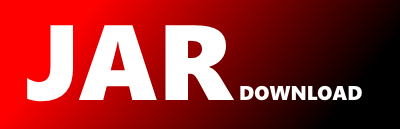
com.alphasystem.docx4j.builder.wml.CTTblPrExBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of open-xml-builder Show documentation
Show all versions of open-xml-builder Show documentation
Alpha system commons library
package com.alphasystem.docx4j.builder.wml;
import com.alphasystem.docx4j.builder.OpenXmlBuilder;
import org.docx4j.wml.CTShd;
import org.docx4j.wml.CTString;
import org.docx4j.wml.CTTblCellMar;
import org.docx4j.wml.CTTblLayoutType;
import org.docx4j.wml.CTTblLook;
import org.docx4j.wml.CTTblPrEx;
import org.docx4j.wml.CTTblPrExChange;
import org.docx4j.wml.Jc;
import org.docx4j.wml.JcEnumeration;
import org.docx4j.wml.STTblLayoutType;
import org.docx4j.wml.TblBorders;
import org.docx4j.wml.TblWidth;
/**
* Fluent API builder for org.docx4j.wml.CTTblPrEx
.
*
*/
public class CTTblPrExBuilder
extends OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public CTTblPrExBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public CTTblPrExBuilder(CTTblPrEx object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public CTTblPrExBuilder(CTTblPrEx src, CTTblPrEx target) {
this(target);
if (src!= null) {
CTTblPrExChange tblPrExChange = src.getTblPrExChange();
if (tblPrExChange!= null) {
tblPrExChange = new CTTblPrExChangeBuilder(tblPrExChange, object.getTblPrExChange()).getObject();
}
TblWidth tblW = src.getTblW();
if (tblW!= null) {
tblW = new com.alphasystem.docx4j.builder.wml.TblWidthBuilder(tblW, object.getTblW()).getObject();
}
Jc jc = src.getJc();
if (jc!= null) {
jc = new com.alphasystem.docx4j.builder.wml.JcBuilder(jc, object.getJc()).getObject();
}
TblWidth tblCellSpacing = src.getTblCellSpacing();
if (tblCellSpacing!= null) {
tblCellSpacing = new com.alphasystem.docx4j.builder.wml.TblWidthBuilder(tblCellSpacing, object.getTblCellSpacing()).getObject();
}
TblWidth tblInd = src.getTblInd();
if (tblInd!= null) {
tblInd = new com.alphasystem.docx4j.builder.wml.TblWidthBuilder(tblInd, object.getTblInd()).getObject();
}
TblBorders tblBorders = src.getTblBorders();
if (tblBorders!= null) {
tblBorders = new TblBordersBuilder(tblBorders, object.getTblBorders()).getObject();
}
CTShd shd = src.getShd();
if (shd!= null) {
shd = new CTShdBuilder(shd, object.getShd()).getObject();
}
CTTblLayoutType tblLayout = src.getTblLayout();
if (tblLayout!= null) {
tblLayout = new com.alphasystem.docx4j.builder.wml.CTTblLayoutTypeBuilder(tblLayout, object.getTblLayout()).getObject();
}
CTTblCellMar tblCellMar = src.getTblCellMar();
if (tblCellMar!= null) {
tblCellMar = new CTTblCellMarBuilder(tblCellMar, object.getTblCellMar()).getObject();
}
CTTblLook tblLook = src.getTblLook();
if (tblLook!= null) {
tblLook = new CTTblLookBuilder(tblLook, object.getTblLook()).getObject();
}
CTString tblCaption = src.getTblCaption();
if (tblCaption!= null) {
tblCaption = new com.alphasystem.docx4j.builder.wml.CTStringBuilder(tblCaption, object.getTblCaption()).getObject();
}
withTblPrExChange(tblPrExChange).withTblW(tblW).withJc(jc).withTblCellSpacing(tblCellSpacing).withTblInd(tblInd).withTblBorders(tblBorders).withShd(shd).withTblLayout(tblLayout).withTblCellMar(tblCellMar).withTblLook(tblLook).withTblCaption(tblCaption);
}
}
protected CTTblPrEx createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createCTTblPrEx();
}
public CTTblPrExBuilder withTblPrExChange(CTTblPrExChange value) {
if (value!= null) {
object.setTblPrExChange(value);
}
return this;
}
public CTTblPrExBuilder withTblW(TblWidth value) {
if (value!= null) {
object.setTblW(value);
}
return this;
}
public CTTblPrExBuilder withJc(Jc value) {
if (value!= null) {
object.setJc(value);
}
return this;
}
public CTTblPrExBuilder withJc(JcEnumeration val) {
if (val!= null) {
object.setJc(new com.alphasystem.docx4j.builder.wml.JcBuilder().withVal(val).getObject());
}
return this;
}
public CTTblPrExBuilder withTblCellSpacing(TblWidth value) {
if (value!= null) {
object.setTblCellSpacing(value);
}
return this;
}
public CTTblPrExBuilder withTblInd(TblWidth value) {
if (value!= null) {
object.setTblInd(value);
}
return this;
}
public CTTblPrExBuilder withTblBorders(TblBorders value) {
if (value!= null) {
object.setTblBorders(value);
}
return this;
}
public CTTblPrExBuilder withShd(CTShd value) {
if (value!= null) {
object.setShd(value);
}
return this;
}
public CTTblPrExBuilder withTblLayout(CTTblLayoutType value) {
if (value!= null) {
object.setTblLayout(value);
}
return this;
}
public CTTblPrExBuilder withTblLayout(STTblLayoutType type) {
if (type!= null) {
object.setTblLayout(new com.alphasystem.docx4j.builder.wml.CTTblLayoutTypeBuilder().withType(type).getObject());
}
return this;
}
public CTTblPrExBuilder withTblCellMar(CTTblCellMar value) {
if (value!= null) {
object.setTblCellMar(value);
}
return this;
}
public CTTblPrExBuilder withTblLook(CTTblLook value) {
if (value!= null) {
object.setTblLook(value);
}
return this;
}
public CTTblPrExBuilder withTblCaption(CTString value) {
if (value!= null) {
object.setTblCaption(value);
}
return this;
}
public CTTblPrExBuilder withTblCaption(String val) {
if (val!= null) {
object.setTblCaption(new com.alphasystem.docx4j.builder.wml.CTStringBuilder().withVal(val).getObject());
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy