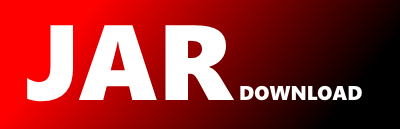
com.binance.api.client.domain.general.ExchangeInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of binance-api-client Show documentation
Show all versions of binance-api-client Show documentation
Java implementation for Binance API
package com.binance.api.client.domain.general;
import com.binance.api.client.constant.BinanceApiConstants;
import com.binance.api.client.exception.BinanceApiException;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import org.apache.commons.lang3.builder.ToStringBuilder;
import java.util.List;
/**
* Current exchange trading rules and symbol information.
* https://github.com/binance-exchange/binance-official-api-docs/blob/master/rest-api.md
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class ExchangeInfo {
private String timezone;
private Long serverTime;
private List rateLimits;
// private List exchangeFilters;
private List symbols;
public String getTimezone() {
return timezone;
}
public void setTimezone(String timezone) {
this.timezone = timezone;
}
public Long getServerTime() {
return serverTime;
}
public void setServerTime(Long serverTime) {
this.serverTime = serverTime;
}
public List getRateLimits() {
return rateLimits;
}
public void setRateLimits(List rateLimits) {
this.rateLimits = rateLimits;
}
public List getSymbols() {
return symbols;
}
public void setSymbols(List symbols) {
this.symbols = symbols;
}
/**
* @param symbol the symbol to obtain information for (e.g. ETHBTC)
* @return symbol exchange information
*/
public SymbolInfo getSymbolInfo(String symbol) {
return symbols.stream().filter(symbolInfo -> symbolInfo.getSymbol().equals(symbol))
.findFirst()
.orElseThrow(() -> new BinanceApiException("Unable to obtain information for symbol " + symbol));
}
@Override
public String toString() {
return new ToStringBuilder(this, BinanceApiConstants.TO_STRING_BUILDER_STYLE)
.append("timezone", timezone)
.append("serverTime", serverTime)
.append("rateLimits", rateLimits)
.append("symbols", symbols)
.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy