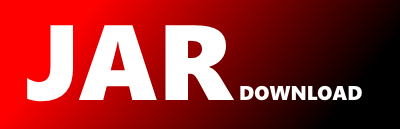
com.alphasystem.docx4j.builder.wml.PBuilder Maven / Gradle / Ivy
package com.alphasystem.docx4j.builder.wml;
import java.util.List;
import com.alphasystem.docx4j.builder.OpenXmlBuilder;
import org.docx4j.wml.P;
import org.docx4j.wml.PPr;
/**
* Fluent API builder for org.docx4j.wml.P
.
*
*/
public class PBuilder
extends OpenXmlBuilder
{
/**
* Initialize the underlying object.
*
*/
public PBuilder() {
this(null);
}
/**
* Initialize the builder with given object.
*
* @param object
* the given object
*/
public PBuilder(P object) {
super(object);
}
/**
* Copies values fom src
into target
. Values of target
will be overridden by the values from src
.
*
* @param src
* source object
* @param target
* target object
*/
public PBuilder(P src, P target) {
this(target);
if (src!= null) {
PPr pPr = src.getPPr();
if (pPr!= null) {
pPr = new PPrBuilder(pPr, object.getPPr()).getObject();
}
List