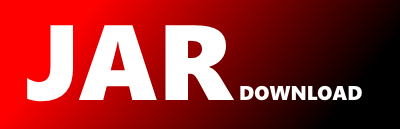
com.alphasystem.docx4j.builder.wml.CTTblPPrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of open-xml-builder Show documentation
Show all versions of open-xml-builder Show documentation
Alpha system commons library
package com.alphasystem.docx4j.builder.wml;
import java.math.BigInteger;
import com.alphasystem.docx4j.builder.OpenXmlBuilder;
import org.docx4j.wml.CTTblPPr;
import org.docx4j.wml.STHAnchor;
import org.docx4j.wml.STVAnchor;
import org.docx4j.wml.STXAlign;
import org.docx4j.wml.STYAlign;
/**
* Fluent API builder for org.docx4j.wml.CTTblPPr
.
*
*/
public class CTTblPPrBuilder
extends OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public CTTblPPrBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public CTTblPPrBuilder(CTTblPPr object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public CTTblPPrBuilder(CTTblPPr src, CTTblPPr target) {
this(target);
if (src!= null) {
withLeftFromText(WmlBuilderFactory.cloneBigInteger(src.getLeftFromText())).withRightFromText(WmlBuilderFactory.cloneBigInteger(src.getRightFromText())).withTopFromText(WmlBuilderFactory.cloneBigInteger(src.getTopFromText())).withBottomFromText(WmlBuilderFactory.cloneBigInteger(src.getBottomFromText())).withVertAnchor(src.getVertAnchor()).withHorzAnchor(src.getHorzAnchor()).withTblpXSpec(src.getTblpXSpec()).withTblpX(WmlBuilderFactory.cloneBigInteger(src.getTblpX())).withTblpYSpec(src.getTblpYSpec()).withTblpY(WmlBuilderFactory.cloneBigInteger(src.getTblpY()));
}
}
protected CTTblPPr createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createCTTblPPr();
}
public CTTblPPrBuilder withLeftFromText(BigInteger value) {
if (value!= null) {
object.setLeftFromText(value);
}
return this;
}
/**
* Calls setLeftFromText
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTTblPPrBuilder withLeftFromText(String value) {
if (value!= null) {
object.setLeftFromText(new BigInteger(value));
}
return this;
}
/**
* Calls setLeftFromText
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTTblPPrBuilder withLeftFromText(Long value) {
if (value!= null) {
object.setLeftFromText(BigInteger.valueOf(value));
}
return this;
}
public CTTblPPrBuilder withRightFromText(BigInteger value) {
if (value!= null) {
object.setRightFromText(value);
}
return this;
}
/**
* Calls setRightFromText
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTTblPPrBuilder withRightFromText(String value) {
if (value!= null) {
object.setRightFromText(new BigInteger(value));
}
return this;
}
/**
* Calls setRightFromText
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTTblPPrBuilder withRightFromText(Long value) {
if (value!= null) {
object.setRightFromText(BigInteger.valueOf(value));
}
return this;
}
public CTTblPPrBuilder withTopFromText(BigInteger value) {
if (value!= null) {
object.setTopFromText(value);
}
return this;
}
/**
* Calls setTopFromText
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTTblPPrBuilder withTopFromText(String value) {
if (value!= null) {
object.setTopFromText(new BigInteger(value));
}
return this;
}
/**
* Calls setTopFromText
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTTblPPrBuilder withTopFromText(Long value) {
if (value!= null) {
object.setTopFromText(BigInteger.valueOf(value));
}
return this;
}
public CTTblPPrBuilder withBottomFromText(BigInteger value) {
if (value!= null) {
object.setBottomFromText(value);
}
return this;
}
/**
* Calls setBottomFromText
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTTblPPrBuilder withBottomFromText(String value) {
if (value!= null) {
object.setBottomFromText(new BigInteger(value));
}
return this;
}
/**
* Calls setBottomFromText
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTTblPPrBuilder withBottomFromText(Long value) {
if (value!= null) {
object.setBottomFromText(BigInteger.valueOf(value));
}
return this;
}
public CTTblPPrBuilder withVertAnchor(STVAnchor value) {
if (value!= null) {
object.setVertAnchor(value);
}
return this;
}
public CTTblPPrBuilder withHorzAnchor(STHAnchor value) {
if (value!= null) {
object.setHorzAnchor(value);
}
return this;
}
public CTTblPPrBuilder withTblpXSpec(STXAlign value) {
if (value!= null) {
object.setTblpXSpec(value);
}
return this;
}
public CTTblPPrBuilder withTblpX(BigInteger value) {
if (value!= null) {
object.setTblpX(value);
}
return this;
}
/**
* Calls setTblpX
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTTblPPrBuilder withTblpX(String value) {
if (value!= null) {
object.setTblpX(new BigInteger(value));
}
return this;
}
/**
* Calls setTblpX
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTTblPPrBuilder withTblpX(Long value) {
if (value!= null) {
object.setTblpX(BigInteger.valueOf(value));
}
return this;
}
public CTTblPPrBuilder withTblpYSpec(STYAlign value) {
if (value!= null) {
object.setTblpYSpec(value);
}
return this;
}
public CTTblPPrBuilder withTblpY(BigInteger value) {
if (value!= null) {
object.setTblpY(value);
}
return this;
}
/**
* Calls setTblpY
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTTblPPrBuilder withTblpY(String value) {
if (value!= null) {
object.setTblpY(new BigInteger(value));
}
return this;
}
/**
* Calls setTblpY
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public CTTblPPrBuilder withTblpY(Long value) {
if (value!= null) {
object.setTblpY(BigInteger.valueOf(value));
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy