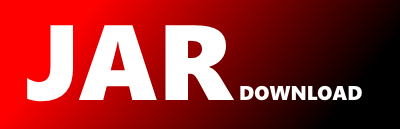
com.alphasystem.docx4j.builder.wml.LvlBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of open-xml-builder Show documentation
Show all versions of open-xml-builder Show documentation
Alpha system commons library
package com.alphasystem.docx4j.builder.wml;
import java.math.BigInteger;
import org.docx4j.wml.BooleanDefaultTrue;
import org.docx4j.wml.Jc;
import org.docx4j.wml.JcEnumeration;
import org.docx4j.wml.Lvl;
import org.docx4j.wml.NumFmt;
import org.docx4j.wml.NumberFormat;
import org.docx4j.wml.PPr;
import org.docx4j.wml.RPr;
/**
* Fluent API builder for org.docx4j.wml.Lvl
.
*
*/
public class LvlBuilder
extends com.alphasystem.docx4j.builder.OpenXmlBuilder
{
private LvlBuilder.StartBuilder startBuilder;
private LvlBuilder.LvlRestartBuilder lvlRestartBuilder;
private LvlBuilder.PStyleBuilder pStyleBuilder;
private LvlBuilder.SuffBuilder suffBuilder;
private LvlBuilder.LvlTextBuilder lvlTextBuilder;
private LvlBuilder.LvlPicBulletIdBuilder lvlPicBulletIdBuilder;
private LvlBuilder.LegacyBuilder legacyBuilder;
/**
* Initialize the underlying object.
*
*/
public LvlBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public LvlBuilder(Lvl object) {
super(object);
startBuilder = new LvlBuilder.StartBuilder(this.object.getStart());
lvlRestartBuilder = new LvlBuilder.LvlRestartBuilder(this.object.getLvlRestart());
pStyleBuilder = new LvlBuilder.PStyleBuilder(this.object.getPStyle());
suffBuilder = new LvlBuilder.SuffBuilder(this.object.getSuff());
lvlTextBuilder = new LvlBuilder.LvlTextBuilder(this.object.getLvlText());
lvlPicBulletIdBuilder = new LvlBuilder.LvlPicBulletIdBuilder(this.object.getLvlPicBulletId());
legacyBuilder = new LvlBuilder.LegacyBuilder(this.object.getLegacy());
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public LvlBuilder(Lvl src, Lvl target) {
this(target);
if (src!= null) {
Lvl.Start start = src.getStart();
if (start!= null) {
start = new LvlBuilder.StartBuilder(start, object.getStart()).getObject();
}
NumFmt numFmt = src.getNumFmt();
if (numFmt!= null) {
numFmt = new com.alphasystem.docx4j.builder.wml.NumFmtBuilder(numFmt, object.getNumFmt()).getObject();
}
Lvl.LvlRestart lvlRestart = src.getLvlRestart();
if (lvlRestart!= null) {
lvlRestart = new LvlBuilder.LvlRestartBuilder(lvlRestart, object.getLvlRestart()).getObject();
}
Lvl.PStyle pStyle = src.getPStyle();
if (pStyle!= null) {
pStyle = new LvlBuilder.PStyleBuilder(pStyle, object.getPStyle()).getObject();
}
Lvl.Suff suff = src.getSuff();
if (suff!= null) {
suff = new LvlBuilder.SuffBuilder(suff, object.getSuff()).getObject();
}
Lvl.LvlText lvlText = src.getLvlText();
if (lvlText!= null) {
lvlText = new LvlBuilder.LvlTextBuilder(lvlText, object.getLvlText()).getObject();
}
Lvl.LvlPicBulletId lvlPicBulletId = src.getLvlPicBulletId();
if (lvlPicBulletId!= null) {
lvlPicBulletId = new LvlBuilder.LvlPicBulletIdBuilder(lvlPicBulletId, object.getLvlPicBulletId()).getObject();
}
Lvl.Legacy legacy = src.getLegacy();
if (legacy!= null) {
legacy = new LvlBuilder.LegacyBuilder(legacy, object.getLegacy()).getObject();
}
Jc lvlJc = src.getLvlJc();
if (lvlJc!= null) {
lvlJc = new com.alphasystem.docx4j.builder.wml.JcBuilder(lvlJc, object.getLvlJc()).getObject();
}
PPr pPr = src.getPPr();
if (pPr!= null) {
pPr = new PPrBuilder(pPr, object.getPPr()).getObject();
}
RPr rPr = src.getRPr();
if (rPr!= null) {
rPr = new RPrBuilder(rPr, object.getRPr()).getObject();
}
withStart(start).withNumFmt(numFmt).withLvlRestart(lvlRestart).withPStyle(pStyle).withIsLgl(WmlBuilderFactory.cloneBooleanDefaultTrue(src.getIsLgl())).withSuff(suff).withLvlText(lvlText).withLvlPicBulletId(lvlPicBulletId).withLegacy(legacy).withLvlJc(lvlJc).withPPr(pPr).withRPr(rPr).withIlvl(WmlBuilderFactory.cloneBigInteger(src.getIlvl())).withTplc(src.getTplc()).withTentative(WmlBuilderFactory.cloneBoolean(src, "tentative"));
}
}
protected Lvl createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createLvl();
}
public LvlBuilder withStart(Lvl.Start value) {
if (value!= null) {
object.setStart(value);
}
return this;
}
public LvlBuilder withStart(Long val) {
boolean initialized = (val!= null);
if (initialized) {
withStart(startBuilder.withVal(val).getObject());
}
return this;
}
public LvlBuilder.StartBuilder getStartBuilder() {
return startBuilder;
}
public LvlBuilder withNumFmt(NumFmt value) {
if (value!= null) {
object.setNumFmt(value);
}
return this;
}
public LvlBuilder withNumFmt(NumberFormat val) {
if (val!= null) {
object.setNumFmt(new com.alphasystem.docx4j.builder.wml.NumFmtBuilder().withVal(val).getObject());
}
return this;
}
public LvlBuilder withLvlRestart(Lvl.LvlRestart value) {
if (value!= null) {
object.setLvlRestart(value);
}
return this;
}
public LvlBuilder withLvlRestart(Long val) {
boolean initialized = (val!= null);
if (initialized) {
withLvlRestart(lvlRestartBuilder.withVal(val).getObject());
}
return this;
}
public LvlBuilder.LvlRestartBuilder getLvlRestartBuilder() {
return lvlRestartBuilder;
}
public LvlBuilder withPStyle(Lvl.PStyle value) {
if (value!= null) {
object.setPStyle(value);
}
return this;
}
public LvlBuilder withPStyle(String val) {
boolean initialized = (val!= null);
if (initialized) {
withPStyle(pStyleBuilder.withVal(val).getObject());
}
return this;
}
public LvlBuilder.PStyleBuilder getPStyleBuilder() {
return pStyleBuilder;
}
public LvlBuilder withIsLgl(BooleanDefaultTrue value) {
if (value!= null) {
object.setIsLgl(value);
}
return this;
}
public LvlBuilder withIsLgl(Boolean val) {
if (val!= null) {
object.setIsLgl(new BooleanDefaultTrueBuilder().withVal(val).getObject());
}
return this;
}
public LvlBuilder withSuff(Lvl.Suff value) {
if (value!= null) {
object.setSuff(value);
}
return this;
}
public LvlBuilder withSuff(String val) {
boolean initialized = (val!= null);
if (initialized) {
withSuff(suffBuilder.withVal(val).getObject());
}
return this;
}
public LvlBuilder.SuffBuilder getSuffBuilder() {
return suffBuilder;
}
public LvlBuilder withLvlText(Lvl.LvlText value) {
if (value!= null) {
object.setLvlText(value);
}
return this;
}
public LvlBuilder withLvlText(String val, Boolean _null) {
boolean initialized = ((val!= null)||(_null!= null));
if (initialized) {
withLvlText(lvlTextBuilder.withVal(val).withNull(_null).getObject());
}
return this;
}
public LvlBuilder.LvlTextBuilder getLvlTextBuilder() {
return lvlTextBuilder;
}
public LvlBuilder withLvlPicBulletId(Lvl.LvlPicBulletId value) {
if (value!= null) {
object.setLvlPicBulletId(value);
}
return this;
}
public LvlBuilder withLvlPicBulletId(Long val) {
boolean initialized = (val!= null);
if (initialized) {
withLvlPicBulletId(lvlPicBulletIdBuilder.withVal(val).getObject());
}
return this;
}
public LvlBuilder.LvlPicBulletIdBuilder getLvlPicBulletIdBuilder() {
return lvlPicBulletIdBuilder;
}
public LvlBuilder withLegacy(Lvl.Legacy value) {
if (value!= null) {
object.setLegacy(value);
}
return this;
}
public LvlBuilder withLegacy(Boolean legacy, Long legacySpace, Long legacyIndent) {
boolean initialized = (((legacy!= null)||(legacySpace!= null))||(legacyIndent!= null));
if (initialized) {
withLegacy(legacyBuilder.withLegacy(legacy).withLegacySpace(legacySpace).withLegacyIndent(legacyIndent).getObject());
}
return this;
}
public LvlBuilder.LegacyBuilder getLegacyBuilder() {
return legacyBuilder;
}
public LvlBuilder withLvlJc(Jc value) {
if (value!= null) {
object.setLvlJc(value);
}
return this;
}
public LvlBuilder withLvlJc(JcEnumeration val) {
if (val!= null) {
object.setLvlJc(new com.alphasystem.docx4j.builder.wml.JcBuilder().withVal(val).getObject());
}
return this;
}
public LvlBuilder withPPr(PPr value) {
if (value!= null) {
object.setPPr(value);
}
return this;
}
public LvlBuilder withRPr(RPr value) {
if (value!= null) {
object.setRPr(value);
}
return this;
}
public LvlBuilder withIlvl(BigInteger value) {
if (value!= null) {
object.setIlvl(value);
}
return this;
}
/**
* Calls setIlvl
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public LvlBuilder withIlvl(String value) {
if (value!= null) {
object.setIlvl(new BigInteger(value));
}
return this;
}
/**
* Calls setIlvl
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public LvlBuilder withIlvl(Long value) {
if (value!= null) {
object.setIlvl(BigInteger.valueOf(value));
}
return this;
}
public LvlBuilder withTplc(String value) {
if (value!= null) {
object.setTplc(value);
}
return this;
}
public LvlBuilder withTentative(Boolean value) {
if (value!= null) {
object.setTentative(value);
}
return this;
}
/**
* Fluent API builder for org.docx4j.wml.Lvl$Legacy
.
*
*/
public static class LegacyBuilder
extends com.alphasystem.docx4j.builder.OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public LegacyBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public LegacyBuilder(Lvl.Legacy object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public LegacyBuilder(Lvl.Legacy src, Lvl.Legacy target) {
this(target);
if (src!= null) {
withLegacy(WmlBuilderFactory.cloneBoolean(src, "legacy")).withLegacySpace(WmlBuilderFactory.cloneBigInteger(src.getLegacySpace())).withLegacyIndent(WmlBuilderFactory.cloneBigInteger(src.getLegacyIndent()));
}
}
protected Lvl.Legacy createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createLvlLegacy();
}
public LvlBuilder.LegacyBuilder withLegacy(Boolean value) {
if (value!= null) {
object.setLegacy(value);
}
return this;
}
public LvlBuilder.LegacyBuilder withLegacySpace(BigInteger value) {
if (value!= null) {
object.setLegacySpace(value);
}
return this;
}
/**
* Calls setLegacySpace
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public LvlBuilder.LegacyBuilder withLegacySpace(String value) {
if (value!= null) {
object.setLegacySpace(new BigInteger(value));
}
return this;
}
/**
* Calls setLegacySpace
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public LvlBuilder.LegacyBuilder withLegacySpace(Long value) {
if (value!= null) {
object.setLegacySpace(BigInteger.valueOf(value));
}
return this;
}
public LvlBuilder.LegacyBuilder withLegacyIndent(BigInteger value) {
if (value!= null) {
object.setLegacyIndent(value);
}
return this;
}
/**
* Calls setLegacyIndent
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public LvlBuilder.LegacyBuilder withLegacyIndent(String value) {
if (value!= null) {
object.setLegacyIndent(new BigInteger(value));
}
return this;
}
/**
* Calls setLegacyIndent
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public LvlBuilder.LegacyBuilder withLegacyIndent(Long value) {
if (value!= null) {
object.setLegacyIndent(BigInteger.valueOf(value));
}
return this;
}
}
/**
* Fluent API builder for org.docx4j.wml.Lvl$LvlPicBulletId
.
*
*/
public static class LvlPicBulletIdBuilder
extends com.alphasystem.docx4j.builder.OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public LvlPicBulletIdBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public LvlPicBulletIdBuilder(Lvl.LvlPicBulletId object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public LvlPicBulletIdBuilder(Lvl.LvlPicBulletId src, Lvl.LvlPicBulletId target) {
this(target);
if (src!= null) {
withVal(WmlBuilderFactory.cloneBigInteger(src.getVal()));
}
}
protected Lvl.LvlPicBulletId createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createLvlLvlPicBulletId();
}
public LvlBuilder.LvlPicBulletIdBuilder withVal(BigInteger value) {
if (value!= null) {
object.setVal(value);
}
return this;
}
/**
* Calls setVal
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public LvlBuilder.LvlPicBulletIdBuilder withVal(String value) {
if (value!= null) {
object.setVal(new BigInteger(value));
}
return this;
}
/**
* Calls setVal
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public LvlBuilder.LvlPicBulletIdBuilder withVal(Long value) {
if (value!= null) {
object.setVal(BigInteger.valueOf(value));
}
return this;
}
}
/**
* Fluent API builder for org.docx4j.wml.Lvl$LvlRestart
.
*
*/
public static class LvlRestartBuilder
extends com.alphasystem.docx4j.builder.OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public LvlRestartBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public LvlRestartBuilder(Lvl.LvlRestart object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public LvlRestartBuilder(Lvl.LvlRestart src, Lvl.LvlRestart target) {
this(target);
if (src!= null) {
withVal(WmlBuilderFactory.cloneBigInteger(src.getVal()));
}
}
protected Lvl.LvlRestart createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createLvlLvlRestart();
}
public LvlBuilder.LvlRestartBuilder withVal(BigInteger value) {
if (value!= null) {
object.setVal(value);
}
return this;
}
/**
* Calls setVal
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public LvlBuilder.LvlRestartBuilder withVal(String value) {
if (value!= null) {
object.setVal(new BigInteger(value));
}
return this;
}
/**
* Calls setVal
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public LvlBuilder.LvlRestartBuilder withVal(Long value) {
if (value!= null) {
object.setVal(BigInteger.valueOf(value));
}
return this;
}
}
/**
* Fluent API builder for org.docx4j.wml.Lvl$LvlText
.
*
*/
public static class LvlTextBuilder
extends com.alphasystem.docx4j.builder.OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public LvlTextBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public LvlTextBuilder(Lvl.LvlText object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public LvlTextBuilder(Lvl.LvlText src, Lvl.LvlText target) {
this(target);
if (src!= null) {
withVal(src.getVal()).withNull(WmlBuilderFactory.cloneBoolean(src, "_null"));
}
}
protected Lvl.LvlText createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createLvlLvlText();
}
public LvlBuilder.LvlTextBuilder withVal(String value) {
if (value!= null) {
object.setVal(value);
}
return this;
}
public LvlBuilder.LvlTextBuilder withNull(Boolean value) {
if (value!= null) {
object.setNull(value);
}
return this;
}
}
/**
* Fluent API builder for org.docx4j.wml.Lvl$PStyle
.
*
*/
public static class PStyleBuilder
extends com.alphasystem.docx4j.builder.OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public PStyleBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public PStyleBuilder(Lvl.PStyle object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public PStyleBuilder(Lvl.PStyle src, Lvl.PStyle target) {
this(target);
if (src!= null) {
withVal(src.getVal());
}
}
protected Lvl.PStyle createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createLvlPStyle();
}
public LvlBuilder.PStyleBuilder withVal(String value) {
if (value!= null) {
object.setVal(value);
}
return this;
}
}
/**
* Fluent API builder for org.docx4j.wml.Lvl$Start
.
*
*/
public static class StartBuilder
extends com.alphasystem.docx4j.builder.OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public StartBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public StartBuilder(Lvl.Start object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public StartBuilder(Lvl.Start src, Lvl.Start target) {
this(target);
if (src!= null) {
withVal(WmlBuilderFactory.cloneBigInteger(src.getVal()));
}
}
protected Lvl.Start createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createLvlStart();
}
public LvlBuilder.StartBuilder withVal(BigInteger value) {
if (value!= null) {
object.setVal(value);
}
return this;
}
/**
* Calls setVal
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public LvlBuilder.StartBuilder withVal(String value) {
if (value!= null) {
object.setVal(new BigInteger(value));
}
return this;
}
/**
* Calls setVal
method.
*
* @param value
* Value to set
* @return
* reference to this
*/
public LvlBuilder.StartBuilder withVal(Long value) {
if (value!= null) {
object.setVal(BigInteger.valueOf(value));
}
return this;
}
}
/**
* Fluent API builder for org.docx4j.wml.Lvl$Suff
.
*
*/
public static class SuffBuilder
extends com.alphasystem.docx4j.builder.OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public SuffBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public SuffBuilder(Lvl.Suff object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public SuffBuilder(Lvl.Suff src, Lvl.Suff target) {
this(target);
if (src!= null) {
withVal(src.getVal());
}
}
protected Lvl.Suff createObject() {
return WmlBuilderFactory.OBJECT_FACTORY.createLvlSuff();
}
public LvlBuilder.SuffBuilder withVal(String value) {
if (value!= null) {
object.setVal(value);
}
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy