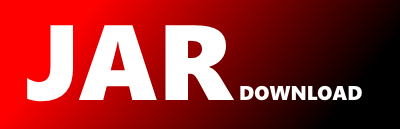
com.binance.api.client.impl.BinanceApiCallbackAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of binance-api-client Show documentation
Show all versions of binance-api-client Show documentation
Java implementation for Binance API
package com.binance.api.client.impl;
import com.binance.api.client.BinanceApiCallback;
import com.binance.api.client.BinanceApiError;
import com.binance.api.client.exception.BinanceApiException;
import retrofit2.Call;
import retrofit2.Callback;
import retrofit2.Response;
import java.io.IOException;
import static com.binance.api.client.impl.BinanceApiServiceGenerator.getBinanceApiError;
/**
* An adapter/wrapper which transforms a Callback from Retrofit into a BinanceApiCallback which is exposed to the client.
*/
public class BinanceApiCallbackAdapter implements Callback {
private final BinanceApiCallback callback;
public BinanceApiCallbackAdapter(BinanceApiCallback callback) {
this.callback = callback;
}
public void onResponse(Call call, Response response) {
if (response.isSuccessful()) {
callback.onResponse(response.body());
} else {
if (response.code() == 504) {
// HTTP 504 return code is used when the API successfully sent the message but not get a response within the timeout period.
// It is important to NOT treat this as a failure; the execution status is UNKNOWN and could have been a success.
return;
}
try {
BinanceApiError apiError = getBinanceApiError(response);
onFailure(call, new BinanceApiException(apiError));
} catch (IOException e) {
onFailure(call, new BinanceApiException(e));
}
}
}
@Override
public void onFailure(Call call, Throwable throwable) {
if (throwable instanceof BinanceApiException) {
callback.onFailure(throwable);
} else {
callback.onFailure(new BinanceApiException(throwable));
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy