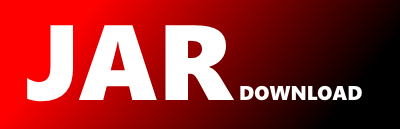
io.github.serpro69.kfaker.provider.unique.GlobalUniqueDataDataProvider.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kotlin-faker Show documentation
Show all versions of kotlin-faker Show documentation
Generate realistically looking fake data such as names, addresses, banking details, and many more, that can be used for testing and data anonymization purposes.
package io.github.serpro69.kfaker.provider.unique
import io.github.serpro69.kfaker.provider.FakeDataProvider
import io.github.serpro69.kfaker.Faker
import kotlin.reflect.KClass
import kotlin.reflect.KProperty0
/**
* Global provider for unique values.
*
* This provider is used in [Faker] class to control global unique generation configuration of faker providers.
*
* Example usage:
* ```
* val faker = Faker()
* faker.unique.configuration {
* enable(faker::address) // enables unique generation for all functions of Address provider
* enable(faker::name) // enables unique generation for all functions of Name provider
* exclude(listOfValues) // exclude values from `listOfValue` collection from being generated (for all providers that are enabled for unique generation)
* enable(faker::internet) { enables unique generation for all functions of Internet provider
* exclude(listOfValues) // exclude values from `listOfValue` collection from being generated with Internet provider
* exclude(faker::internet, listOfPatterns)
* }
* }
* ```
*/
@Suppress("UNCHECKED_CAST")
class GlobalUniqueDataDataProvider internal constructor() : UniqueDataProvider() {
@JvmSynthetic
@PublishedApi
internal val config = UniqueProviderConfiguration()
/**
* Disables "unique generation" for all providers that were configured to return unique values,
* and clears out any already returned values, so they can possibly be returned again.
*/
override fun disableAll() {
config.disableAll()
}
/**
* Clears the already returned (used) unique values and exclusion patterns so that values can again be returned.
*/
override fun clearAll() {
config.usedProviderFunctionValues.keys.forEach { k -> config.usedProviderFunctionValues[k] = hashMapOf() }
config.providerFunctionExclusionPatterns.keys.forEach { k -> config.providerFunctionExclusionPatterns[k] = hashMapOf() }
}
fun clear(providerProperty: KProperty0) {
config.clear(providerProperty.returnType.classifier as KClass)
}
@Deprecated(
level = DeprecationLevel.WARNING,
message = "This functionality is deprecated and will be removed in release 1.7.0",
replaceWith = ReplaceWith("faker.unique.configuration { this.exclude(funcName, values) }")
)
inline fun exclude(funcName: String, values: List) {
exclude(funcName, *values.toTypedArray())
}
@Deprecated(
level = DeprecationLevel.WARNING,
message = "This functionality is deprecated and will be removed in release 1.7.0",
replaceWith = ReplaceWith("faker.unique.configuration { this.exclude(funcName, values) }")
)
inline fun exclude(funcName: String, vararg values: String) {
if (config.markedUnique.contains(T::class)) {
config.usedProviderFunctionValues[T::class]?.merge(funcName, values.toMutableSet()) { oldSet, newSet ->
oldSet.apply { addAll(newSet) }
}
}
}
@Deprecated(
level = DeprecationLevel.WARNING,
message = "This functionality is deprecated and will be removed in release 1.7.0",
replaceWith = ReplaceWith("faker.unique.configuration { this.enable(providerProperty) }")
)
fun enable(providerProperty: KProperty0) {
config.enable(providerProperty.returnType.classifier as KClass)
}
@Deprecated(
level = DeprecationLevel.WARNING,
message = "This functionality is deprecated and will be removed in release 1.7.0",
replaceWith = ReplaceWith("faker.unique.configuration { this.disable(providerProperty) }")
)
fun disable(providerProperty: KProperty0) {
config.disable(providerProperty.returnType.classifier as KClass)
}
/**
* Configures `this` Unique provider.
*/
fun configuration(function: UniqueProviderConfiguration.() -> Unit) {
config.apply(function)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy