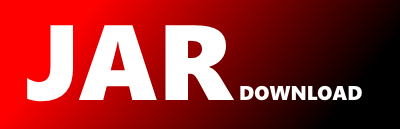
com.binance.api.client.BinanceApiAsyncRestClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of binance-api-client Show documentation
Show all versions of binance-api-client Show documentation
Java implementation for Binance API
package com.binance.api.client;
import com.binance.api.client.domain.account.Account;
import com.binance.api.client.domain.account.DepositAddress;
import com.binance.api.client.domain.account.DepositHistory;
import com.binance.api.client.domain.account.NewOrder;
import com.binance.api.client.domain.account.NewOrderResponse;
import com.binance.api.client.domain.account.Order;
import com.binance.api.client.domain.account.Trade;
import com.binance.api.client.domain.account.TradeHistoryItem;
import com.binance.api.client.domain.account.WithdrawHistory;
import com.binance.api.client.domain.account.WithdrawResult;
import com.binance.api.client.domain.account.request.AllOrdersRequest;
import com.binance.api.client.domain.account.request.CancelOrderRequest;
import com.binance.api.client.domain.account.request.CancelOrderResponse;
import com.binance.api.client.domain.account.request.OrderRequest;
import com.binance.api.client.domain.account.request.OrderStatusRequest;
import com.binance.api.client.domain.event.ListenKey;
import com.binance.api.client.domain.general.Asset;
import com.binance.api.client.domain.general.ExchangeInfo;
import com.binance.api.client.domain.general.ServerTime;
import com.binance.api.client.domain.market.AggTrade;
import com.binance.api.client.domain.market.BookTicker;
import com.binance.api.client.domain.market.Candlestick;
import com.binance.api.client.domain.market.CandlestickInterval;
import com.binance.api.client.domain.market.OrderBook;
import com.binance.api.client.domain.market.TickerPrice;
import com.binance.api.client.domain.market.TickerStatistics;
import java.util.List;
/**
* Binance API facade, supporting asynchronous/non-blocking access Binance's REST API.
*/
public interface BinanceApiAsyncRestClient {
// General endpoints
/**
* Test connectivity to the Rest API.
* @param callback a callback
*/
void ping(BinanceApiCallback callback);
/**
* Check server time.
* @param callback callback
*/
void getServerTime(BinanceApiCallback callback);
/**
* Current exchange trading rules and symbol information
* @param callback callback
*/
void getExchangeInfo(BinanceApiCallback callback);
/**
* ALL supported assets and whether or not they can be withdrawn.
* @param callback callback
*/
void getAllAssets(BinanceApiCallback> callback);
// Market Data endpoints
/**
* Get order book of a symbol (asynchronous)
*
* @param symbol ticker symbol (e.g. ETHBTC)
* @param limit depth of the order book (max 100)
* @param callback the callback that handles the response
*/
void getOrderBook(String symbol, Integer limit, BinanceApiCallback callback);
/**
* Get recent trades (up to last 500). Weight: 1
*
* @param symbol ticker symbol (e.g. ETHBTC)
* @param limit of last trades (Default 500; max 1000.)
* @param callback the callback that handles the response
*/
void getTrades(String symbol, Integer limit, BinanceApiCallback> callback);
/**
* Get older trades. Weight: 5
*
* @param symbol ticker symbol (e.g. ETHBTC)
* @param limit of last trades (Default 500; max 1000.)
* @param fromId TradeId to fetch from. Default gets most recent trades.
* @param callback the callback that handles the response
*/
void getHistoricalTrades(String symbol, Integer limit, Long fromId, BinanceApiCallback> callback);
/**
* Get compressed, aggregate trades. Trades that fill at the time, from the same order, with
* the same price will have the quantity aggregated.
*
* If both startTime
and endTime
are sent, limit
should not
* be sent AND the distance between startTime
and endTime
must be less than 24 hours.
*
* @param symbol symbol to aggregate (mandatory)
* @param fromId ID to get aggregate trades from INCLUSIVE (optional)
* @param limit Default 500; max 1000 (optional)
* @param startTime Timestamp in ms to get aggregate trades from INCLUSIVE (optional).
* @param endTime Timestamp in ms to get aggregate trades until INCLUSIVE (optional).
* @param callback the callback that handles the response
*/
void getAggTrades(String symbol, String fromId, Integer limit, Long startTime, Long endTime, BinanceApiCallback> callback);
/**
* Return the most recent aggregate trades for symbol
*
* @param symbol symbol
* @param callback callback
* @see #getAggTrades(String, String, Integer, Long, Long, BinanceApiCallback)
*/
void getAggTrades(String symbol, BinanceApiCallback> callback);
/**
* Kline/candlestick bars for a symbol. Klines are uniquely identified by their open time.
*
* @param symbol symbol to aggregate (mandatory)
* @param interval candlestick interval (mandatory)
* @param limit Default 500; max 1000 (optional)
* @param startTime Timestamp in ms to get candlestick bars from INCLUSIVE (optional).
* @param endTime Timestamp in ms to get candlestick bars until INCLUSIVE (optional).
* @param callback the callback that handles the response containing a candlestick bar for the given symbol and interval
*/
void getCandlestickBars(String symbol, CandlestickInterval interval, Integer limit, Long startTime, Long endTime, BinanceApiCallback> callback);
/**
* Kline/candlestick bars for a symbol. Klines are uniquely identified by their open time.
*
* @param symbol symbol to aggregate (mandatory)
* @param interval candlestick interval (mandatory)
* @param limit Default 500; max 1000 (optional)
* @param callback the callback that handles the response containing a candlestick bar for the given symbol and interval
*/
void getCandlestickBars(String symbol, CandlestickInterval interval, Integer limit, BinanceApiCallback> callback);
/**
* Kline/candlestick bars for a symbol. Klines are uniquely identified by their open time.
*
* @see #getCandlestickBars(String, CandlestickInterval, BinanceApiCallback)
*/
void getCandlestickBars(String symbol, CandlestickInterval interval, BinanceApiCallback> callback);
/**
* Get 24 hour price change statistics (asynchronous).
*
* @param symbol ticker symbol (e.g. ETHBTC)
* @param callback the callback that handles the response
*/
void get24HrPriceStatistics(String symbol, BinanceApiCallback callback);
/**
* Get 24 hour price change statistics for all symbols (asynchronous).
*
* @param callback the callback that handles the response
*/
void getAll24HrPriceStatistics(BinanceApiCallback> callback);
/**
* Get Latest price for all symbols (asynchronous).
*
* @param callback the callback that handles the response
*/
void getAllPrices(BinanceApiCallback> callback);
/**
* Get latest price for symbol
(asynchronous).
*
* @param symbol ticker symbol (e.g. ETHBTC)
* @param callback the callback that handles the response
*/
void getPrice(String symbol , BinanceApiCallback callback);
/**
* Get best price/qty on the order book for all symbols (asynchronous).
*
* @param callback the callback that handles the response
*/
void getBookTickers(BinanceApiCallback> callback);
// Account endpoints
/**
* Send in a new order (asynchronous)
*
* @param order the new order to submit.
* @param callback the callback that handles the response
*/
void newOrder(NewOrder order, BinanceApiCallback callback);
/**
* Test new order creation and signature/recvWindow long. Creates and validates a new order but does not send it into the matching engine.
*
* @param order the new TEST order to submit.
* @param callback the callback that handles the response
*/
void newOrderTest(NewOrder order, BinanceApiCallback callback);
/**
* Check an order's status (asynchronous).
*
* @param orderStatusRequest order status request parameters
* @param callback the callback that handles the response
*/
void getOrderStatus(OrderStatusRequest orderStatusRequest, BinanceApiCallback callback);
/**
* Cancel an active order (asynchronous).
*
* @param cancelOrderRequest order status request parameters
* @param callback the callback that handles the response
*/
void cancelOrder(CancelOrderRequest cancelOrderRequest, BinanceApiCallback callback);
/**
* Get all open orders on a symbol (asynchronous).
*
* @param orderRequest order request parameters
* @param callback the callback that handles the response
*/
void getOpenOrders(OrderRequest orderRequest, BinanceApiCallback> callback);
/**
* Get all account orders; active, canceled, or filled.
*
* @param orderRequest order request parameters
* @param callback the callback that handles the response
*/
void getAllOrders(AllOrdersRequest orderRequest, BinanceApiCallback> callback);
/**
* Get current account information (async).
*/
void getAccount(Long recvWindow, Long timestamp, BinanceApiCallback callback);
/**
* Get current account information using default parameters (async).
*/
void getAccount(BinanceApiCallback callback);
/**
* Get trades for a specific account and symbol.
*
* @param symbol symbol to get trades from
* @param limit default 500; max 1000
* @param fromId TradeId to fetch from. Default gets most recent trades.
* @param callback the callback that handles the response with a list of trades
*/
void getMyTrades(String symbol, Integer limit, Long fromId, Long recvWindow, Long timestamp, BinanceApiCallback> callback);
/**
* Get trades for a specific account and symbol.
*
* @param symbol symbol to get trades from
* @param limit default 500; max 1000
* @param callback the callback that handles the response with a list of trades
*/
void getMyTrades(String symbol, Integer limit, BinanceApiCallback> callback);
/**
* Get trades for a specific account and symbol.
*
* @param symbol symbol to get trades from
* @param callback the callback that handles the response with a list of trades
*/
void getMyTrades(String symbol, BinanceApiCallback> callback);
/**
* Submit a withdraw request.
*
* Enable Withdrawals option has to be active in the API settings.
*
* @param asset asset symbol to withdraw
* @param address address to withdraw to
* @param amount amount to withdraw
* @param name description/alias of the address
* @param addressTag Secondary address identifier for coins like XRP,XMR etc.
*/
void withdraw(String asset, String address, String amount, String name, String addressTag, BinanceApiCallback callback);
/**
* Fetch account deposit history.
*
* @param callback the callback that handles the response and returns the deposit history
*/
void getDepositHistory(String asset, BinanceApiCallback callback);
/**
* Fetch account withdraw history.
*
* @param callback the callback that handles the response and returns the withdraw history
*/
void getWithdrawHistory(String asset, BinanceApiCallback callback);
/**
* Fetch deposit address.
*
* @param callback the callback that handles the response and returns the deposit address
*/
void getDepositAddress(String asset, BinanceApiCallback callback);
// User stream endpoints
/**
* Start a new user data stream.
*
* @param callback the callback that handles the response which contains a listenKey
*/
void startUserDataStream(BinanceApiCallback callback);
/**
* PING a user data stream to prevent a time out.
*
* @param listenKey listen key that identifies a data stream
* @param callback the callback that handles the response which contains a listenKey
*/
void keepAliveUserDataStream(String listenKey, BinanceApiCallback callback);
/**
* Close out a new user data stream.
*
* @param listenKey listen key that identifies a data stream
* @param callback the callback that handles the response which contains a listenKey
*/
void closeUserDataStream(String listenKey, BinanceApiCallback callback);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy