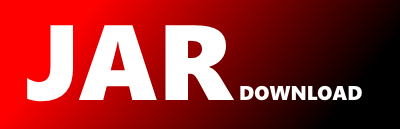
com.binance.api.client.impl.BinanceApiServiceGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of binance-api-client Show documentation
Show all versions of binance-api-client Show documentation
Java implementation for Binance API
package com.binance.api.client.impl;
import com.binance.api.client.BinanceApiError;
import com.binance.api.client.config.BinanceApiConfig;
import com.binance.api.client.exception.BinanceApiException;
import com.binance.api.client.security.AuthenticationInterceptor;
import okhttp3.Dispatcher;
import okhttp3.OkHttpClient;
import okhttp3.ResponseBody;
import org.apache.commons.lang3.StringUtils;
import retrofit2.Call;
import retrofit2.Converter;
import retrofit2.Response;
import retrofit2.Retrofit;
import retrofit2.converter.jackson.JacksonConverterFactory;
import java.io.IOException;
import java.lang.annotation.Annotation;
import java.util.concurrent.TimeUnit;
/**
* Generates a Binance API implementation based on @see {@link BinanceApiService}.
*/
public class BinanceApiServiceGenerator {
private static final OkHttpClient sharedClient;
private static final Converter.Factory converterFactory = JacksonConverterFactory.create();
static {
Dispatcher dispatcher = new Dispatcher();
dispatcher.setMaxRequestsPerHost(500);
dispatcher.setMaxRequests(500);
sharedClient = new OkHttpClient.Builder()
.dispatcher(dispatcher)
.pingInterval(20, TimeUnit.SECONDS)
.build();
}
@SuppressWarnings("unchecked")
private static final Converter errorBodyConverter =
(Converter)converterFactory.responseBodyConverter(
BinanceApiError.class, new Annotation[0], null);
public static S createService(Class serviceClass) {
return createService(serviceClass, null, null);
}
/**
* Create a Binance API service.
*
* @param serviceClass the type of service.
* @param apiKey Binance API key.
* @param secret Binance secret.
*
* @return a new implementation of the API endpoints for the Binance API service.
*/
public static S createService(Class serviceClass, String apiKey, String secret) {
String baseUrl = null;
if (!BinanceApiConfig.useTestnet) { baseUrl = BinanceApiConfig.getApiBaseUrl(); }
else {
baseUrl = /*BinanceApiConfig.useTestnetStreaming ?
BinanceApiConfig.getStreamTestNetBaseUrl() :*/
BinanceApiConfig.getTestNetBaseUrl();
}
Retrofit.Builder retrofitBuilder = new Retrofit.Builder()
.baseUrl(baseUrl)
.addConverterFactory(converterFactory);
if (StringUtils.isEmpty(apiKey) || StringUtils.isEmpty(secret)) {
retrofitBuilder.client(sharedClient);
} else {
// `adaptedClient` will use its own interceptor, but share thread pool etc with the 'parent' client
AuthenticationInterceptor interceptor = new AuthenticationInterceptor(apiKey, secret);
OkHttpClient adaptedClient = sharedClient.newBuilder().addInterceptor(interceptor).build();
retrofitBuilder.client(adaptedClient);
}
Retrofit retrofit = retrofitBuilder.build();
return retrofit.create(serviceClass);
}
/**
* Execute a REST call and block until the response is received.
*/
public static T executeSync(Call call) {
try {
Response response = call.execute();
if (response.isSuccessful()) {
return response.body();
} else {
BinanceApiError apiError = getBinanceApiError(response);
throw new BinanceApiException(apiError);
}
} catch (IOException e) {
throw new BinanceApiException(e);
}
}
/**
* Extracts and converts the response error body into an object.
*/
public static BinanceApiError getBinanceApiError(Response> response) throws IOException, BinanceApiException {
return errorBodyConverter.convert(response.errorBody());
}
/**
* Returns the shared OkHttpClient instance.
*/
public static OkHttpClient getSharedClient() {
return sharedClient;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy