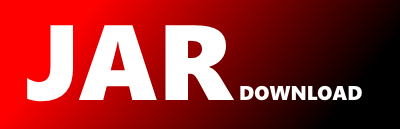
com.alphasystem.docx4j.builder.wml.CTBorderBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of open-xml-builder Show documentation
Show all versions of open-xml-builder Show documentation
Alpha system commons library
package com.alphasystem.docx4j.builder.wml;
import java.math.BigInteger;
import com.alphasystem.docx4j.builder.OpenXmlBuilder;
import org.docx4j.wml.CTBorder;
import org.docx4j.wml.STBorder;
import org.docx4j.wml.STThemeColor;
/**
* Fluent API builder for org.docx4j.wml.CTBorder
.
*
*/
public class CTBorderBuilder
extends OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public CTBorderBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public CTBorderBuilder(CTBorder object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public CTBorderBuilder(CTBorder src, CTBorder target) {
this(target);
if (src!= null) {
withVal(src.getVal()).withColor(src.getColor()).withThemeColor(src.getThemeColor()).withThemeTint(src.getThemeTint()).withThemeShade(src.getThemeShade()).withSz(WmlBuilderFactory.cloneBigInteger(src.getSz())).withSpace(WmlBuilderFactory.cloneBigInteger(src.getSpace())).withShadow(WmlBuilderFactory.cloneBoolean(src, "shadow")).withFrame(WmlBuilderFactory.cloneBoolean(src, "frame"));
}
}
protected CTBorder createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createCTBorder();
}
public CTBorderBuilder withVal(STBorder value) {
if (value!= null) {
object.setVal(value);
}
return this;
}
public CTBorderBuilder withColor(String value) {
if (value!= null) {
object.setColor(value);
}
return this;
}
public CTBorderBuilder withThemeColor(STThemeColor value) {
if (value!= null) {
object.setThemeColor(value);
}
return this;
}
public CTBorderBuilder withThemeTint(String value) {
if (value!= null) {
object.setThemeTint(value);
}
return this;
}
public CTBorderBuilder withThemeShade(String value) {
if (value!= null) {
object.setThemeShade(value);
}
return this;
}
public CTBorderBuilder withSz(BigInteger value) {
if (value!= null) {
object.setSz(value);
}
return this;
}
/**
* Calls setSz
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTBorderBuilder withSz(String value) {
if (value!= null) {
object.setSz(new BigInteger(value));
}
return this;
}
/**
* Calls setSz
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTBorderBuilder withSz(Long value) {
if (value!= null) {
object.setSz(BigInteger.valueOf(value));
}
return this;
}
public CTBorderBuilder withSpace(BigInteger value) {
if (value!= null) {
object.setSpace(value);
}
return this;
}
/**
* Calls setSpace
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTBorderBuilder withSpace(String value) {
if (value!= null) {
object.setSpace(new BigInteger(value));
}
return this;
}
/**
* Calls setSpace
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTBorderBuilder withSpace(Long value) {
if (value!= null) {
object.setSpace(BigInteger.valueOf(value));
}
return this;
}
public CTBorderBuilder withShadow(Boolean value) {
if (value!= null) {
object.setShadow(value);
}
return this;
}
public CTBorderBuilder withFrame(Boolean value) {
if (value!= null) {
object.setFrame(value);
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy