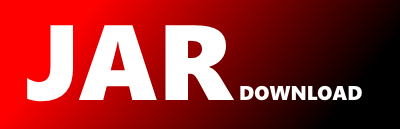
com.alphasystem.docx4j.builder.wml.CTTblStylePrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of open-xml-builder Show documentation
Show all versions of open-xml-builder Show documentation
Alpha system commons library
package com.alphasystem.docx4j.builder.wml;
import com.alphasystem.docx4j.builder.OpenXmlBuilder;
import org.docx4j.wml.CTTblPrBase;
import org.docx4j.wml.CTTblStylePr;
import org.docx4j.wml.PPr;
import org.docx4j.wml.RPr;
import org.docx4j.wml.STTblStyleOverrideType;
import org.docx4j.wml.TcPr;
import org.docx4j.wml.TrPr;
/**
* Fluent API builder for org.docx4j.wml.CTTblStylePr
.
*
*/
public class CTTblStylePrBuilder
extends OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public CTTblStylePrBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public CTTblStylePrBuilder(CTTblStylePr object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public CTTblStylePrBuilder(CTTblStylePr src, CTTblStylePr target) {
this(target);
if (src!= null) {
PPr pPr = src.getPPr();
if (pPr!= null) {
pPr = new PPrBuilder(pPr, object.getPPr()).getObject();
}
RPr rPr = src.getRPr();
if (rPr!= null) {
rPr = new RPrBuilder(rPr, object.getRPr()).getObject();
}
CTTblPrBase tblPr = src.getTblPr();
if (tblPr!= null) {
tblPr = new CTTblPrBaseBuilder(tblPr, object.getTblPr()).getObject();
}
TrPr trPr = src.getTrPr();
if (trPr!= null) {
trPr = new TrPrBuilder(trPr, object.getTrPr()).getObject();
}
TcPr tcPr = src.getTcPr();
if (tcPr!= null) {
tcPr = new TcPrBuilder(tcPr, object.getTcPr()).getObject();
}
withPPr(pPr).withRPr(rPr).withTblPr(tblPr).withTrPr(trPr).withTcPr(tcPr).withType(src.getType());
}
}
protected CTTblStylePr createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createCTTblStylePr();
}
public CTTblStylePrBuilder withPPr(PPr value) {
if (value!= null) {
object.setPPr(value);
}
return this;
}
public CTTblStylePrBuilder withRPr(RPr value) {
if (value!= null) {
object.setRPr(value);
}
return this;
}
public CTTblStylePrBuilder withTblPr(CTTblPrBase value) {
if (value!= null) {
object.setTblPr(value);
}
return this;
}
public CTTblStylePrBuilder withTrPr(TrPr value) {
if (value!= null) {
object.setTrPr(value);
}
return this;
}
public CTTblStylePrBuilder withTcPr(TcPr value) {
if (value!= null) {
object.setTcPr(value);
}
return this;
}
public CTTblStylePrBuilder withType(STTblStyleOverrideType value) {
if (value!= null) {
object.setType(value);
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy