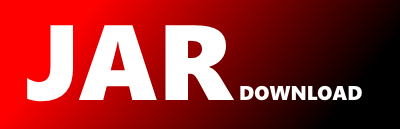
com.alphasystem.docx4j.builder.wml.CTFramePrBuilder Maven / Gradle / Ivy
package com.alphasystem.docx4j.builder.wml;
import java.math.BigInteger;
import com.alphasystem.docx4j.builder.OpenXmlBuilder;
import org.docx4j.wml.CTFramePr;
import org.docx4j.wml.STDropCap;
import org.docx4j.wml.STHAnchor;
import org.docx4j.wml.STHeightRule;
import org.docx4j.wml.STVAnchor;
import org.docx4j.wml.STWrap;
import org.docx4j.wml.STXAlign;
import org.docx4j.wml.STYAlign;
/**
* Fluent API builder for org.docx4j.wml.CTFramePr
.
*
*/
public class CTFramePrBuilder
extends OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public CTFramePrBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public CTFramePrBuilder(CTFramePr object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public CTFramePrBuilder(CTFramePr src, CTFramePr target) {
this(target);
if (src!= null) {
withDropCap(src.getDropCap()).withLines(WmlBuilderFactory.cloneBigInteger(src.getLines())).withW(WmlBuilderFactory.cloneBigInteger(src.getW())).withH(WmlBuilderFactory.cloneBigInteger(src.getH())).withVSpace(WmlBuilderFactory.cloneBigInteger(src.getVSpace())).withHSpace(WmlBuilderFactory.cloneBigInteger(src.getHSpace())).withWrap(src.getWrap()).withHAnchor(src.getHAnchor()).withVAnchor(src.getVAnchor()).withX(WmlBuilderFactory.cloneBigInteger(src.getX())).withXAlign(src.getXAlign()).withY(WmlBuilderFactory.cloneBigInteger(src.getY())).withYAlign(src.getYAlign()).withHRule(src.getHRule()).withAnchorLock(WmlBuilderFactory.cloneBoolean(src, "anchorLock"));
}
}
protected CTFramePr createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createCTFramePr();
}
public CTFramePrBuilder withDropCap(STDropCap value) {
if (value!= null) {
object.setDropCap(value);
}
return this;
}
public CTFramePrBuilder withLines(BigInteger value) {
if (value!= null) {
object.setLines(value);
}
return this;
}
/**
* Calls setLines
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTFramePrBuilder withLines(String value) {
if (value!= null) {
object.setLines(new BigInteger(value));
}
return this;
}
/**
* Calls setLines
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTFramePrBuilder withLines(Long value) {
if (value!= null) {
object.setLines(BigInteger.valueOf(value));
}
return this;
}
public CTFramePrBuilder withW(BigInteger value) {
if (value!= null) {
object.setW(value);
}
return this;
}
/**
* Calls setW
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTFramePrBuilder withW(String value) {
if (value!= null) {
object.setW(new BigInteger(value));
}
return this;
}
/**
* Calls setW
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTFramePrBuilder withW(Long value) {
if (value!= null) {
object.setW(BigInteger.valueOf(value));
}
return this;
}
public CTFramePrBuilder withH(BigInteger value) {
if (value!= null) {
object.setH(value);
}
return this;
}
/**
* Calls setH
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTFramePrBuilder withH(String value) {
if (value!= null) {
object.setH(new BigInteger(value));
}
return this;
}
/**
* Calls setH
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTFramePrBuilder withH(Long value) {
if (value!= null) {
object.setH(BigInteger.valueOf(value));
}
return this;
}
public CTFramePrBuilder withVSpace(BigInteger value) {
if (value!= null) {
object.setVSpace(value);
}
return this;
}
/**
* Calls setVSpace
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTFramePrBuilder withVSpace(String value) {
if (value!= null) {
object.setVSpace(new BigInteger(value));
}
return this;
}
/**
* Calls setVSpace
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTFramePrBuilder withVSpace(Long value) {
if (value!= null) {
object.setVSpace(BigInteger.valueOf(value));
}
return this;
}
public CTFramePrBuilder withHSpace(BigInteger value) {
if (value!= null) {
object.setHSpace(value);
}
return this;
}
/**
* Calls setHSpace
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTFramePrBuilder withHSpace(String value) {
if (value!= null) {
object.setHSpace(new BigInteger(value));
}
return this;
}
/**
* Calls setHSpace
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTFramePrBuilder withHSpace(Long value) {
if (value!= null) {
object.setHSpace(BigInteger.valueOf(value));
}
return this;
}
public CTFramePrBuilder withWrap(STWrap value) {
if (value!= null) {
object.setWrap(value);
}
return this;
}
public CTFramePrBuilder withHAnchor(STHAnchor value) {
if (value!= null) {
object.setHAnchor(value);
}
return this;
}
public CTFramePrBuilder withVAnchor(STVAnchor value) {
if (value!= null) {
object.setVAnchor(value);
}
return this;
}
public CTFramePrBuilder withX(BigInteger value) {
if (value!= null) {
object.setX(value);
}
return this;
}
/**
* Calls setX
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTFramePrBuilder withX(String value) {
if (value!= null) {
object.setX(new BigInteger(value));
}
return this;
}
/**
* Calls setX
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTFramePrBuilder withX(Long value) {
if (value!= null) {
object.setX(BigInteger.valueOf(value));
}
return this;
}
public CTFramePrBuilder withXAlign(STXAlign value) {
if (value!= null) {
object.setXAlign(value);
}
return this;
}
public CTFramePrBuilder withY(BigInteger value) {
if (value!= null) {
object.setY(value);
}
return this;
}
/**
* Calls setY
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTFramePrBuilder withY(String value) {
if (value!= null) {
object.setY(new BigInteger(value));
}
return this;
}
/**
* Calls setY
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTFramePrBuilder withY(Long value) {
if (value!= null) {
object.setY(BigInteger.valueOf(value));
}
return this;
}
public CTFramePrBuilder withYAlign(STYAlign value) {
if (value!= null) {
object.setYAlign(value);
}
return this;
}
public CTFramePrBuilder withHRule(STHeightRule value) {
if (value!= null) {
object.setHRule(value);
}
return this;
}
public CTFramePrBuilder withAnchorLock(Boolean value) {
if (value!= null) {
object.setAnchorLock(value);
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy