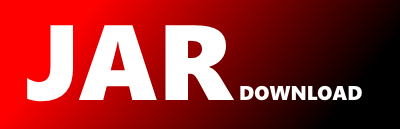
com.rcll.refbox.RefboxConnection Maven / Gradle / Ivy
package com.rcll.refbox;
import com.google.protobuf.GeneratedMessageV3;
import lombok.Getter;
import lombok.extern.apachecommons.CommonsLog;
import com.rcll.llsf_comm.ProtobufUpdBroadcastConnection;
import com.rcll.llsf_comm.ProtobufMessage;
import com.rcll.llsf_comm.ProtobufMessageHandler;
import java.io.IOException;
@CommonsLog
@Getter
class RefboxConnection {
private ProtobufUpdBroadcastConnection peer;
public RefboxConnection(String ip, int sendPort, int receivePort,
ProtobufMessageHandler handler, boolean encrypt, int cipher_type, String cryptoKey) {
log.info("Creating broadcast peer ip: " + ip + " sendPort: " + sendPort + " receivePort: " + receivePort);
peer = new ProtobufUpdBroadcastConnection(ip, sendPort, receivePort, encrypt, cipher_type, cryptoKey);
peer.register_handler(handler);
}
public RefboxConnection(String ip, int sendPort, int receivePort,
ProtobufMessageHandler handler) {
this(ip, sendPort, receivePort, handler, false, -1, null);
}
public void start(String threadName) throws IOException {
log.info("Starting Refbox Connection....");
peer.start(threadName);
}
public void add_message(Class classType) {
log.info("Adding message: " + classType.getName());
this.peer.add_message(classType);
}
public void enqueue(ProtobufMessage msg) {
this.peer.enqueue(msg);
}
public void enqueue(GeneratedMessageV3 msg) {
this.peer.enqueue(msg);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy