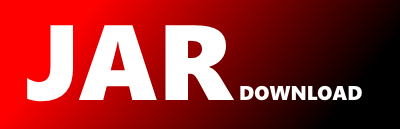
io.github.rmuhamedgaliev.service.HDD.impl.HDDInformationImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of SystemInformation Show documentation
Show all versions of SystemInformation Show documentation
Library for getting information about local computer implemented on java. Used 2 Linux commands, other implemented on Java.
package io.github.rmuhamedgaliev.service.HDD.impl;
import org.springframework.stereotype.Service;
import io.github.rmuhamedgaliev.service.HDD.HDDInformation;
import javax.swing.filechooser.FileSystemView;
import java.io.BufferedReader;
import java.io.File;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.file.*;
import java.nio.file.attribute.BasicFileAttributes;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.StringTokenizer;
/**
* Developer: Rinat Muhamedgaliev
* Date: 07.03.13
*/
@Service(value = "hddInformation")
public class HDDInformationImpl implements HDDInformation {
private Long diskSpace = null;
private final static int KILO = 1024;
@Override
public List getListPathInDirectory(Path path) {
final List pathList = new ArrayList<>();
try {
Files.walkFileTree(Paths.get(path.toString()), new SimpleFileVisitor() {
@Override
public FileVisitResult visitFile(Path file, BasicFileAttributes attrs) throws IOException {
if (file.toFile().exists() && file.toFile().canRead())
{
pathList.add(file);
}
return super.visitFile(file, attrs);
}
});
}
catch (IOException e)
{
e.printStackTrace();
}
return pathList;
}
@Override
public List getRootPathForLinux()
{
return Arrays.asList(FileSystemView.getFileSystemView().getRoots());
}
@Override
public Long getTotalSizePartition(File partition, String measure)
{
Long totalSpace;
totalSpace = partition.getTotalSpace();
if (measure == null)
{
return totalSpace;
}
else if (measure.trim().length() > 0)
{
switch (measure)
{
case "KiB":
diskSpace = totalSpace / KILO;
break;
case "MiB":
diskSpace = totalSpace / KILO / KILO;
break;
case "GiB":
diskSpace = totalSpace / KILO / KILO / KILO;
break;
default:
diskSpace = totalSpace;
break;
}
}
return diskSpace;
}
@Override
public Long getFreeSizePartition(File partition, String measure)
{
Long freeSpace;
freeSpace = partition.getFreeSpace();
if (measure == null)
{
return freeSpace;
}
else if (measure.trim().length() > 0)
{
switch (measure){
case "KiB":
diskSpace = freeSpace / KILO;
break;
case "MiB":
diskSpace = freeSpace / KILO / KILO;
break;
case "GiB":
diskSpace = freeSpace / KILO / KILO / KILO;
break;
default:
diskSpace = freeSpace;
break;
}
}
return diskSpace;
}
@Override
public Long getUsedSizePartition(File partition, String measure)
{
return getTotalSizePartition(partition, measure) - getFreeSizePartition(partition, measure);
}
public List getMountedDisks()
{
List disks = new ArrayList<>();
try
{
Process process = Runtime.getRuntime().exec("df");
BufferedReader outputCommand = new BufferedReader(new InputStreamReader(process.getInputStream(), "UTF-8"));
outputCommand.readLine();
int iterator = 0;
String output;
while ((output = outputCommand.readLine()) != null)
{
StringTokenizer stringTokenizer = new StringTokenizer(output, " ");
while (stringTokenizer.hasMoreElements())
{
String value = String.valueOf(stringTokenizer.nextElement());
iterator++;
if ((iterator % 6) == 0)
{
disks.add(new File(value));
}
}
}
outputCommand.close();
return disks;
} catch (Exception e){
e.printStackTrace();
}
return disks;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy