRunnable(RateLimiter rateLimiter, CheckedRunnable runnable) {
return decorateCheckedRunnable(rateLimiter, 1, runnable);
}
/**
* Creates a runnable which is restricted by a RateLimiter.
*
* @param rateLimiter the RateLimiter
* @param permits number of permits that this call requires
* @param runnable the original runnable
* @return a runnable which is restricted by a RateLimiter.
*/
static CheckedRunnable decorateCheckedRunnable(RateLimiter rateLimiter, int permits, CheckedRunnable runnable) {
return () -> {
waitForPermission(rateLimiter, permits);
try {
runnable.run();
rateLimiter.onSuccess();
} catch (Exception exception) {
rateLimiter.onError(exception);
throw exception;
}
};
}
/**
* Creates a function which is restricted by a RateLimiter.
*
* @param rateLimiter the RateLimiter
* @param function the original function
* @param the type of function argument
* @param the type of function results
* @return a function which is restricted by a RateLimiter.
*/
static CheckedFunction1 decorateCheckedFunction(RateLimiter rateLimiter,
CheckedFunction1 function) {
return decorateCheckedFunction(rateLimiter, 1, function);
}
/**
* Creates a function which is restricted by a RateLimiter.
*
* @param rateLimiter the RateLimiter
* @param permits number of permits that this call requires
* @param function the original function
* @param the type of function argument
* @param the type of function results
* @return a function which is restricted by a RateLimiter.
*/
static CheckedFunction1 decorateCheckedFunction(RateLimiter rateLimiter,
int permits, CheckedFunction1 function) {
return (T t) -> decorateCheckedSupplier(rateLimiter, permits, () -> function.apply(t))
.apply();
}
/**
* Creates a function which is restricted by a RateLimiter.
*
* @param rateLimiter the RateLimiter
* @param permitsCalculator calculates the number of permits required by this call based on the
* functions argument
* @param function the original function
* @param the type of function argument
* @param the type of function results
* @return a function which is restricted by a RateLimiter.
*/
static CheckedFunction1 decorateCheckedFunction(RateLimiter rateLimiter,
Function permitsCalculator, CheckedFunction1 function) {
return (T t) -> decorateCheckedFunction(rateLimiter, permitsCalculator.apply(t), function)
.apply(t);
}
/**
* Creates a supplier which is restricted by a RateLimiter.
*
* @param rateLimiter the RateLimiter
* @param supplier the original supplier
* @param the type of results supplied supplier
* @return a supplier which is restricted by a RateLimiter.
*/
static Supplier> decorateTrySupplier(RateLimiter rateLimiter, Supplier> supplier) {
return decorateTrySupplier(rateLimiter, 1, supplier);
}
/**
* Creates a supplier which is restricted by a RateLimiter.
*
* @param rateLimiter the RateLimiter
* @param permits number of permits that this call requires
* @param supplier the original supplier
* @param the type of results supplied supplier
* @return a supplier which is restricted by a RateLimiter.
*/
static Supplier> decorateTrySupplier(RateLimiter rateLimiter, int permits, Supplier> supplier) {
return () -> {
try {
waitForPermission(rateLimiter, permits);
try {
Try result = supplier.get();
if (result.isSuccess()) {
rateLimiter.onResult(result.get());
} else {
rateLimiter.onError(result.getCause());
}
return result;
} catch (Exception exception) {
rateLimiter.onError(exception);
throw exception;
}
} catch (RequestNotPermitted requestNotPermitted) {
return Try.failure(requestNotPermitted);
}
};
}
/**
* Creates a supplier which is restricted by a RateLimiter.
*
* @param rateLimiter the RateLimiter
* @param supplier the original supplier
* @param the type of results supplied supplier
* @return a supplier which is restricted by a RateLimiter.
*/
static Supplier> decorateEitherSupplier(RateLimiter rateLimiter,
Supplier> supplier) {
return decorateEitherSupplier(rateLimiter, 1, supplier);
}
/**
* Creates a supplier which is restricted by a RateLimiter.
*
* @param rateLimiter the RateLimiter
* @param permits number of permits that this call requires
* @param supplier the original supplier
* @param the type of results supplied supplier
* @return a supplier which is restricted by a RateLimiter.
*/
static Supplier> decorateEitherSupplier(RateLimiter rateLimiter,
int permits, Supplier> supplier) {
return () -> {
try {
waitForPermission(rateLimiter, permits);
try {
Either extends Exception, T> result = supplier.get();
if (result.isRight()) {
rateLimiter.onResult(result.get());
} else {
rateLimiter.onError(result.getLeft());
}
return Either.narrow(result);
} catch (Exception exception) {
rateLimiter.onError(exception);
throw exception;
}
} catch (RequestNotPermitted requestNotPermitted) {
return Either.left(requestNotPermitted);
}
};
}
/**
* Decorates and executes the decorated Supplier.
*
* @param supplier the original Supplier
* @param the type of results supplied by this supplier
* @return the result of the decorated Supplier.
*/
static Try executeTrySupplier(RateLimiter rateLimiter,Supplier> supplier) {
return executeTrySupplier(rateLimiter,1, supplier);
}
/**
* Decorates and executes the decorated Supplier.
*
* @param permits number of permits that this call requires
* @param supplier the original Supplier
* @param the type of results supplied by this supplier
* @return the result of the decorated Supplier.
*/
static Try executeTrySupplier(RateLimiter rateLimiter, int permits, Supplier> supplier) {
return decorateTrySupplier(rateLimiter, permits, supplier).get();
}
/**
* Decorates and executes the decorated Supplier.
*
* @param supplier the original Supplier
* @param the type of results supplied by this supplier
* @return the result of the decorated Supplier.
*/
static Either executeEitherSupplier(RateLimiter rateLimiter,
Supplier> supplier) {
return executeEitherSupplier(rateLimiter,1, supplier);
}
/**
* Decorates and executes the decorated Supplier.
*
* @param permits number of permits that this call requires
* @param supplier the original Supplier
* @param the type of results supplied by this supplier
* @return the result of the decorated Supplier.
*/
static Either executeEitherSupplier(RateLimiter rateLimiter, int permits,
Supplier> supplier) {
return decorateEitherSupplier(rateLimiter, permits, supplier).get();
}
/**
* Decorates and executes the decorated Supplier.
*
* @param checkedSupplier the original Supplier
* @param the type of results supplied by this supplier
* @return the result of the decorated Supplier.
* @throws Throwable if something goes wrong applying this function to the given arguments
*/
static T executeCheckedSupplier(RateLimiter rateLimiter, CheckedFunction0 checkedSupplier) throws Throwable {
return executeCheckedSupplier(rateLimiter,1, checkedSupplier);
}
/**
* Decorates and executes the decorated Supplier.
*
* @param permits number of permits that this call requires
* @param checkedSupplier the original Supplier
* @param the type of results supplied by this supplier
* @return the result of the decorated Supplier.
* @throws Throwable if something goes wrong applying this function to the given arguments
*/
static T executeCheckedSupplier(RateLimiter rateLimiter, int permits, CheckedFunction0 checkedSupplier)
throws Throwable {
return decorateCheckedSupplier(rateLimiter, permits, checkedSupplier).apply();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy