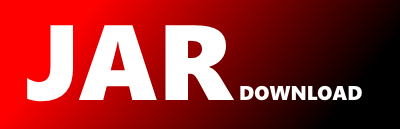
io.github.selcukes.commons.helper.SingletonContext Maven / Gradle / Ivy
/*
* Copyright (c) Ramesh Babu Prudhvi.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.selcukes.commons.helper;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Supplier;
import static java.util.Optional.ofNullable;
/**
* A thread-safe context for managing per-thread singleton instances of a type
* T. This class uses a ThreadLocal variable to store a thread-local map of
* singleton instances of type T, where each thread has its own instance. The
* instances are created lazily using a Supplier object passed to the
* constructor of this class. Example usage: SingletonContext
* context = SingletonContext.with(MySingleton::getInstance); MySingleton
* singleton = context.get(); // returns a thread-local singleton instance of
* MySingleton context.remove(); // removes the thread-local singleton instance
* for the current thread
*
* @param the type of the singleton instances managed by this context.
*/
public final class SingletonContext {
private final ThreadLocal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy