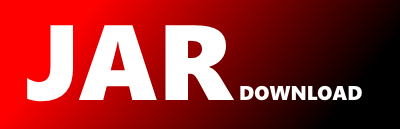
io.github.serpro69.kfaker.RandomService.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kotlin-faker Show documentation
Show all versions of kotlin-faker Show documentation
Generate realistically looking fake data such as names, addresses, banking details, and many more, that can be used for testing and data anonymization purposes.
package io.github.serpro69.kfaker
import java.util.*
/**
* Wrapper around [Random] that contains some extra helper functions.
*/
internal class RandomService(private val random: Random) {
private val alphabeticSource = "abcdefghijklmnopqrstuvwxyz"
fun nextInt() = random.nextInt()
fun nextInt(bound: Int) = random.nextInt(bound)
fun nextInt(intRange: IntRange): Int {
val lowerBound = requireNotNull(intRange.minOrNull())
val upperBound = requireNotNull(intRange.maxOrNull())
return nextInt(lowerBound, upperBound)
}
fun nextInt(min: Int, max: Int) = random.nextInt(max - min + 1) + min
fun randomValue(list: List) = list[nextInt(list.size)]
fun randomValue(array: Array) = array[nextInt(array.size)]
fun nextLetter(upper: Boolean): Char {
val source = if (upper) alphabeticSource.toUpperCase() else alphabeticSource
return source[nextInt(source.length)]
}
fun nextBoolean() = random.nextBoolean()
fun nextLong() = random.nextLong()
fun nextFloat() = random.nextFloat()
fun nextDouble() = random.nextDouble()
fun nextChar() = random.nextInt().toChar()
fun nextString() = List(100) { nextInt().toChar().toString() }.joinToString("")
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy